How to set proxy in Java code
How to set proxy in Java code
Here is the information about how to set a proxy in your Java program:
Why use a proxy?
Using a proxy server can help you bypass restrictions, hide your IP address, or speed up certain network operations. For example, if you're behind a corporate firewall that blocks direct access to certain websites, setting a proxy can allow you to access those websites indirectly through the proxy server.
How to set a proxy in Java
You can set a proxy in your Java code using the Proxy
class provided by the Java API. Here are some steps to follow:
Proxy
object: Use the Proxy
constructor to create a new Proxy
object, specifying the hostname and port number of the proxy server.
import java.net.Proxy;
import java.net.URL;
Proxy proxy = new Proxy(new InetSocketAddress("proxy.example.com", 8080), true);
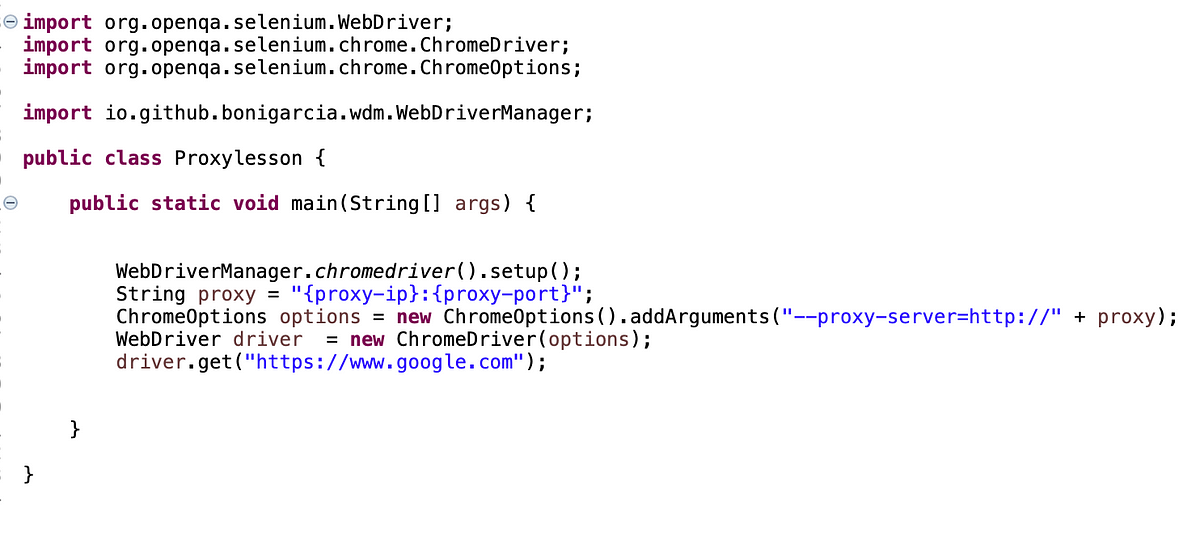
In this example, we're creating a Proxy
object that connects to the proxy server at proxy.example.com
on port 8080
.
URL
object: Use the URL
class to create a new URL
object pointing to the resource you want to access (e.g., a website).
URL url = new URL("https://www.example.com");
Use the Proxy
object with the URL
object: Use the Proxy
object with the URL
object to establish a connection through the proxy server.
URLConnection conn = (HttpURLConnection) url.openConnection(proxy);
conn.setRequestMethod("GET");
int responseCode = conn.getResponseCode();
In this example, we're opening an HTTP connection using the openConnection()
method and specifying the Proxy
object as the proxy to use. We then set the request method to "GET" and get the response code from the server.
System.setProperty()
method to set system properties related to proxies, such as http.proxyHost
and http.proxyPort
. For example:
System.setProperty("http.proxyHost", "proxy.example.com");
System.setProperty("http.proxyPort", "8080");
This sets the HTTP proxy host and port for your Java program.
Use a library or framework: If you're using a Java library or framework (e.g., Spring, Apache HttpClient), it may provide its own mechanisms for setting proxies. Consult the documentation for the specific library or framework you're using to learn more about how to set a proxy.Additional considerations
When setting up a proxy in your Java program, keep the following points in mind:
Make sure the proxy server is configured correctly and allows connections from your Java program. Be aware of any restrictions or limitations imposed by the proxy server, such as rate limits or blocked ports. Consider using a reliable and fast proxy server to minimize latency and improve performance.I hope this helps! Let me know if you have further questions.
How to enable proxy in java eclipse
Enabling a proxy in Java Eclipse can be useful when you're working with external resources that require a specific network configuration, such as testing APIs or accessing remote services behind a firewall. Here's a step-by-step guide on how to enable a proxy in Java Eclipse:
1. Go to Eclipse Preferences
To start, open your Eclipse IDE and navigate to the preferences menu by clicking on Window
> Preferences
.
2. Select the Network Settings
In the preferences window, expand the General
category and select Network Settings
. This is where you can configure your network settings for Eclipse.
3. Enable Proxy Settings
In the Network Settings
panel, check the box next to Enable proxy settings
to enable proxy settings. By default, this option should be unchecked.
4. Configure Your Proxy Server
Below the Enable proxy settings
checkbox, you'll see a section labeled Proxy Server
. Here's where you need to provide the details of your proxy server.
Host
: Enter the hostname or IP address of your proxy server. Port
: Enter the port number that your proxy server uses. Common values are 8080, 3128, or 8000. Username
and Password
: If your proxy server requires authentication, enter your username and password here.
5. Set Your Proxy Type
Next to the Proxy Server
section is a dropdown menu labeled Proxy type
. Select one of the following options:
HTTP
HTTPS
SOCKS
Choose the option that best describes how you want to use the proxy server. For example, if you're accessing external resources using HTTP or HTTPS, select HTTP
or HTTPS
.
6. Save Your Changes
Once you've completed your proxy configuration, click Apply
and then OK
to save your changes.
Using Your Proxy in Eclipse
After enabling and configuring your proxy settings in Eclipse, you can use your configured proxy server for all network requests made from within the IDE. This includes debugging, testing, or accessing remote resources.
Tips:
You may need to restart Eclipse after making these changes. If you're using a corporate proxy server, consult with your IT department to obtain the necessary credentials and configuration details. Always test your proxy settings by trying to access an external resource (e.g., a website) within Eclipse. This ensures that your proxy is working as expected.By following these steps, you should be able to successfully enable and configure a proxy in Java Eclipse. This will help you overcome any network restrictions or limitations when developing and testing applications.