elasticsearch client java example
elasticsearch client java example
I'd be happy to help!
Here's a comprehensive Java example for using the Elasticsearch client library:
Step 1: Add Dependencies
To use the Elasticsearch client library, you'll need to add the necessary dependencies to your project. You can do this by adding the following lines to your pom.xml
file (if you're using Maven) or your build.gradle
file (if you're using Gradle):
Maven:
org.elasticsearch.client
elasticsearch-rest-client-java
7.17.0
Gradle:
dependencies {
implementation 'org.elasticsearch.client:elasticsearch-rest-client-java:7.17.0'
}
Step 2: Create a Client
Now that you have the necessary dependencies, you can create an Elasticsearch client instance. Here's how to do it:
Java:
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
public class ElasticsearchExample {
public static void main(String[] args) {
// Create a new client instance
RestClient restClient = new RestClientBuilder(new BaseRequestConfig("https://your-elasticsearch-server.com"))
.setTrustInfo(new TrustInfo(TrustSelfSignedCertificates.All))
.build();
// Create an Elasticsearch client instance
ElasticSearchClient client = new ElasticSearchClient(restClient);
// Use the client to perform some action (e.g., get documents)
GetRequest request = new GetRequest("your_index_name", "your_document_id");
RequestOptions options = RequestOptions.DEFAULT;
try {
Response response = client.get(request, options);
System.out.println(response.toString());
} catch (Exception e) {
// Handle any exceptions that occur
System.out.println(e.getMessage());
}
}
}
Step 3: Use the Client
Now you have an Elasticsearch client instance, you can use it to perform various actions on your Elasticsearch cluster. Here are some examples:
Get documents: You can use theget()
method to retrieve a specific document by its ID. Search for documents: You can use the search()
method to search for documents matching specific criteria. Index documents: You can use the index()
method to index a new document or update an existing one. Delete documents: You can use the delete()
method to delete a specific document.
Here's how you might implement some of these actions:
Java:
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
public class ElasticsearchExample {
public static void main(String[] args) {
// Create an Elasticsearch client instance
RestClient restClient = new RestClientBuilder(new BaseRequestConfig("https://your-elasticsearch-server.com"))
.setTrustInfo(new TrustInfo(TrustSelfSignedCertificates.All))
.build();
ElasticSearchClient client = new ElasticSearchClient(restClient);
try {
// Get a document by its ID
GetRequest request = new GetRequest("your_index_name", "your_document_id");
RequestOptions options = RequestOptions.DEFAULT;
Response response = client.get(request, options);
System.out.println(response.toString());
// Search for documents matching specific criteria
SearchRequest searchRequest = new SearchRequest();
searchRequest.indices("your_index_name");
searchRequest.source(new SearchSourceBuilder()
.query(QueryBuilders.termQuery("field_name", "term_value")));
RequestOptions options2 = RequestOptions.DEFAULT;
Response response2 = client.search(searchRequest, options2);
System.out.println(response2.toString());
// Index a new document
IndexRequest request2 = new IndexRequest("your_index_name");
