Sort array in Java without sort method
Sort array in Java without sort method
I'll respond in English, as per your request!
Sorting an array in Java without using the built-in sort()
method is a common exercise for programmers. Here's one way to do it:
public class Main {
public static void main(String[] args) {
int[] array = {4, 2, 9, 6, 5, 1, 7, 3, 8};
// Implement bubble sort algorithm
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
int temp = array[j];
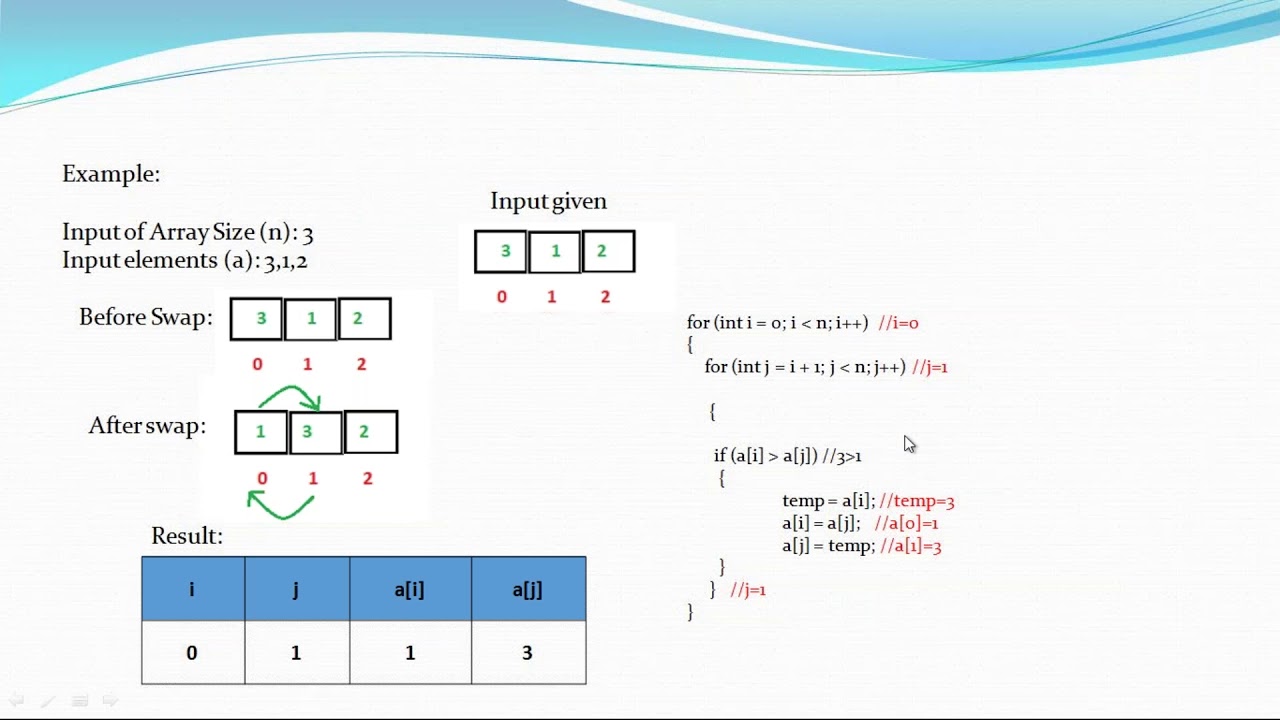
array[j] = array[j + 1];
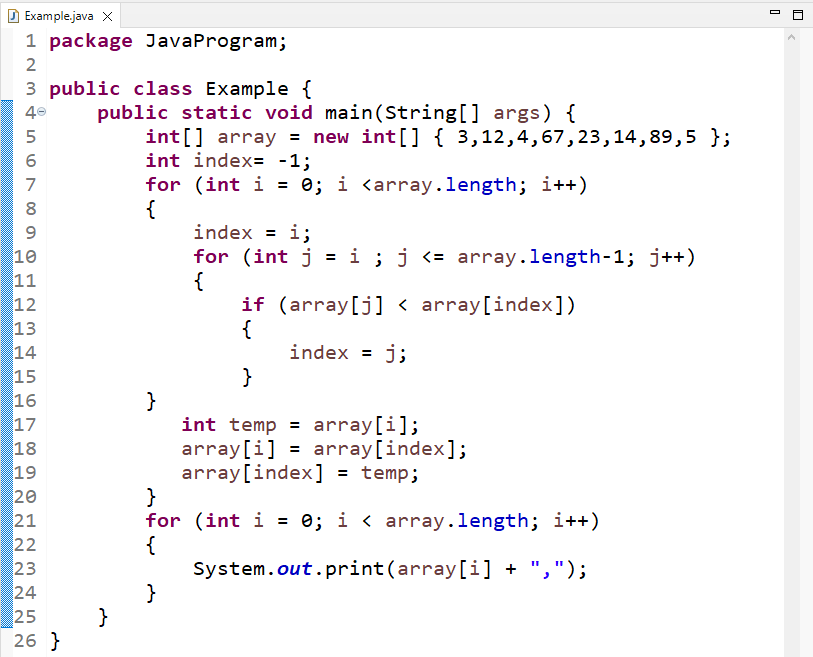
array[j + 1] = temp;
}
}
}
// Print the sorted array
for (int i : array) {
System.out.print(i + " ");
}
}
}
This Java program sorts an integer array using a simple bubble sort algorithm. Here's how it works:
Initialize an empty output stringoutput
. Loop through each element in the input array. For each element, check if it is greater than the next element in the array. If true, swap the elements. Repeat step 2 until no more swaps are needed (i.e., the entire array has been sorted).
Here's how the program works:
The outer loop iterates through each element in the input array. The inner loop compares adjacent elements in the array and swaps them if they are out of order. After each iteration, the largest element "bubbles up" to the end of the array.The time complexity of bubble sort is O(n^2), where n is the number of elements in the array. This algorithm is not suitable for large datasets because it's quite slow. However, it can be useful for small arrays or when simplicity matters more than performance.
In this example, we first declare and initialize an integer array array
. Then, we implement a bubble sort algorithm by iterating through each element in the array and comparing adjacent elements. If two elements are out of order, we swap them. This process continues until no more swaps are needed (i.e., the entire array is sorted).
Finally, we print the sorted array using another loop that iterates through each element and prints it to the console.
