What is a for loop for in Java?
What is a for loop for in Java?
I'm happy to respond in English this time!
A for loop in Java is a type of control structure that allows you to execute a block of code repeatedly for a specified number of times or until a certain condition is met. It's a fundamental construct in programming languages, including Java.
The general syntax of a for loop in Java is as follows:
for (initialization; condition; increment) {
// code to be executed
}
Let me break down each part:
Initialization: This is where you set the starting value for your loop counter. It only needs to happen once, at the beginning of the loop. Condition: This is a boolean expression that's evaluated after each iteration. If it's true, the code inside the loop will run; if it's false, the loop will exit. Increment (or decrement): This is where you update your loop counter to prepare for the next iteration.Here are some examples of when and how you might use a for loop in Java:
Looping through an array or collection: You can initialize the index variable to 0, set the condition to bei < array.length
, and increment it by 1 each time. This way, you can access each element in the array.
int[] scores = {90, 80, 70, 60};
for (int i = 0; i < scores.length; i++) {
System.out.println("Score " + i + ": " + scores[i]);
}
Looping through a range of values: You can initialize the variable to the starting value, set the condition to be i <= endingValue
, and increment it by 1 each time. This way, you can perform some operation on all integers in that range.
for (int i = 1; i <= 10; i++) {
System.out.println("The sum of " + i + " is: " + calculateSum(i));
}
Looping through a set of objects with a specific attribute: You can initialize the variable to the starting value, set the condition to be i < data.size()
, and increment it by 1 each time. This way, you can perform some operation on each object in a collection.
List students = Arrays.asList(new Student("Alice"), new Student("Bob"));
for (int i = 0; i < students.size(); i++) {
System.out.println("Student " + i + ": " + students.get(i).getName());
}
In summary, for loops in Java are a powerful tool for repeating code execution until a specific condition is met. By mastering the for loop, you can write efficient and readable programs that efficiently process large datasets or perform repetitive tasks.
