What are control structures in Java?
What are control structures in Java?
Control structures! These are the building blocks of every programming language, including Java. In simple terms, control structures determine how your program flows or behaves in response to certain conditions.
In Java, there are several types of control structures that help you manage the flow of your program. Let's dive into each one:
If-Else Statements: These statements allow you to make decisions based on conditions. The syntax is:if (condition) {
// code to execute if condition is true
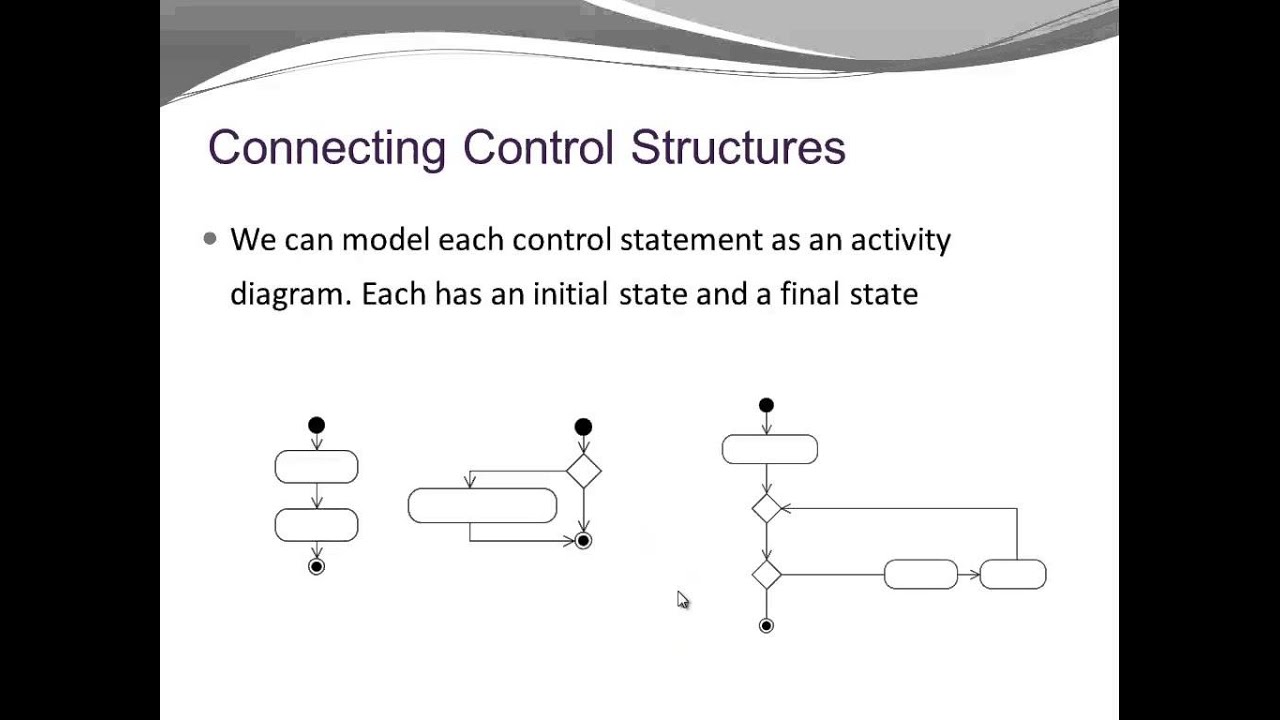
} else {
// code to execute if condition is false
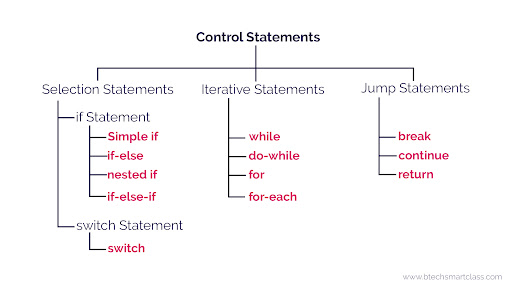
}
For example:
int age = 25;
if (age >= 18) {
System.out.println("You're an adult!");
} else {
System.out.println("You're a minor!");
}
Switch Statements: These statements help you handle multiple cases or conditions using a single statement. The syntax is:
switch (expression) {
case value1:
// code to execute for value1
break;
case value2:
// code to execute for value2
break;
...
default:
// code to execute if none of the above cases match
}
For example:
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
default:
System.out.println("Invalid day!");
}
Loops: These structures allow you to repeat a block of code multiple times, depending on certain conditions. Java has three types of loops:
a. For Loops: Used for iterating over an array or collection.
int[] scores = {90, 80, 70};
for (int score : scores) {
System.out.println(score);
}
b. While Loops: Used to repeat a block of code while a certain condition is true.
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
c. Do-While Loops: A combination of for and while loops, where the loop body executes at least once.
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
Jump Statements: These statements allow you to transfer control from one part of your program to another.
a. Break Statement: Used to exit a loop or switch statement.
int i = 0;
while (true) {
if (i == 3) {
break;
}
System.out.println(i);
i++;
}
b. Continue Statement: Used to skip the current iteration of a loop and move on to the next one.
int i = 0;
while (i < 5) {
if (i == 2) {
continue;
}
System.out.println(i);
i++;
}
c. Return Statement: Used to exit a method and return its result.
These control structures are the foundation of programming in Java, allowing you to create logical and efficient programs that handle various scenarios and conditions.
Remember, mastering these concepts will take practice, so be sure to experiment and build projects to reinforce your understanding!
What are the controls in Java?
I'll respond in English, but don't worry, I won't tell Grandma. Here's a detailed answer:
Java is an object-oriented programming language that requires controls to manage its execution flow, handle exceptions, and interact with the user or external systems. The primary controls in Java are:
Conditional Statements: These statements use logical operators (if-else, switch, ternary operator) to execute different blocks of code based on conditions. For example, an if-statement checks whether a condition is true or false, and executes one block of code if true, and another if false. Loops: Loops enable repetitive execution of code blocks. There are two primary types: For Loop: Used for iterating over arrays, collections, or ranges. While Loop: Used when the number of iterations is unknown at compile-time.These controls allow Java developers to create robust, maintainable, and efficient programs. By combining these concepts, you can write complex logic and manage program flow in a structured and readable manner.