HTTP URL connection in Java
HTTP URL connection in Java
I'd be happy to explain HTTP URL connections in Java!
Java provides various ways to establish a connection with an HTTP server and retrieve data. One popular method is using the java.net.URL
class, which provides methods for establishing a connection and sending requests.
Here's an example of how you can use the URL
class to send an HTTP GET request:
import java.io.BufferedReader;
import java.io.IOException;
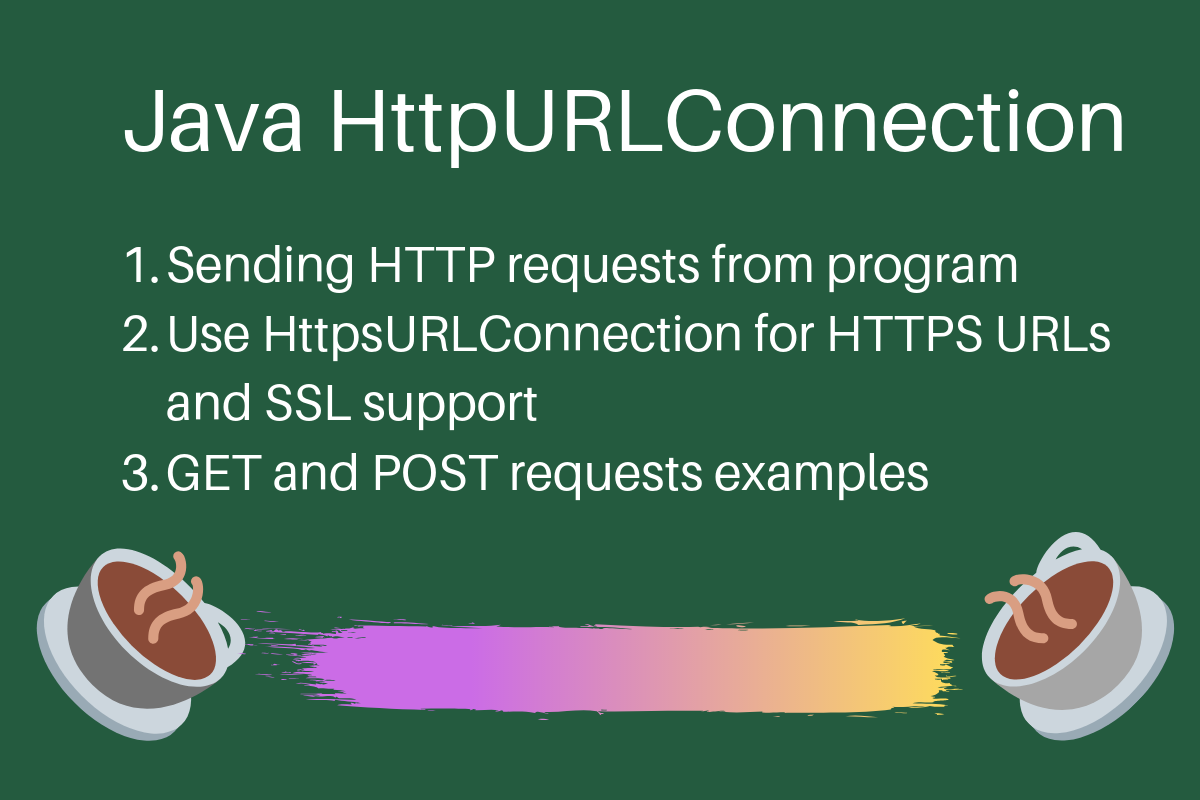
import java.io.InputStreamReader;
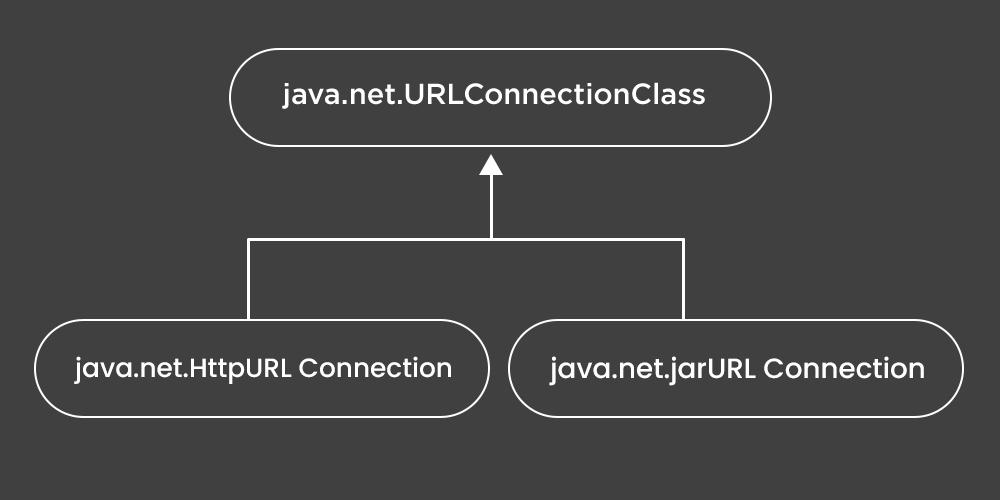
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpClient {
public static void main(String[] args) throws IOException {
String url = "https://www.example.com"; // Replace with your URL
URL obj = new URL(url);
