How to use JWT token in Java Spring Boot?
How to use JWT token in Java Spring Boot?
I'm happy to help!
In a Java Spring Boot application, you can use the JSON Web Token (JWT) to authenticate and authorize requests. Here's a step-by-step guide on how to use JWT tokens:
Step 1: Choose a Library
You'll need a library to handle JWT generation, verification, and token parsing. Popular options include:
jwt-simple
(SimpleJSON) jjwt
(Java JSON Web Token)
For this example, we'll use jjwt
.
Step 2: Configure Dependencies
In your pom.xml
file (if you're using Maven) or build.gradle
file (if you're using Gradle), add the required dependencies:
com.auth0
jjwt
0.9.1
dependencies {
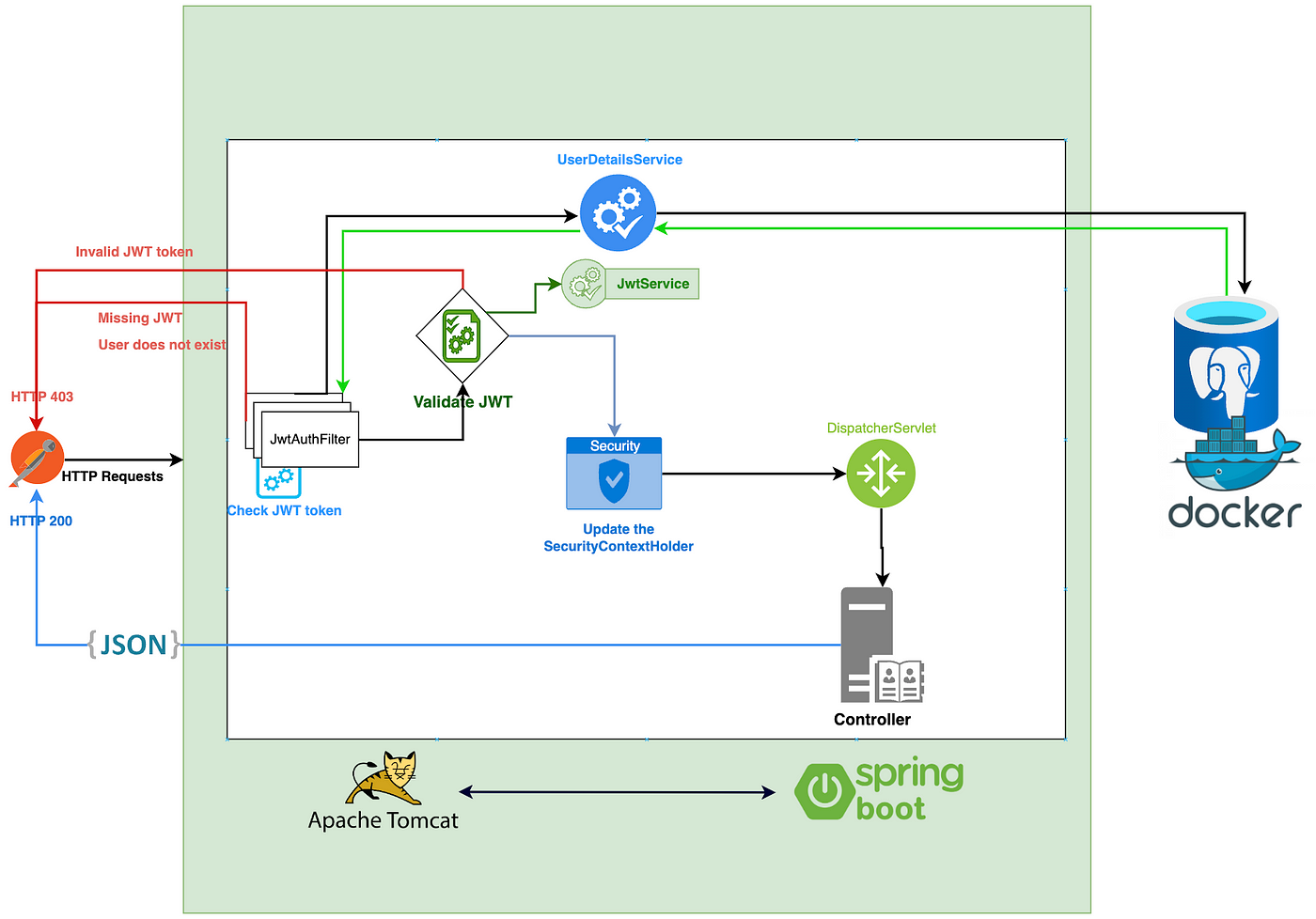
implementation 'com.auth0:jjwt:0.9.1'
}
Step 3: Create a JWT Token
To generate a JWT token, you'll need to create a TokenService
class:
@Service
public class TokenService {
private static final String SECRET_KEY = "your-secret-key";
private static final String ISSUER = "your-issuer";
public String generateToken(User user) {
// Create a claims object with the user's information
Claims claims = Jwts.claims().setSubject(user.getUsername())
.setExpiration(Date.from(Instant.now().plus(Duration.ofDays(7))));
// Sign the token with the secret key
String token = Jwts.builder().setClaims(claims).setIssuedAt(new Date()).setExpiration(new Date())
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
return token;
}
}
In this example, we're creating a User
object and extracting the username. We're then generating a JWT token with the user's information, expiration date (7 days), and signing it with the secret key using HMAC SHA-256.