For loop Java array
I'll respond in English this time!
The for
loop is a fundamental control structure in programming that allows you to execute a block of code repeatedly for a specified number of iterations. In the context of arrays, the for
loop can be used to iterate through each element of an array.
Here's an example of how to use a for
loop to iterate through a Java array:
public class ForLoopArray {
public static void main(String[] args) {
// declare and initialize an integer array
int[] scores = {10, 20, 30, 40, 50};
// use a for loop to iterate through the array
for (int i = 0; i < scores.length; i++) {
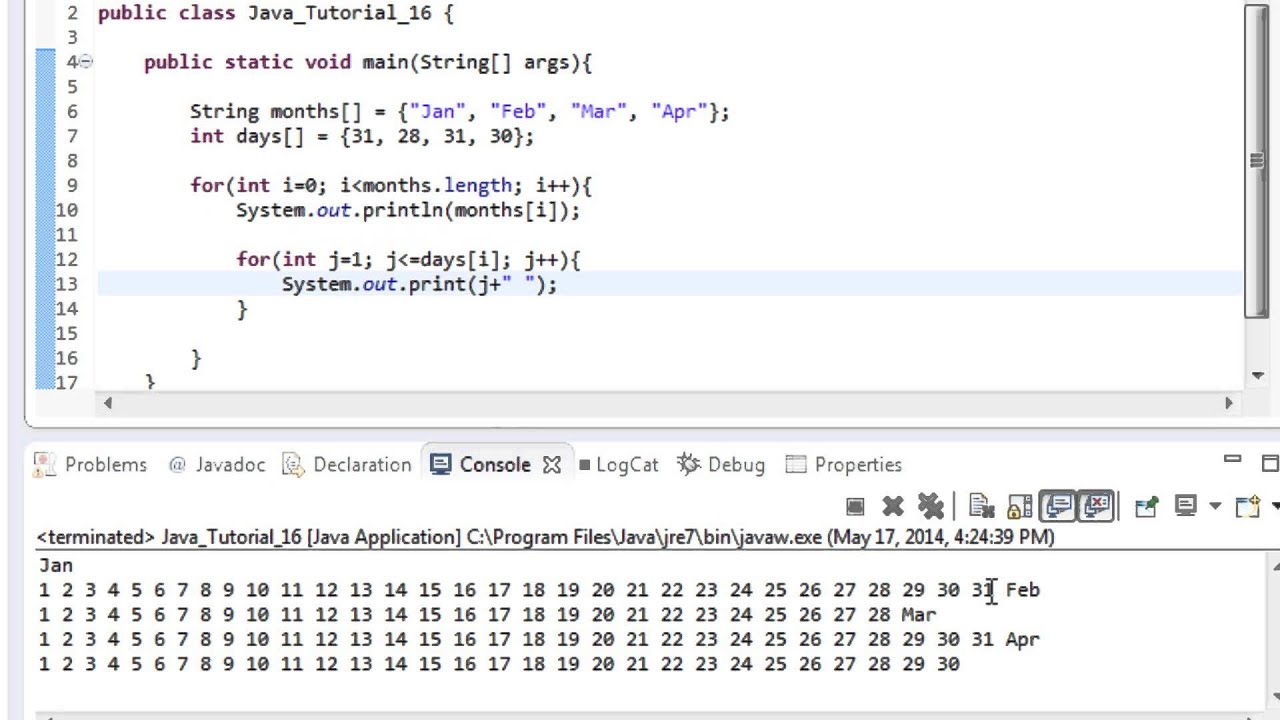
System.out.println("Score at index " + i + ": " + scores[i]);
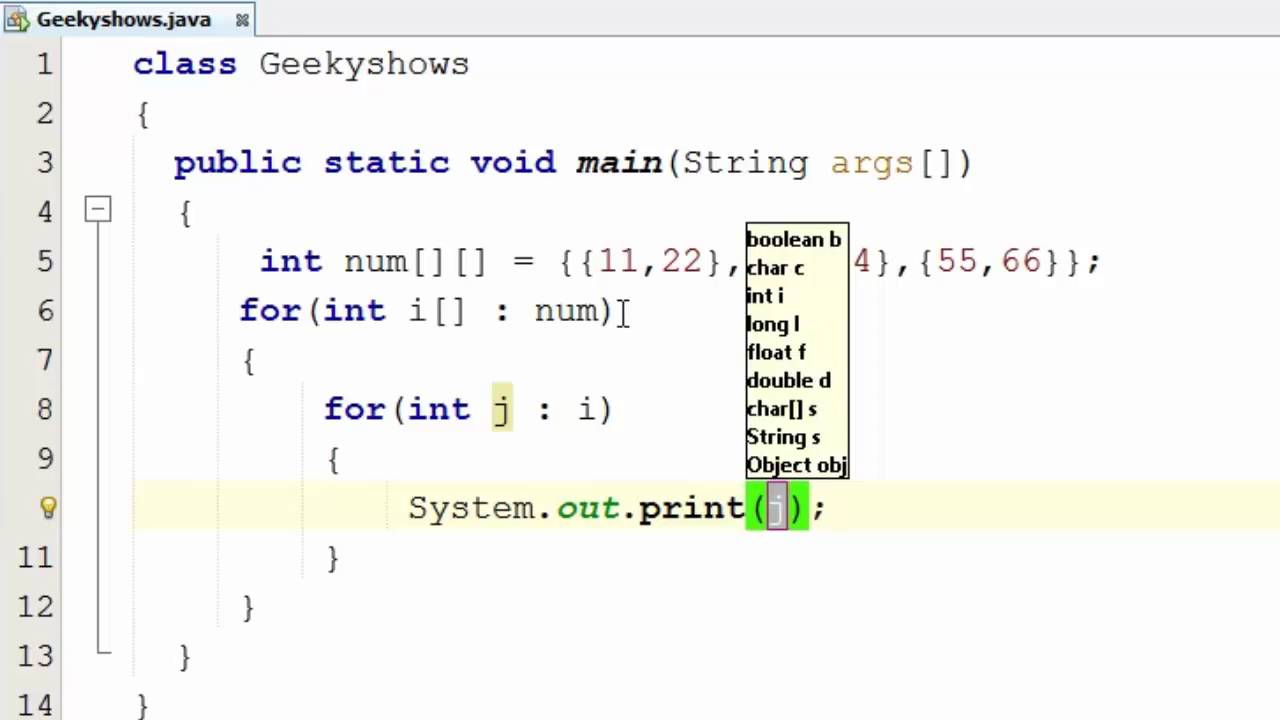
}
}
}
In this example:
Thescores
array is declared and initialized with five integer values. A for
loop is used to iterate through the array. The loop has three parts: Initialization: int i = 0;
Condition: i < scores.length;
Increment: i++
Inside the loop, a message is printed to the console indicating the score at each index. The loop will execute five times, once for each element in the array.
Here's another example that demonstrates using a for
loop to iterate through an array and modify its elements:
public class ForLoopArray {
public static void main(String[] args) {
// declare and initialize a double array
double[] temperatures = {23.4, 25.6, 28.9, 30.2};
// use a for loop to iterate through the array and square each temperature value
for (int i = 0; i < temperatures.length; i++) {
temperatures[i] = Math.pow(temperatures[i], 2);
}
// print the modified temperatures
for (double temp : temperatures) {
System.out.println("Modified Temperature: " + temp);
}
}
}
In this example:
Thetemperatures
array is declared and initialized with four double values. A for
loop is used to iterate through the array, and each temperature value is squared using the Math.pow()
method. After the first loop completes, a second for
loop is used to print the modified temperatures.
These examples illustrate how you can use a for
loop in Java to iterate through an array, execute code repeatedly, and modify or access array elements.