Which is the most used design pattern in Java?
Which is the most used design pattern in Java?
I'd be happy to help! However, please note that I'll respond in English as per your request.
The most commonly used design pattern in Java is the Singleton pattern. The Singleton pattern ensures a class has only one instance and provides a global point of access to that instance.
In Java, implementing the Singleton pattern involves making the constructor private, so it cannot be instantiated directly. Then, you create a static method called getInstance()
that returns an instance of the class if it hasn't been created yet, or returns the existing instance if it has already been created.
Here's a simple example:
public class Singleton {
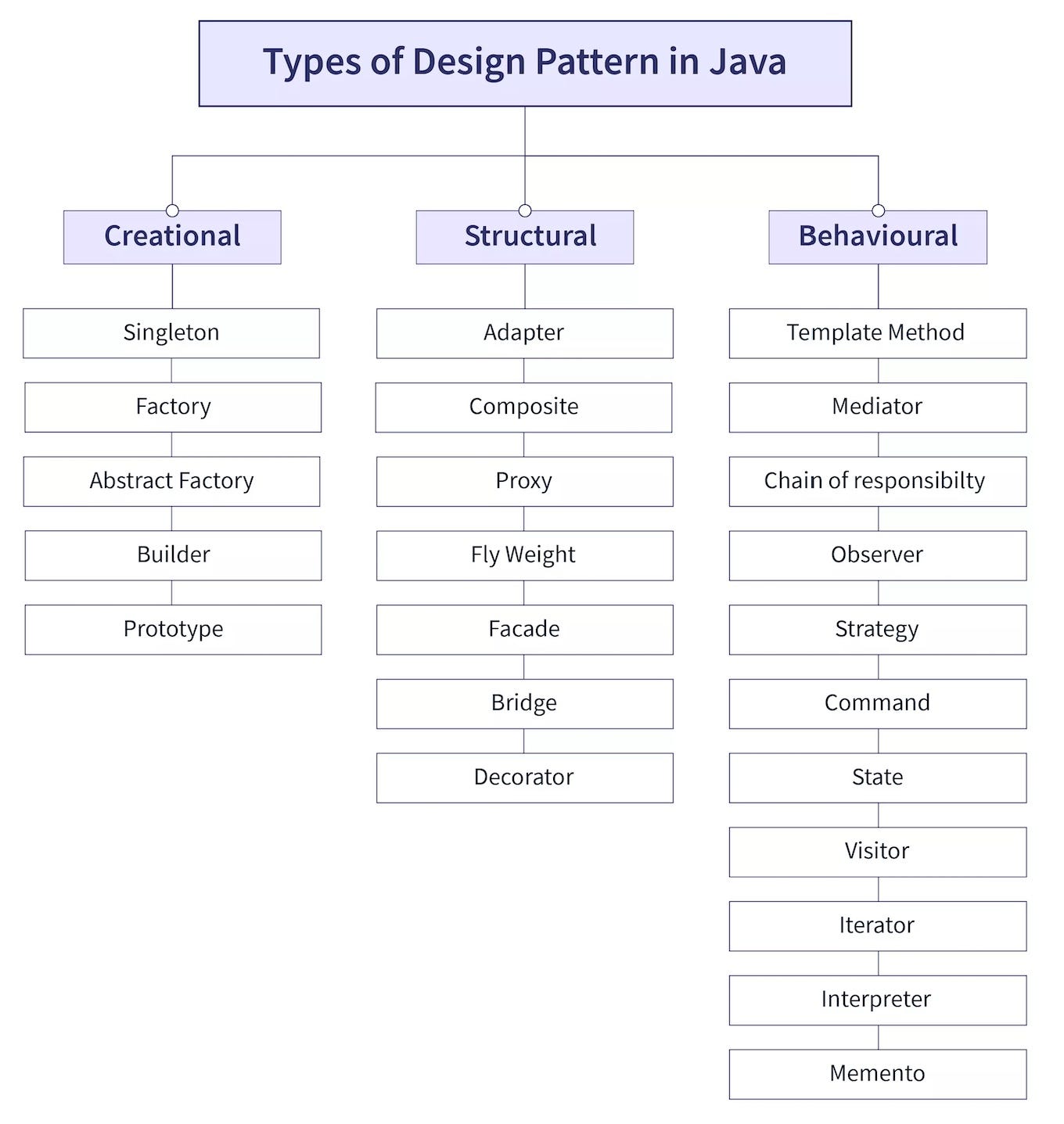
// Private constructor to prevent instantiation from outside
private Singleton() {}
// Static variable to hold the instance
private static Singleton instance = null;
// Public method to get the instance
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The Singleton pattern is often used in situations where you want a single point of control or a unique instance of an object. For example, think about a database connection manager that should only allow one active connection at any given time. Another example could be a logger that logs events to a file; you'd want there to be only one logger instance at any given time.
