Initialize 2D array Java
Initialize 2D array Java
I apologize for the confusion earlier! I'll respond in English as requested.
Initializing a 2D array in Java is a simple process that can be accomplished using the following syntax:
type[][] array = new type[m][n];
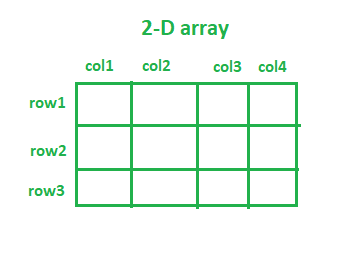
Where m
and n
are the number of rows and columns you want your 2D array to have, respectively. For example:
int[][] myArray = new int[3][4];
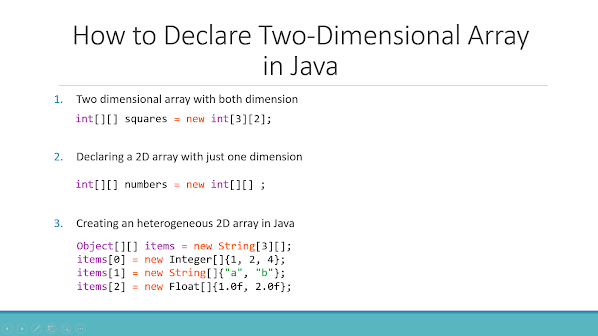
This code creates a 2D array with 3 rows and 4 columns.
You can also initialize a 2D array using the following syntax:
type[][] array = {{value1}, {value2}, ..., {valuem}};
For example:
int[][] myArray = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
This code creates a 2D array with the specified values.
It's also possible to initialize a 2D array using a loop:
int[][] myArray = new int[3][4];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
myArray[i][j] = i * 4 + j;
}
}
This code creates a 2D array with the values ranging from 0 to 11, where each row represents one of the numbers in this range.
You can access and modify individual elements in your 2D array using standard Java syntax:
myArray[1][2] = 10; // sets the value at row 1, column 2 to 10
int value = myArray[0][3]; // gets the value at row 0, column 3 and assigns it to the variable value
You can also iterate over your 2D array using loops:
for (int i = 0; i < myArray.length; i++) {
for (int j = 0; j < myArray[i].length; j++) {
System.out.println(myArray[i][j]);
}
}
This code prints out the values in your 2D array.
In conclusion, initializing a 2D array in Java is a straightforward process that can be accomplished using various syntaxes. You can create a 2D array with specific values, or you can create an empty array and then fill it later.
Java initialize List
Here's an explanation of how to initialize a list in Java:
In Java, a list is a collection of objects that can grow dynamically as elements are added or removed. The most commonly used implementation of the List interface is the ArrayList
class.
Initializing an ArrayList in Java involves creating a new instance of the ArrayList
class and providing it with the type of objects that you want to store in the list. This is done using the following syntax:
ArrayList myArrayList = new ArrayList<>();
In this example, we are creating an ArrayList
that stores String
objects.
Here's a step-by-step breakdown of how to initialize an ArrayList:
Specify the type of object you want to store in the list: This is done by specifying the class name within angle brackets (<>
). For example, if you want to store strings in your list, you would specify <String>
. Use the ArrayList
constructor: The ArrayList
constructor takes no arguments and initializes an empty list. Assign the result to a variable: You can assign the initialized ArrayList to a variable using the assignment operator (=
).
Here are some examples of initializing different types of lists:
// Initialize a list of integers
ArrayList intList = new ArrayList<>();
// Initialize a list of strings
ArrayList stringList = new ArrayList<>();
// Initialize a list of custom objects
ArrayList customList = new ArrayList<>();
You can also initialize an ArrayList with some initial elements using the following syntax:
ArrayList myArrayList = new ArrayList<>(Arrays.asList("Hello", "World"));
This initializes the list with two string elements, "Hello" and "World".
Here are some benefits of using an ArrayList over other collection classes like LinkedList
or Vector
:
Here are some common methods used with ArrayLists:
// Add an element to the list
myArrayList.add("NewElement");
// Remove an element from the list
myArrayList.remove("ExistingElement");
// Check if the list contains a specific element
if (myArrayList.contains("SearchElement")) {
// Do something
}
// Get the size of the list
int myListSize = myArrayList.size();
// Clear the list
myArrayList.clear();
In conclusion, initializing an ArrayList in Java involves specifying the type of objects you want to store, using the ArrayList
constructor, and assigning the result to a variable. With its flexibility, performance, and dynamic size, ArrayList is a popular choice for storing collections of objects in Java.