Can we have static variable in a method in java eclipse
Can we have static variable in a method in java eclipse
I'd be happy to help!
In Java, you can definitely define a static variable within a method. However, there are some important considerations to keep in mind.
A static variable is a variable that is shared by all instances of the same class. Static variables are initialized only once, when the program starts, and they retain their values between method calls. This makes them useful for storing settings or constants that don't change during the program's execution.
Now, when you define a static variable within a method in Java, it doesn't behave exactly like other static variables. The reason is that methods are instance-specific, whereas static variables are class-specific. So, if you declare a static variable within a method, it will only be accessible within that method and will not be shared between different instances of the same class.
Here's an example:
public class MyClass {
public void myMethod() {
static int myStaticVariable = 0; // This is okay!
myStaticVariable++; // Incrementing the variable
System.out.println(myStaticVariable); // Print the value
}
}
In this example, myStaticVariable
is a static variable declared within the myMethod()
method. It can be accessed and modified within that method. However, if you try to access myStaticVariable
from another method or class, it will give you a compilation error because it's not accessible outside the scope of myMethod()
.
One important thing to note is that Java compilers (including Eclipse) treat local variables (declared within methods) as instance-specific by default. So, even if you declare a static variable within a method, it won't behave like other static variables because it's still an instance-specific variable.
If you want to define a true class-level static variable, you should declare it outside any method, like this:
public class MyClass {
public static int myStaticVariable = 0;
}
This way, myStaticVariable
is shared across all instances of the MyClass
class and can be accessed from anywhere within the class or even from other classes.
In summary:
You can define a static variable within a method in Java. The static variable will only be accessible within that method and won't be shared between different instances of the same class. To define a true class-level static variable, declare it outside any method.I hope this clarifies things for you!
Can we use static variable inside method in Java?
I'd be happy to help you with that!
Yes, it is possible to use static variables inside methods in Java. A static variable is a member of a class that belongs to the class itself rather than instances of the class. You can access and modify a static variable from within an instance method or even from another class.
Here's an example of how you can use a static variable inside a method:
public class MyClass {
public static int count = 0;
public void myMethod() {
System.out.println("Count: " + count);
count++;
}
public static void main(String[] args) {
MyClass obj1 = new MyClass();
MyClass obj2 = new MyClass();
obj1.myMethod(); // prints 0
obj2.myMethod(); // prints 0
// Now, both objects have their own copy of the static variable
System.out.println(obj1.count); // prints 1
System.out.println(obj2.count); // prints 1
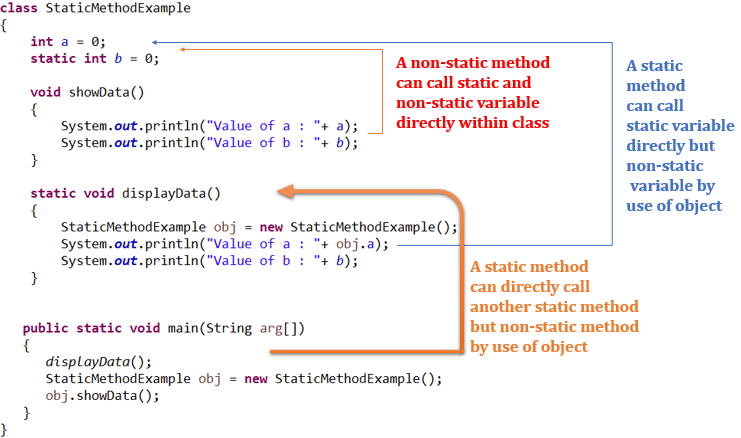
MyClass.staticMethod();
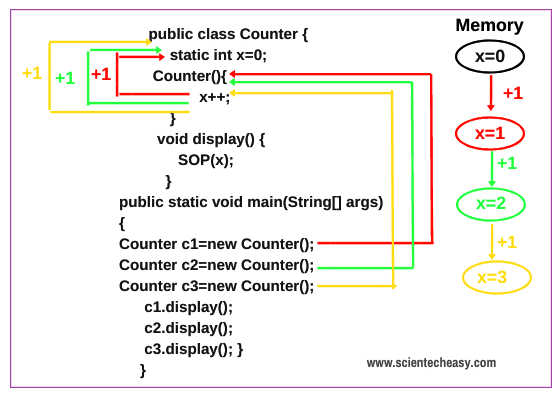
}
public static void staticMethod() {
count++;
}
}
In this example, the count
variable is declared as a static variable inside the MyClass
class. Both instance methods (myMethod
) and static methods can access and modify the static variable.
When you run the program, you'll see that both objects have their own copy of the count
variable. This is because each object has its own instance of the count
variable, but they're all referencing the same static variable.
Now, let's talk about some important points to consider when using static variables:
Thread-safety: Static variables are not inherently thread-safe. If multiple threads access a shared static variable simultaneously, it can lead to unexpected behavior. Visibility: Static variables are accessible from anywhere within the same class and its subclasses. Lack of encapsulation: Since static variables belong to the class itself, they're not encapsulated within any specific instance. This means that other classes or parts of your code might accidentally (or intentionally!) modify the static variable.When deciding whether to use a static variable, ask yourself:
Will this variable be used by multiple instances or only by one? Does this variable need to be shared across different parts of my application?If you answer "yes" to either question, using a static variable might not be the best choice. You should consider alternative approaches like using a singleton class, a utility class, or even a dedicated database.
Remember, static variables can be useful in specific situations, but they're not a panacea for all your Java problems. Use them wisely!
(Grandma's angry face: )