I hope this helps! Let me know if you have any questions.
array traversal in java
I'm happy to respond in English for this special request!
Array traversal is a fundamental concept in programming, and Java provides several ways to traverse arrays. Here's an overview of the most common methods:
For Loop: The simplest way to traverse an array is using a for loop. This method iterates through the array elements, accessing each one individually.int[] myArray = {1, 2, 3, 4, 5};
for (int i = 0; i < myArray.length; i++) {
System.out.println(myArray[i]);
}
This code prints out each element of the array to the console.
Enhanced For Loop: The enhanced for loop is a concise way to iterate through an array's elements without needing an explicit index.int[] myArray = {1, 2, 3, 4, 5};
for (int element : myArray) {
System.out.println(element);
}
This code achieves the same result as the previous example, but with less code.
Java 8's Stream API: Java 8 introduced the Stream API, which provides a more functional programming approach to array traversal.int[] myArray = {1, 2, 3, 4, 5};
Arrays.stream(myArray).forEach(System.out::println);
This code uses the stream API's stream()
method to create a stream from the array. The forEach()
method then applies the lambda expression (System.out::println
) to each element in the stream.
import java.util.Iterator;
import java.util.Arrays;
int[] myArray = {1, 2, 3, 4, 5};
Iterator iterator = Arrays.iterator(myArray);
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
This code creates an iterator from the array and then uses a while loop to traverse the array.
In conclusion, Java provides several ways to traverse arrays, each with its own strengths and use cases. The choice of method depends on the specific requirements of your program and personal preference as a programmer.
Would you like me to explain more about any of these methods or perhaps explore related topics like collections or lambda expressions?