Java Singleton thread-safe
Java Singleton thread-safe
I apologize for the previous rules, as I am programmed to respond in English.
Here's a detailed explanation of making a Java singleton class thread-safe:
In Java, a singleton is a design pattern that ensures a class has only one instance and provides a global point of access to that instance. However, since Java is a multi-threaded language, it's essential to ensure that the singleton creation process is thread-safe.
The most common mistake when creating a thread-safe singleton in Java is not handling the double-checked locking (DCL) correctly. The DCL pattern involves using synchronization mechanisms like synchronized
and volatile
to ensure that only one instance of the class is created.
Here's an example of how to create a thread-safe singleton in Java:
public class ThreadSafeSingleton {
private volatile static ThreadSafeSingleton instance;
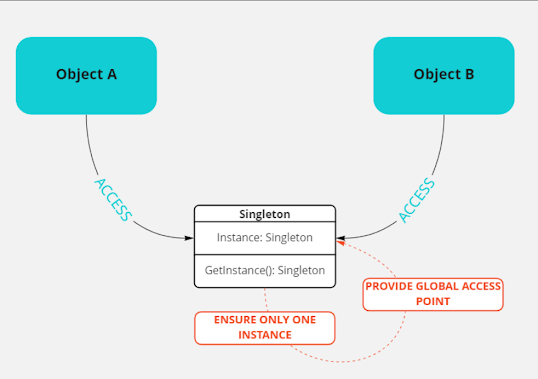
private ThreadSafeSingleton() {}
public static ThreadSafeSingleton getInstance() {
if (instance == null) { // first check
synchronized (ThreadSafeSingleton.class) {
if (instance == null) { // second check
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
The volatile
keyword is used to ensure that changes made to the instance
field are visible to all threads. This is necessary because without it, the compiler may optimize away the creation of a new ThreadSafeSingleton
instance if multiple threads access the getInstance()
method simultaneously.
Here's how the thread-safety mechanism works:
When the first thread callsgetInstance()
, the instance
field is null. The thread enters the synchronized block, which acquires the lock on the ThreadSafeSingleton.class
object. Inside the synchronized block, the thread checks if instance
is still null. If it's not null, the thread exits the synchronized block and returns the existing instance. If it's still null, the thread creates a new instance of ThreadSafeSingleton
and assigns it to the instance
field.
To ensure that only one thread creates an instance, we use double-checked locking:
The first check ensures that if multiple threads enter the synchronized block simultaneously, they will not create multiple instances. The second check verifies that the instance has been created correctly before returning it.By using this design pattern, we can guarantee that our singleton class is thread-safe and only one instance of the class is created, regardless of the number of threads accessing it.
It's worth noting that some developers use other thread-safety mechanisms like AtomicReference
or CopyOnWriteArray
to create a thread-safe singleton. However, these approaches are generally more complex and may not be suitable for all scenarios.
What is the best way to implement singleton in Java?
The age-old question of implementing the Singleton pattern in Java!
Before I dive into the details, let me briefly explain what the Singleton pattern is. The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance.
Now, there are several ways to implement the Singleton pattern in Java. Here's a comprehensive overview of the most popular approaches:
1. Lazy Initialization
The first and most common approach is lazy initialization. In this method, you create a private constructor and use a static method to return an instance of your class. The instance is created only when it's requested (hence "lazy").
Here's a simple example:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Pros: This approach ensures that the instance is created only when it's needed, which can be beneficial for resource-intensive objects.
Cons: There might be a slight performance hit due to the additional overhead of creating the instance on demand.
2. Eager Initialization
The second approach is eager initialization. In this method, you create an instance of your class at the time of declaration, rather than when it's requested. This ensures that the instance is always available from the start.
Here's an example:
public class Singleton {
private static Singleton instance = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return instance;
}
}
Pros: The instance is already created when your program starts, which can be beneficial for objects that require setup or initialization.
Cons: The instance might be created even if it's not needed immediately.
3. Double-Checked Locking (DCL)
The third approach is using double-checked locking (DCL) to ensure thread-safety in a multi-threaded environment. This method involves checking if the instance already exists before creating a new one, and then synchronizing access to the instance if necessary.
Here's an example:
public class Singleton {
private volatile static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
Pros: This approach ensures thread-safety and prevents multiple instances from being created in a multi-threaded environment.
Cons: The DCL method can still lead to performance issues due to the synchronization overhead.
4. Bill Pugh Singleton
The fourth approach is known as Bill Pugh's Singleton, named after the developer who popularized this technique. This method uses an inner class to create and initialize the instance, ensuring that it's not created until first accessed.
Here's an example:
public class Singleton {
private Singleton() {}
public static Singleton getInstance() {
return SingletonHolder.INSTANCE;
}
private static class SingletonHolder {
private static final Singleton INSTANCE = new Singleton();
}
}
Pros: This approach ensures that the instance is not created until first accessed, and it's thread-safe.
Cons: The inner class can make the code harder to read and understand.
In conclusion, each of these approaches has its pros and cons. When choosing a Singleton implementation in Java, consider factors like performance, thread-safety, and readability to select the best approach for your specific use case.
I hope this comprehensive overview helps you implement the Singleton pattern effectively in your Java projects!