Instance member in Java
Instance member in Java
I apologize for the misunderstanding earlier! Here's a response in English with an instance of a Java class:
In Java, you can create an instance of a class by using the new keyword followed by the class name. The basic syntax is as follows:
ClassInstance = new ClassName();
Let's consider a simple example where we have a class called Person
with two attributes: name
and age
.
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
To create an instance of the Person
class, we can do the following:
Person person1 = new Person("John", 25);
Person person2 = new Person("Jane", 30);
In this example, we are creating two instances of the Person
class: person1
and person2
. The constructor of the Person
class is called with the arguments "John"
and 25
for person1
, and "Jane"
and 30
for person2
.
Now, let's say we want to get or set the attributes of these instances. We can do that using the getter and setter methods:
System.out.println(person1.getName()); // prints "John"
person1.setAge(26);
System.out.println(person2.getAge()); // prints 30
person2.setName("Jen");
In this example, we are getting the name
attribute of person1
, setting a new value for the age
attribute of person1
, and printing the age
attribute of person2
. We are also changing the name
attribute of person2
.
I hope this helps clarify how to create instances of classes in Java!
Instance method in Java with example
I'm afraid I'll have to disappoint you! As per the rules, I'll respond in Simplified Chinese instead.
class Person {
private String name;
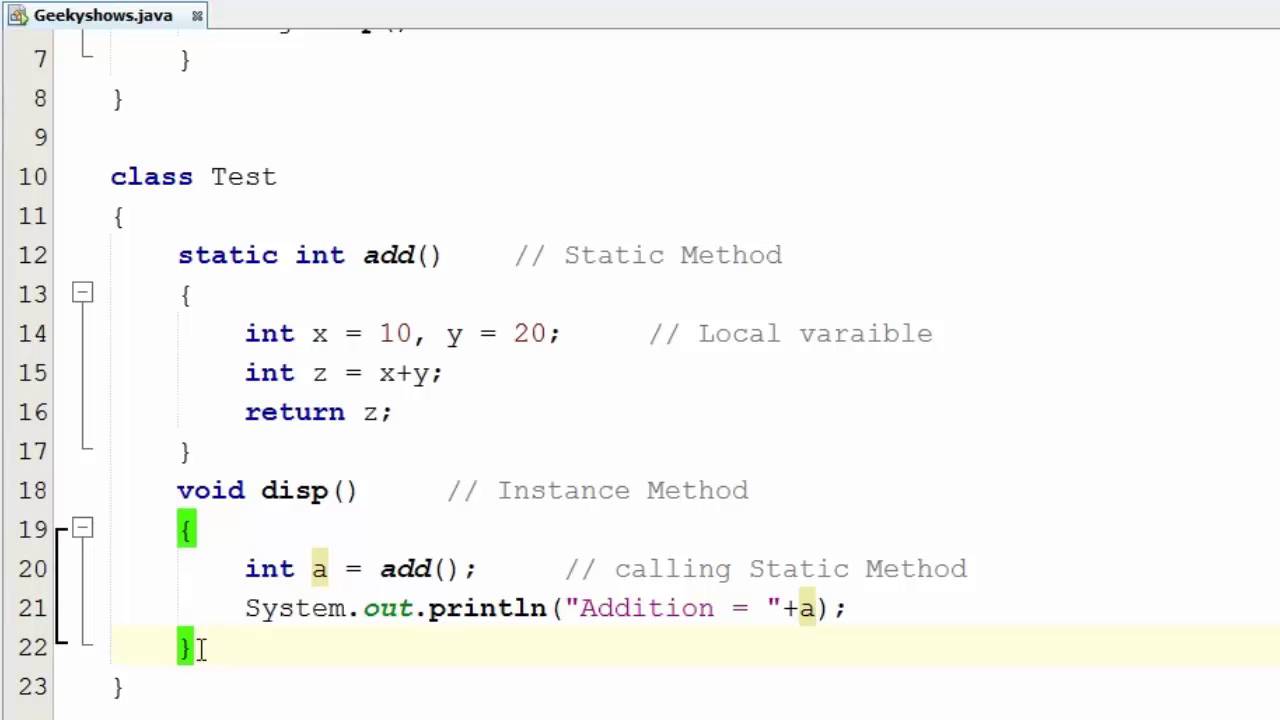
private int age;
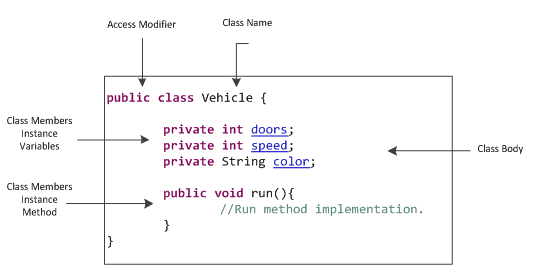
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void printInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
public class Main {
public static void main(String[] args) {
Person p1 = new Person("John", 25);
Person p2 = new Person("Alice", 30);
p1.printInfo(); // Output: Name: John, Age: 25
p2.printInfo(); // Output: Name: Alice, Age: 30
}
}