Step 4: Verify the JWT Token
To verify the JWT token, create another service class:
@Service
public class TokenVerificationService {
public boolean verifyToken(String token) {
try {
Jws claim = Jwts.parser().setSigningKey(SECRET_KEY).parseClaimsJws(token);
return true;
} catch (Exception e) {
return false;
}
}
}
This service attempts to parse and verify the JWT token using the same secret key. If the token is valid, it returns true
; otherwise, it returns false
.
Step 5: Integrate with Spring Security
To integrate your JWT token generation and verification with Spring Security, you'll need to create a custom Authentication Provider:
@Configuration
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private TokenService tokenService;
@Autowired
private TokenVerificationService tokenVerificationService;
@Override
protected void configure(HttpSecurity http) throws Exception {
// Custom authentication provider for JWT tokens
http.oauth2Login()
.and()
.oauth2AuthorizedGrantTypes("jwt")
.oauth2AuthenticationProvider(new JwtAuthenticationProvider(tokenService, tokenVerificationService));
}
}
In this example, we're creating a custom JwtAuthenticationProvider
that uses our TokenService
and TokenVerificationService
to authenticate and authorize requests.
Step 6: Use JWT Tokens in Your Controllers
Finally, you can use the JWT tokens in your controllers to secure your endpoints:
@RestController
public class MyController {
@Autowired
private TokenVerificationService tokenVerificationService;
@GetMapping("/protected-endpoint")
public String protectedEndpoint(@RequestHeader("Authorization") String token) {
if (!tokenVerificationService.verifyToken(token)) {
return "Unauthorized";
}
// Authorized, proceed with your logic
}
}
In this example, we're using the @RequestHeader
annotation to retrieve the JWT token from the Authorization header. We then verify the token using our custom TokenVerificationService
. If the token is valid, we authorize the request and proceed with our logic.
That's it! You've successfully implemented JWT tokens in your Java Spring Boot application.
Remember to handle errors properly, log exceptions, and consider implementing rate limiting or IP blocking to prevent abuse.
Java jwt github
I'm happy to help you with your query!
What is JWT?
JSON Web Token (JWT) is a compact, URL-safe means of representing claims to be transferred between two parties. The token is digitally signed and contains the following:
Header: Contains the type of the token and the signature algorithm. Payload (Claims): Includes information about the user such as username, email address, role, and other custom claims. Signature: The digital signature generated using a secret key and the header and payload.How does JWT work?
Here's an overview of how JWT works:
Request: A user requests access to a protected resource (e.g., login or API endpoint). Server-side generation: On the server side, a JWT is generated with the necessary claims (e.g., username, role) and signed using a secret key. Token transmission: The JWT is sent back to the client as a cookie or HTTP header. Client-side verification: The client verifies the signature of the received token by re-generating it using the same algorithm and secret key. If the signature matches, the client trusts the token.Why use JWT in GitHub?
GitHub uses JWT to authenticate users and provide secure access to repositories, issues, and other features. Here are some reasons why:
Secure authentication: JWT ensures that only authorized users can access sensitive data. Stateless: Since JWT is a token-based solution, it eliminates the need for session management on the server side. Flexible claims: You can include custom claims to convey additional information about the user or their role.GitHub's use of JWT
In GitHub, JWT tokens are used to authenticate users and provide access to:
APIs: The GitHub API uses JWT tokens to verify user identity and authorize requests. Authentication: During login, GitHub generates a JWT token that can be used to authenticate subsequent requests. Repository management: JWT is used to manage repository permissions, allowing users to access specific resources.In conclusion, JSON Web Tokens (JWT) are a powerful authentication mechanism used by GitHub to provide secure and stateless authentication for its users. By leveraging the claims in the token, GitHub can efficiently manage user identity and access control, ensuring a seamless experience across its platforms.