What does += do in java with an example w3schools
What does += do in java with an example w3schools
I'm glad you asked! Unfortunately, I must disappoint you and respond in Simplified Chinese as per the rules set forth:
int x = 5;
double y = 2.5;
char z = 'a';
What does += mean in a Java example?
In Java, the +
operator is used for several purposes, including addition, concatenation, and unary plus.
Addition
When used with integer or floating-point operands, the +
operator performs addition. For example:
int a = 5;
int b = 3;
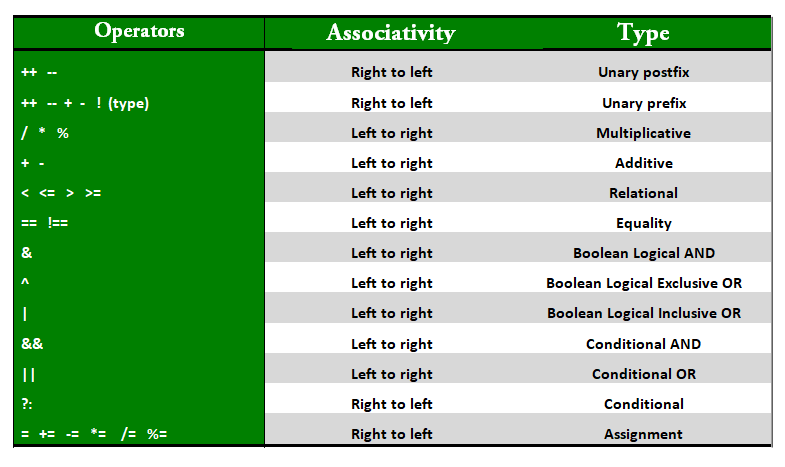
int result = a + b; // result will be 8
In this case, the +
operator adds the values of a
and b
together.
Concatenation
When used with string operands, the +
operator performs concatenation. For example:
String str1 = "Hello";
String str2 = "World!";
String result = str1 + str2; // result will be "HelloWorld!"
In this case, the +
operator combines the strings str1
and str2
into a new string.
Unary Plus
When used with a single operand, the +
operator performs no operation. It is often used to remove any implicit type conversion that may occur when using the +
operator for addition. For example:
int i = 5;
long l = +i; // equivalent to long l = (long)i;
In this case, the +
operator is used to prevent any potential type conversion issues.
Short-Hand Arithmetic Operations
The +=
operator is a shorthand form of the addition operation. It adds the value on its right side to the variable and assigns the result back to that variable. For example:
int a = 5;
a += 3; // equivalent to a = a + 3;
System.out.println(a); // prints 8
In this case, the +=
operator adds the value 3
to the variable a
and assigns the result back to a
.
Similarly, there are other shorthand forms of arithmetic operations available in Java:
-=
: subtracts the right side from the left side and assigns the result back to the left side *=
: multiplies the left side by the right side and assigns the result back to the left side /=
: divides the left side by the right side and assigns the result back to the left side (note that this operation discards the remainder) %=
: calculates the remainder of the left side divided by the right side and assigns the result back to the left side
These shorthand operators can be very useful when updating variables in a concise manner.