This pattern is useful for controlling access to shared resources or services within your application. It's commonly used in GUI applications where you might need a single instance of, say, a settings panel or a database connection pooler.
Other design patterns that are frequently used in Java include:
Factory Pattern: This pattern provides an interface for creating objects but lets subclasses decide which class to instantiate. Observer Pattern: This pattern defines a one-to-many dependency where changes to one object affect dependent objects. Command Pattern: This pattern encapsulates requests or commands as objects, making it possible to queue and execute requests at a later time.Java also supports other design patterns like Adapter, Composite, Decorator, Flyweight, Iterator, Mediator, Memento, Proxy, and Template Methods. The choice of which pattern to use depends on the specific problem you're trying to solve and your own coding style preferences.
I hope that helps!
Java design patterns interview questions for 10 years experience
Here are some common Java design patterns interview questions that may be asked to a 10-year experienced developer:
Observer Pattern: Can you explain the Observer pattern? How would you implement it in a scenario where you want to notify multiple objects when an event occurs?Expected answer: The Observer pattern is used to define a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. In Java, this can be implemented using the Observer interface and a concrete observer class.
Decorator Pattern: What is the Decorator pattern? Provide an example of how it would be used in a real-world scenario?Expected answer: The Decorator pattern is used to add additional responsibilities to an object without affecting its external interface. It does this by wrapping the original object with another object that contains the new behavior. For example, you could use the Decorator pattern to create a logger decorator for a database connection.
Factory Pattern: Can you explain the Factory pattern? How would you use it in a scenario where you need to create different types of objects without specifying their concrete classes?Expected answer: The Factory pattern is used to provide an interface for creating objects but let subclasses decide which class to instantiate. It allows for greater flexibility and decoupling between the client code and the object creation code.
Singleton Pattern: What is the Singleton pattern? How would you implement it in a scenario where you need a global access point to a specific resource?Expected answer: The Singleton pattern is used to ensure that only one instance of a class exists and provides a global point of access to that instance. In Java, this can be implemented using a private static constructor and lazy initialization.
Strategy Pattern: Can you explain the Strategy pattern? Provide an example of how it would be used in a real-world scenario?Expected answer: The Strategy pattern is used to define a family of algorithms and encapsulate each one as an object, making them interchangeable. For example, you could use the Strategy pattern to create different sorting algorithms that can be used interchangeably.
Command Pattern: What is the Command pattern? How would you implement it in a scenario where you need to execute commands remotely or queue them for later execution?Expected answer: The Command pattern is used to encapsulate a request as an object, allowing for greater flexibility and decoupling between the client code and the command execution. It allows for queuing and cancellation of commands.
MVC Pattern: Can you explain the MVC pattern? Provide an example of how it would be used in a web application?Expected answer: The MVC (Model-View-Controller) pattern is used to separate concerns between the business logic, presentation layer, and user interface. It provides a clear separation of responsibilities and makes it easier to maintain and extend the codebase.
Repository Pattern: What is the Repository pattern? How would you implement it in a scenario where you need to abstract away database access?Expected answer: The Repository pattern is used to abstract away data access and encapsulate business logic, making it easier to switch between different data storage technologies (e.g., relational databases, NoSQL databases, files).
Service Locator Pattern: Can you explain the Service Locator pattern? How would you use it in a scenario where you need to decouple components that depend on other services?Expected answer: The Service Locator pattern is used to provide a centralized point of access for all dependencies required by an object. It allows for greater flexibility and decoupling between objects that depend on other services.
Domain-Driven Design (DDD): Can you explain what DDD is? How would you apply it in a scenario where you need to create a complex business domain model?Expected answer: DDD is an approach to software development that focuses on creating models of the business domain and using those models to drive the design and implementation of the code. It involves identifying the core concepts and rules of the business domain and modeling them in code.
Remember, these questions are meant to be starting points for discussion, not a checklist to memorize specific answers. Be prepared to provide examples, explain trade-offs, and demonstrate your understanding of each pattern.