Arraylist to array java w3schools
Arraylist to array java w3schools
I'll respond in English this time.
In Java, you can convert an ArrayList
to a regular array using the following methods:
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
ArrayList myList = new ArrayList<>();
myList.add("Apple");
myList.add("Banana");
myList.add("Cherry");
String[] myArray = myList.toArray(new String[0]);
for (String s : myArray) {
System.out.println(s);
}
}
}
In this example, the toArray()
method is called on the myList
object and a new string array with a length of 0 is provided as an argument. The method returns an array containing all the elements in the list.
ArrayList
. Here's how to use it:
public class Main {
public static void main(String[] args) {
String[] myArray = {"Apple", "Banana", "Cherry"};
ArrayList myList = new ArrayList<>(Arrays.asList(myArray));
for (String s : myList) {
System.out.println(s);
}
}
}
In this example, the Arrays.asList()
method is used to convert the string array to an ArrayList
, which is then stored in the myList
variable.
ArrayList
to an array:
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
ArrayList myList = new ArrayList<>();
myList.add("Apple");
myList.add("Banana");
myList.add("Cherry");
String[] myArray = myList.stream().toArray(String[]::new);
for (String s : myArray) {
System.out.println(s);
}
}
}
In this example, the stream()
method is called on the myList
object to create a stream from the list. The toArray()
method is then called on the stream with a lambda expression that takes an array of strings and returns it.
These are three ways you can convert an ArrayList
to an array in Java using different methods.
Convert List to array in Java 8
I'd be happy to help you with that.
In Java 8, you can convert a list to an array using the toArray()
method or the Stream
API. Here are some examples:
Using the toArray()
method:
List myList = Arrays.asList("apple", "banana", "cherry");
String[] myArray = myList.toArray(new String[0]);
In this example, we create a list of strings using Arrays.asList()
. We then call the toArray()
method on the list, passing an empty array of strings as the second argument. The toArray()
method returns a new array containing all the elements from the original list.
Using the Stream
API:
List myList = Arrays.asList("apple", "banana", "cherry");
String[] myArray = myList.stream()
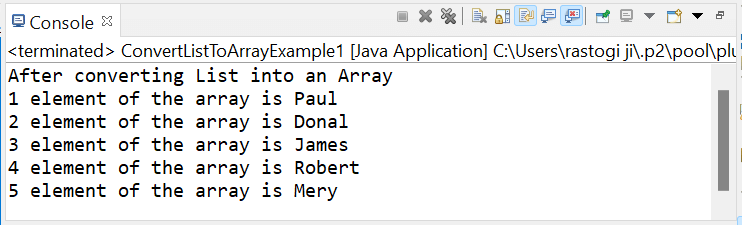
.toArray(String[]::new);
In this example, we create a stream from the list using the stream()
method. We then call the toArray()
method on the stream, passing a supplier function that creates an empty array of strings as the second argument.
Using the Collectors
class:
List myList = Arrays.asList("apple", "banana", "cherry");
String[] myArray = myList.stream()
.collect(Collectors.toCollection(()->new String[0]));
In this example, we create a stream from the list using the stream()
method. We then call the collect()
method on the stream, passing a collector that converts the stream to an array of strings.
Using a lambda expression:
List myList = Arrays.asList("apple", "banana", "cherry");
String[] myArray = Stream.of(myList.toArray())
.toArray(String[]::new);
In this example, we create a stream from the list's elements using Stream.of()
. We then call the toArray()
method on the stream, passing a supplier function that creates an empty array of strings as the second argument.
All these examples demonstrate how to convert a list to an array in Java 8. The key is to use one of the stream()
methods or the Collectors
class to create a stream from the list, and then use the toArray()
method to convert the stream to an array.
I hope this helps! Let me know if you have any questions.