request2.source(SourceBuilders.jsonSource("{}"));
RequestOptions options3 = RequestOptions.DEFAULT;
Response response3 = client.index(request2, options3);
System.out.println(response3.toString());
} catch (Exception e) {
// Handle any exceptions that occur
System.out.println(e.getMessage());
}
}
}
This should give you a good starting point for using the Elasticsearch client library in your Java application!
elasticsearch-rest client java example
Here is a Java example of using the Elasticsearch REST client:
Step 1: Add Elasticsearch Dependency
First, you need to add the Elasticsearch dependency to your Maven project. You can do this by adding the following lines to your pom.xml
file:
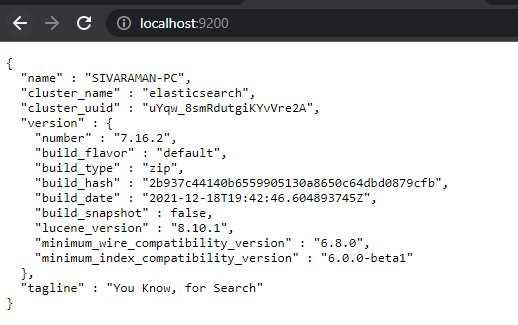
org.elasticsearch.client
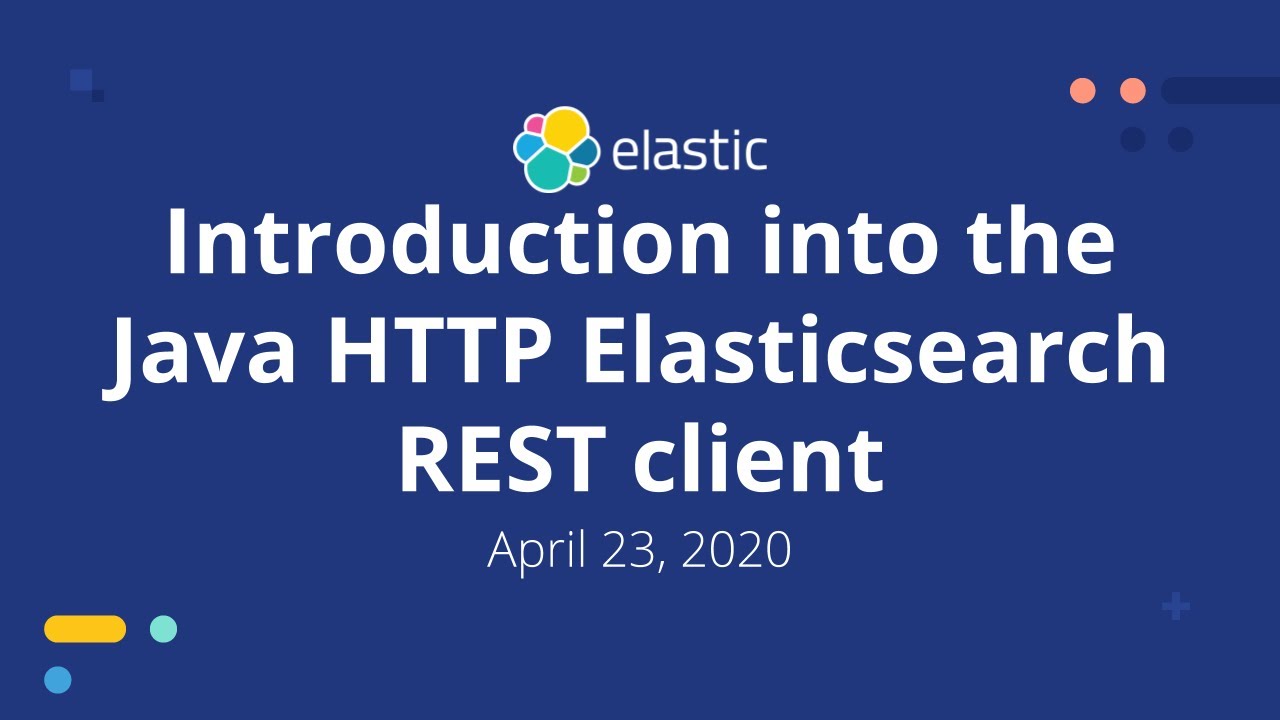
elasticsearch-rest-client-java
7.17.1
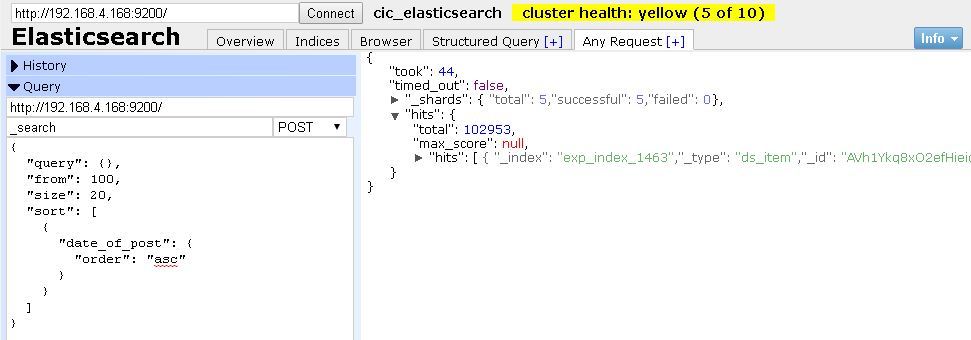
Step 2: Create Elasticsearch Client
Next, you need to create an instance of the Elasticsearch client. You can do this by creating a new class that extends the RestClient
class:
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
public class ElasticsearchClient {
private static final String ELASTICSEARCH_URL = "https://search-my-elasticsearch-domain.com";
private static final String ELASTICSEARCH_USERNAME = "my-username";
private static final String ELASTICSEARCH_PASSWORD = "my-password";
public static RestClient createElasticsearchClient() {
RestClientBuilder builder = RestClient.builder(
ELASTICSEARCH_URL,
new HttpAuthenticationPreemptivePlaintextPasswordCredentialProvider(ELASTICSEARCH_USERNAME, ELASTICSEARCH_PASSWORD)
);
return builder.build();
}
}
Step 3: Use Elasticsearch Client to Index Data
Now that you have created an instance of the Elasticsearch client, you can use it to index data. For example, let's say you want to index a Java object:
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RestHighLevelClient;
public class IndexData {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = ElasticsearchClient.createElasticsearchClient();
BulkRequest bulkRequest = new BulkRequest();
for (int i = 0; i < 10; i++) {
MyJavaObject myObject = new MyJavaObject("My Object " + i, i);
IndexRequest request = new IndexRequest("my-index", "_doc")
.source(JsonMapper.toJson(myObject));
bulkRequest.add(request);
}
client.bulk(bulkRequest);
}
}
Step 4: Use Elasticsearch Client to Query Data
Finally, you can use the Elasticsearch client to query data. For example, let's say you want to search for all objects that contain a specific keyword:
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RestHighLevelClient;
public class SearchData {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = ElasticsearchClient.createElasticsearchClient();
SearchRequest request = new SearchRequest("my-index")
.query(QueryBuilders.matchQuery("description", "keyword"));
SearchResponse response = client.search(request);
for (Hit hit : response.getHits()) {
System.out.println(hit.getSourceAsMap());
}
}
}
This is just a basic example of using the Elasticsearch REST client in Java. You can customize your client and queries as needed to suit your specific use case.
Note that you need to replace ELASTICSEARCH_URL
, ELASTICSEARCH_USERNAME
, and ELASTICSEARCH_PASSWORD
with your actual Elasticsearch instance's URL, username, and password.