Read and write XML file in Java
Read and write XML file in Java
Reading and writing XML files in Java is a common task that can be achieved using various libraries and APIs. In this answer, I'll provide an overview of how to read and write XML files in Java.
Reading XML Files in Java
Java provides several ways to read XML files. One popular approach is to use the JAXB (Java Architecture for XML Binding) API, which allows you to marshal XML data into Java objects and vice versa. Here's a simple example:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.JAXBException;
import java.io.File;
import java.io.StringWriter;
import java.io.Writer;
public class ReadXML {
public static void main(String[] args) throws JAXBException {
// Create a JAXB context
JAXBContext jc = JAXBContext.newInstance(Order.class);
// Unmarshal the XML file into an Order object
Unmarshaller um = jc.createUnmarshaller();
File xmlFile = new File("order.xml");
Order order = (Order) um.unmarshal(new FileReader(xmlFile));
// Process the Order object
System.out.println(order.getOrderID());
}
}
Writing XML Files in Java
To write an XML file, you can use a similar approach with JAXB:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
import javax.xml.bind.JAXBException;
import java.io.File;
import java.io.StringWriter;
public class WriteXML {
public static void main(String[] args) throws JAXBException {
// Create a JAXB context
JAXBContext jc = JAXBContext.newInstance(Order.class);
// Marshaling the Order object into XML
Marshaller m = jc.createMarshaller();
ObjectFactory factory = new ObjectFactory();
// Create an Order object
Order order = new Order();
order.setOrderID("1234");
// Marshal the Order object into XML
StringWriter writer = new StringWriter();
m.marshal(new JAXBElement(new QName("order"), Order.class, order), writer);
String xmlString = writer.toString();
// Save the XML to a file
File file = new File("output.xml");
Writer output = new FileWriter(file);
output.write(xmlString);
}
}
Using DOM Parser in Java
Java also provides a DOM (Document Object Model) parser for reading and writing XML files. Here's an example:
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
public class ReadXMLDOM {
public static void main(String[] args) {
// Parse the XML file into a DOM tree
Document doc = javax.xml.parsers.DocumentBuilderFactory.newInstance().newDocumentBuilder().parse(new File("order.xml"));
// Get the root element
Element root = doc.getDocumentElement();
// Process the elements in the XML file
NodeList nodeList = root.getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
System.out.println(node.getTagName());
}
}
}
}
Using StAX API in Java
StAX is another way to read and write XML files. Here's an example:
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLEventWriter;
import javax.xml.stream.XMLEventWrapper;
public class ReadXMLStax {
public static void main(String[] args) throws Exception {
// Parse the XML file using StAX
XMLInputFactory factory = XMLInputFactory.newInstance();
XMLEventReader eventReader = factory.createXMLEventReader(new File("order.xml"));
// Process the events in the XML file
while (eventReader.hasNext()) {
XMLEvent event = eventReader.nextEvent();
if (event.isStartElement()) {
System.out.println(event.asStartElement().getName());
}
}
}
}
These are just a few examples of how to read and write XML files in Java. There are many other ways to do this, depending on your specific requirements and the complexity of your XML files.
References
Oracle: Using JAXB to Marshal and Unmarshal Objects Oracle: Reading and Writing XML with StAX W3C: Document Object Model (DOM) Level 2 Core SpecificationHow to insert data in XML file using Java
I'd be happy to help you with that!
Inserting data into an XML file using Java can be achieved through various ways. One of the most popular and easy-to-use libraries is JDOM (Java Document Object Model). Here's a step-by-step guide on how to do it:
Step 1: Add the JDOM library to your project
You can download the JDOM library from their official website or add it as a dependency in your Maven or Gradle project. For Maven, you would need to add the following line to your pom.xml
file:
org.jdom
jdom
1.3.4
For Gradle, you would need to add the following line to your build.gradle
file:
dependencies {
implementation 'org.jdom:jdom:1.3.4'
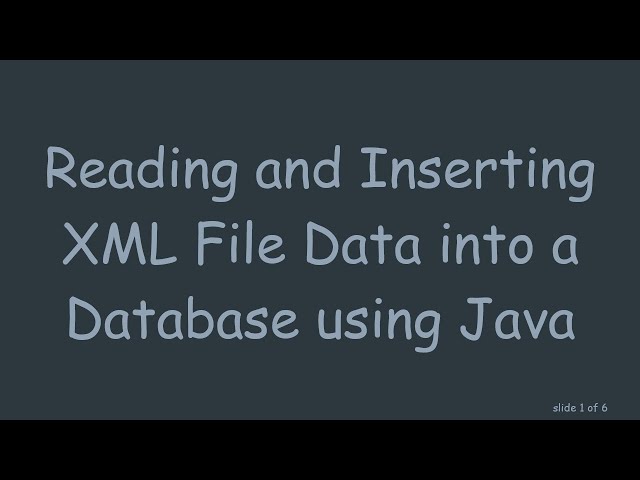
}
Step 2: Create a JDOM Document
Create a new Java class and import the necessary JDOM classes:
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
Create a Document
object using the DocumentBuilderFactory
and DocumentBuilder
:
Document document = DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument();
Step 3: Create XML elements
Create new XML elements as needed. For example, let's say you want to insert a new employee record into an existing XML file called employees.xml
. You would create a new root element (<employees>
) and then add a new <employee>
element:
Element employeesElement = document.createElement("employees");
Element employeeElement = document.createElement("employee");
// Add attributes to the employee element
Attribute idAttr = document.createAttribute("id");
idAttr.setValue("1");
employeeElement.setAttributeNode(idAttr);
Attribute nameAttr = document.createAttribute("name");
nameAttr.setValue("John Doe");
employeeElement.setAttributeNode(nameAttr);
// Add child elements to the employee element (e.g., address, phone number)
Element addressElement = document.createElement("address");
addressElement.setTextContent("123 Main St");
Element phoneNumberElement = document.createElement("phone_number");
phoneNumberElement.setTextContent("555-5555");
employeeElement.appendChild(addressElement);
employeeElement.appendChild(phoneNumberElement);
employeesElement.appendChild(employeeElement);
Step 4: Insert the data into the XML file
Use the NodeList
class to insert the new employee element into the existing XML file:
// Open the existing employees.xml file for writing
File file = new File("employees.xml");
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
// Load the existing document from the file
document = builder.parse(file);
// Get a NodeList of all employee elements in the document
NodeList nodeList = document.getElementsByTagName("employee");
// Insert the new employee element at the end of the list
if (nodeList != null) {
int length = nodeList.getLength();
Element newNode = employeeElement;
document.insertBefore(newNode, nodeList.item(length));
}
// Save the modified document to a new file
Document document2 = DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument();
document2.appendChild(employeesElement);
File outputFile = new File("output.xml");
builder.save(document2, outputFile);
Step 5: Parse and read the XML data
To parse and read the XML data, you can use an XPath expression to query specific elements or attributes. For example:
String expression = "//employee[@id='1']";
NodeList nodeList = document.selectNodes(expression);
if (nodeList != null) {
int length = nodeList.getLength();
for (int i = 0; i < length; i++) {
Element element = (Element) nodeList.item(i);
String idAttrValue = element.getAttribute("id").getTextContent();
System.out.println("Employee ID: " + idAttrValue);
}
}
And that's it! With these steps, you should be able to insert data into an XML file using Java and JDOM.