2D ArrayList in Java W3Schools
2D ArrayList in Java W3Schools
I'm happy to help you with that!
In Java, a 2D array list is typically represented as a nested ArrayList, where each inner list represents a row or column in the 2D array. Here's an example of how you can create and use a 2D ArrayList in Java using W3Schools:
Creating a 2D ArrayList:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<List> twoDArrayList = new ArrayList<>();
for (int i = 0; i < 4; i++) { // Create rows
List tempList = new ArrayList<>();
for (int j = 0; j < 3; j++) { // Create columns
tempList.add(i * 3 + j); // Initialize with some values
}
twoDArrayList.add(tempList);
}
for (int i = 0; i < 2DArrayList.size(); i++) {
System.out.println(twoDArrayList.get(i)); // Print the contents of each row
}
}
}
In this example, we create a 2D ArrayList with four rows and three columns. Each inner list represents a row in the 2D array.
Accessing Elements:
To access an element in a 2D ArrayList, you can use the following syntax:
int value = twoDArrayList.get(0).get(1); // Get the element at (0,1)
In this example, twoDArrayList.get(0)
returns the first inner list (i.e., the first row), and get(1)
returns the second element in that row.
Iterating over a 2D ArrayList:
To iterate over a 2D ArrayList, you can use nested loops:
for (int i = 0; i < twoDArrayList.size(); i++) {
for (int j = 0; j < twoDArrayList.get(i).size(); j++) {
int value = twoDArrayList.get(i).get(j);
System.out.println("Element at (" + i + "," + j + "): " + value);
}
}
This code will print the contents of each element in the 2D ArrayList.
Advantages of Using a 2D ArrayList:
Using a 2D ArrayList can have several advantages, such as:
Flexibility: A 2D ArrayList allows you to easily add or remove elements from any row or column. Efficient memory usage: Unlike traditional 2D arrays, which require contiguous memory allocation, a 2D ArrayList uses pointers to its constituent inner lists, making it more memory-efficient. Easy manipulation of rows and columns: With a 2D ArrayList, you can easily manipulate the contents of individual rows or columns without affecting the rest of the array.Disadvantages of Using a 2D ArrayList:
While a 2D ArrayList offers many advantages, there are also some potential drawbacks to consider:
Performance overhead: Creating and manipulating a 2D ArrayList can be slower than working with traditional 2D arrays due to the overhead of managing the inner lists. Additional memory usage: While the use of pointers makes a 2D ArrayList more efficient in terms of contiguous memory allocation, it still requires additional memory to store the references to the inner lists.Overall, using a 2D ArrayList can be a powerful tool for representing complex data structures in Java. However, it's essential to weigh the advantages and disadvantages before deciding whether this approach is suitable for your specific use case.
How to use for loop for 2D list in Java?
I'd be happy to help!
In Java, a 2D array is an array of arrays, where each inner array represents a row or column in the 2D array. The for
loop can be used to iterate over both the outer and inner arrays.
Here's an example of how to use a for
loop to iterate over a 2D list (which is essentially a 2D array):
int[][] twoDArray = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
// Using a for loop to iterate over the outer array (rows)
for (int i = 0; i < twoDArray.length; i++) {
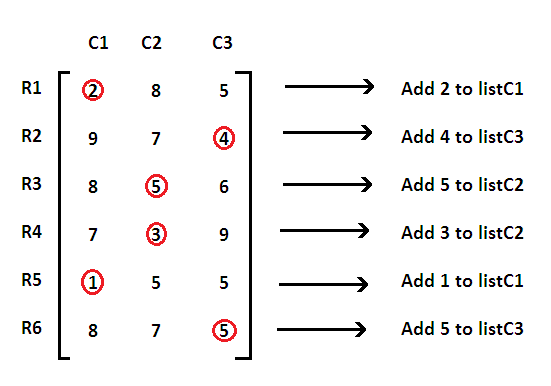
// Print the row
System.out.println(Arrays.toString(twoDArray[i]));
// Now iterate over the inner array (column)
for (int j = 0; j < twoDArray[i].length; j++) {
// Print each element in the column
System.out.print(twoDArray[i][j] + " ");
}
System.out.println();
}
In this example, we first declare and initialize a 2D array twoDArray
with three rows (outer arrays) and three columns (inner arrays). Each inner array represents a row in the 2D array.
The outer loop iterates over each row (i
) in the 2D array. For each row, we print the entire row using Arrays.toString(twoDArray[i])
.
The inner loop iterates over each element (j
) within each row (or column). We use this loop to print out each individual element in the row.
When we run this code, we'll see the output:
[1, 2, 3]
1 2 3
[4, 5, 6]
4 5 6
[7, 8, 9]
7 8 9
As you can see, the outer loop iterates over each row, and the inner loop iterates over each element within that row.
Here are some important notes to keep in mind:
The number of rows (outer arrays) should match the length of the arraytwoDArray.length
. Each inner array should have the same number of elements as the desired column width. You can use either a single loop or multiple loops (as shown here) to iterate over a 2D array. The choice depends on your specific needs and the structure of your data.
I hope this helps!