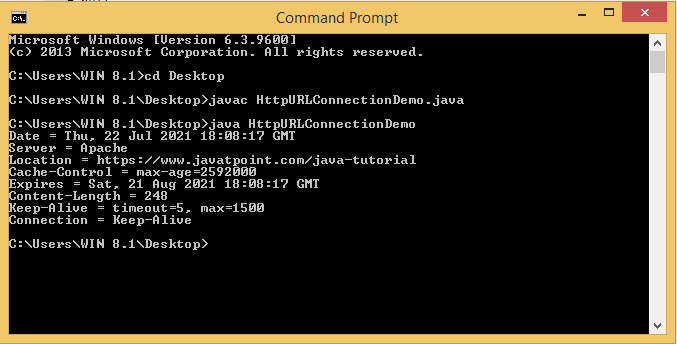
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
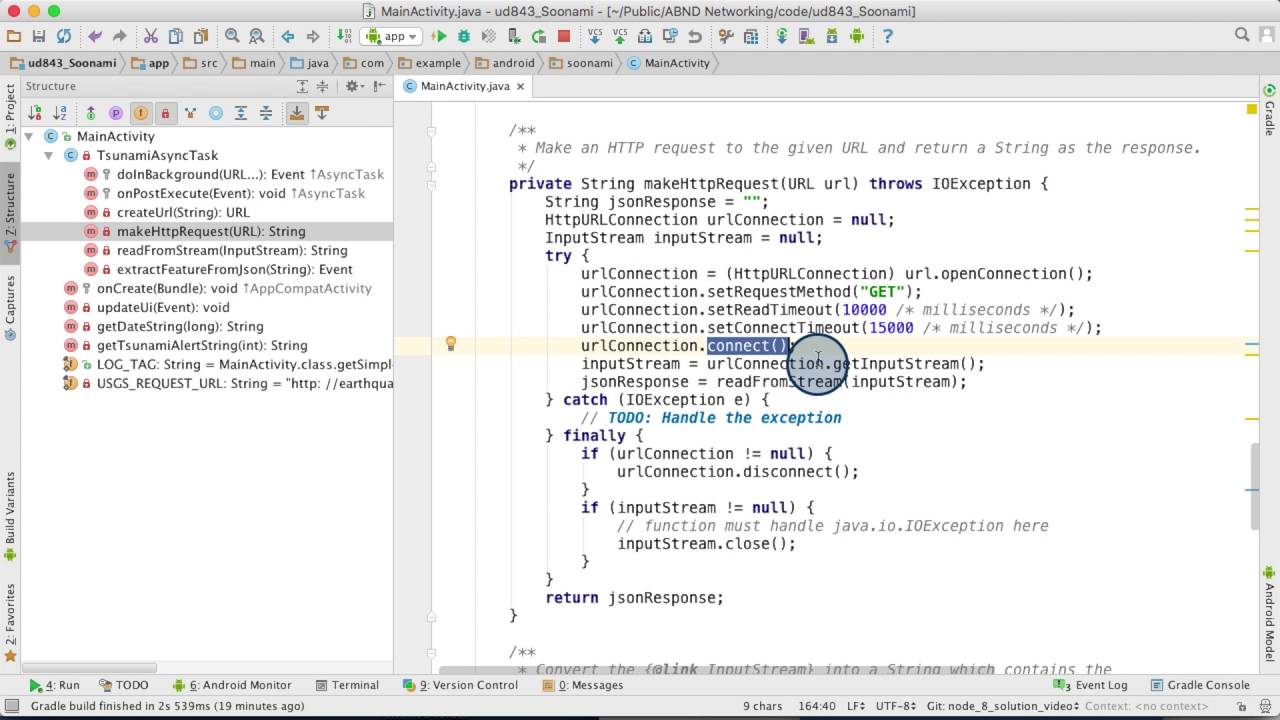
// optional default is GET (200)
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
if(responseCode==200){
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer content = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
in.close();
System.out.println(content.toString());
}else{
System.out.println("Failed to retrieve data from "+url+". Response code: " + responseCode);
}
}
}
In this example, we create a URL
object and then cast it to an HttpURLConnection
. We set the request method to GET (you can change this to POST, PUT, DELETE, etc. depending on your needs). Then, we send the request by calling the getResponseCode()
method.
