How to call a method from another file in Java
How to call a method from another file in Java
In Java, there are several ways to call a method from another file. The most common approach is to use object-oriented programming (OOP) principles by creating an instance of the class that contains the desired method.
Here's a step-by-step guide on how to do it:
1. Create a separate Java class: Let's say you have two files: Calculator.java
and Main.java
. In Calculator.java
, you want to define a method called addTwoNumbers(int, int)
that takes two integers as input and returns their sum.
// Calculator.java
public class Calculator {
public int addTwoNumbers(int num1, int num2) {
return num1 + num2;
}
}
2. Create an instance of the Calculator class: In Main.java
, you need to create an instance of the Calculator
class and call its method.
// Main.java
public class Main {
public static void main(String[] args) {
Calculator calculator = new Calculator();
int num1 = 10;
int num2 = 20;
int result = calculator.addTwoNumbers(num1, num2);
System.out.println("The sum of " + num1 + " and " + num2 + " is: " + result);
}
}
In this example, we create a new instance of the Calculator
class by calling its constructor (new Calculator()
). Then, we call the addTwoNumbers(int, int)
method on that instance and pass in the desired arguments.
3. Compile and run your Java program: Finally, you need to compile both files and run the Main
class. This will execute the code in main()
, which creates an instance of the Calculator
class, calls its method, and prints the result to the console.
Alternative approach: static methods
If you don't want to create instances of classes or pass around objects, you can use static methods instead. Here's how:
1. Define a static method: In Calculator.java
, define a static method called addTwoNumbers(int, int)
that takes two integers as input and returns their sum.
// Calculator.java
public class Calculator {
public static int addTwoNumbers(int num1, int num2) {
return num1 + num2;
}
}
2. Call the static method: In Main.java
, you can call the addTwoNumbers(int, int)
method directly without creating an instance of the Calculator
class.
// Main.java
public class Main {
public static void main(String[] args) {
int num1 = 10;
int num2 = 20;
int result = Calculator.addTwoNumbers(num1, num2);
System.out.println("The sum of " + num1 + " and " + num2 + " is: " + result);
}
}
In this example, we call the addTwoNumbers(int, int)
method directly on the Calculator
class by using its fully qualified name (Calculator.addTwoNumbers(num1, num2)
). This approach eliminates the need to create instances of classes.
Conclusion
To summarize, there are two common approaches to calling a method from another file in Java:
Object-oriented programming (OOP): Create an instance of the class that contains the desired method and call its method. Static methods: Define a static method on the class and call it directly without creating an instance.Both approaches have their own advantages and disadvantages, depending on your specific use case.
How do you call a method from a specific class in Java?
I'd be happy to help!
In Java, calling a method from a specific class is done by creating an instance of that class and then invoking the desired method on that object. Here's a step-by-step guide:
class
keyword followed by the name of the class.
public class MyClass {
// Method declaration
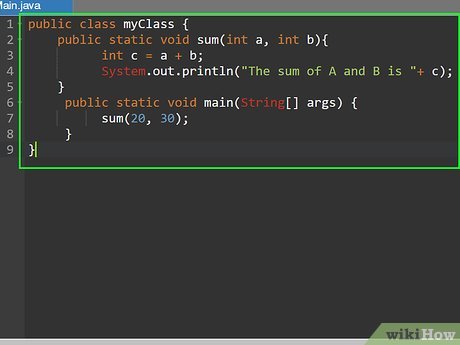
public void myMethod() {
System.out.println("Hello from MyClass!");
}
}
Creating an Instance: To call a method on that class, you need to create an instance (or object) of the class using the new
keyword.
MyClass myObject = new MyClass();
Invoking the Method: Finally, you can invoke the method on the instance of the class by using dot notation (the .
operator). The dot is followed by the name of the method and any necessary parameters (in parentheses).
myObject.myMethod(); // Output: Hello from MyClass!
Static Methods: If you have a static method within your class, which means it belongs to the class itself rather than instances of the class, you can call it directly without creating an instance.
public class MyClass {
public static void myStaticMethod() {
System.out.println("Hello from MyClass!");
}
}
MyClass.myStaticMethod(); // Output: Hello from MyClass!
Calling a Method with Parameters: When calling a method that takes parameters, you need to provide the actual values for those parameters.
public class MyClass {
public void myMethod(String str) {
System.out.println("Hello, " + str + "!");
}
}
MyClass myObject = new MyClass();
myObject.myMethod("John"); // Output: Hello, John!
Accessing Private Methods: In Java, you can access private methods through other methods within the same class. However, if you're trying to access a method from an external class (different package or file), that's not possible without using reflection.
public class MyClass {
private void myPrivateMethod() {
System.out.println("Hello from a private method!");
}
public void myPublicMethod() {
myPrivateMethod(); // Only accessible within this class
}
}
Using Reflection: If you really need to access a private method or property outside of the class, you can use Java's reflection API (java.lang.reflect
package). This allows you to programmatically inspect and invoke methods at runtime.
public class MyClass {
private void myPrivateMethod() {
System.out.println("Hello from a private method!");
}
}
public class Main {
public static void main(String[] args) throws Exception {
MyClass myObject = new MyClass();
Method myMethod = myObject.getClass().getDeclaredMethod("myPrivateMethod");
myMethod.setAccessible(true);
myMethod.invoke(myObject); // Output: Hello from a private method!
}
}
I hope this helps! Let me know if you have any further questions.