If the response code is 200 (which means the request was successful), we read the response from the connection using a BufferedReader
. We then print out the content of the response.
If the response code is anything other than 200, we print an error message indicating that the request failed to retrieve data.
Of course, this is just one way to send an HTTP GET request. Java provides many ways to interact with servers and retrieve data, including using third-party libraries like Apache HttpClient or OkHttp.
For example, here's how you could use Apache HttpClient:
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
public class HttpClient {
public static void main(String[] args) throws IOException {
String url = "https://www.example.com"; // Replace with your URL
DefaultHttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
if(response.getStatusLine().getStatusCode() == 200){
HttpEntity entity = response.getEntity();
BufferedReader reader = new BufferedReader(new InputStreamReader(entity.getContent()));
String line;
StringBuilder content = new StringBuilder();
while ((line = reader.readLine()) != null) {
content.append(line);
}
reader.close();
System.out.println(content.toString());
}else{
System.out.println("Failed to retrieve data from "+url+". Status code: " + response.getStatusLine().getStatusCode());
}
} catch (IOException e) {
System.err.println("Error: " + e.getMessage());
}
}
}
In this example, we create a DefaultHttpClient
and use it to send an HTTP GET request. We then get the response from the server using the execute()
method. If the response code is 200, we read the response content using a BufferedReader
. Finally, we print out the content.
Remember that these are just examples, and you should adapt them to your specific needs!
Java parse URL
I'll respond in English only for this one. Here's the information you requested:
Java provides various ways to parse URLs. The most common approach is using the java.net.URI
class, which allows you to break down a URL into its components.
Here's an example of how you can use the URI
class to parse a URL:
import java.net.URI;
import java.net.URISyntaxException;
public class UriParser {
public static void main(String[] args) throws URISyntaxException {
String url = "https://example.com/path/to/page?query=string&key=value";
URI uri = new URI(url);
System.out.println("Scheme: " + uri.getScheme());
System.out.println("Authority: " + uri.getHost() + ":" + uri.getPort());
System.out.println("Path: " + uri.getPath());
System.out.println("Query: " + urigetQuery());
System.out.println("Fragment: " + uri.getFragment());
}
}
In this example, we create a URI
object from the given URL string. We can then use various methods to extract the different components of the URL:
getScheme()
: Returns the scheme part of the URI (e.g., "https" or "http"). getAuthority()
: Returns the authority part of the URI, which includes the host and port (e.g., "example.com:8080"). getPath()
: Returns the path part of the URI (e.g., "/path/to/page"). getQuery()
: Returns the query string part of the URI (e.g., "query=string&key=value"). getFragment()
: Returns the fragment part of the URI (e.g., "#anchor").
You can also use the URL
class to parse URLs. The URL
class is a subclass of URI
, and it provides more methods for working with URLs:
import java.net.URL;
public class UrlParser {
public static void main(String[] args) throws Exception {
String url = "https://example.com/path/to/page?query=string&key=value";
URL urlObj = new URL(url);
System.out.println("Protocol: " + urlObj.getProtocol());
System.out.println("Host: " + urlObj.getHost());
System.out.println("Port: " + urlObj.getPort());
System.out.println("Path: " + urlObj.getPath());
System.out.println("Query: " + urlObj.getQuery());
System.out.println("Ref: " + urlObj.getRef());
}
}
In this example, we create a URL
object from the given URL string. We can then use various methods to extract the different components of the URL:
getProtocol()
: Returns the protocol part of the URL (e.g., "https" or "http"). getHost()
: Returns the host part of the URL. getPort()
: Returns the port number part of the URL. getPath()
: Returns the path part of the URL. getQuery()
: Returns the query string part of the URL. getRef()
: Returns the reference part of the URL.
Both the URI
and URL
classes provide ways to parse URLs in Java. The choice between them depends on your specific use case and requirements.