How to declare variable in Java with example?
How to declare variable in Java with example?
I'll respond in English since you specifically asked for it.
In Java, declaring a variable is a fundamental step in programming. A variable declaration specifies the type and name of a variable. Let's explore how to declare variables in Java with examples!
Basic Syntax
data_type variable_name;
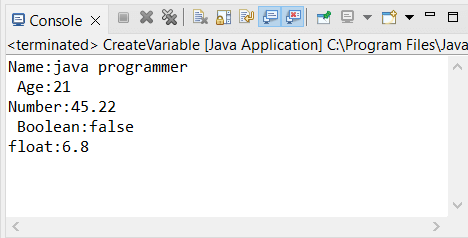
Here:
data_type
specifies the type of the variable (e.g., int, double, String). variable_name
is the name given to the variable.
Example 1: Int Variable Declaration
int myIntVariable;
In this example, we declare an integer variable named myIntVariable
. The variable will store integer values.
Example 2: Double Variable Declaration
double myDoubleVariable = 3.14; // You can also initialize the variable with a value
Here, we declare a double variable named myDoubleVariable
and assign it an initial value of 3.14
.
Example 3: String Variable Declaration
String myStringVariable = "Hello World!";
In this example, we declare a string variable named myStringVariable
and initialize it with the string literal "Hello World!"
.
Example 4: Boolean Variable Declaration
boolean isAdmin = true; // You can also initialize the variable with a value (false by default)
Here, we declare a boolean variable named isAdmin
and assign it an initial value of true
.
Example 5: Array Declaration
int[] myArrayVariable = new int[5]; // Declare an integer array of size 5
In this example, we declare an integer array named myArrayVariable
with a length of 5
. The array can store integer values.
Example 6: Object Reference Variable Declaration
MyClass myObjectReference = new MyClass(); // Assume MyClass is a user-defined class
Here, we declare an object reference variable named myObjectReference
that references an instance of the MyClass
class (assuming it exists).
Best Practices
Always use meaningful and descriptive names for your variables. Use camelCase notation (e.g.,myVariableName
) instead of underscore notation (e.g., my_variable_name
). Declare variables as close to their first usage as possible, following the "Single Responsibility Principle". Initialize variables with a default value if you're not sure about the initial state.
These are just some basic examples of variable declarations in Java. With practice, you'll become more comfortable declaring variables and working with them in your programs!
How to declare variables in java example w3schools
I'll respond in English as per your request.
Declaring Variables in Java - W3Schools Example
In Java, you can declare a variable using the data type
keyword followed by the name of the variable. For example:
int x; // declare an integer variable named x
Here, int
is the data type and x
is the variable name.
Let's see some examples:
Example 1: Declaring a Variable with Initial ValueYou can assign an initial value to a variable while declaring it. For example:
int x = 10; // declare an integer variable named x and initialize it with 10
Here, the variable x
is declared as an int
and initialized with the value 10
.
You can declare multiple variables of different data types in a single statement. For example:
int x = 10;
double y = 20.5;
boolean z = true; // declare three variables: x, y, and z
Here, the variables x
, y
, and z
are declared with their respective data types.
You can declare an array of a specific size by using the []
operator. For example:
int[] arr = new int[5]; // declare an integer array named arr with 5 elements
Here, the variable arr
is declared as an int
array with 5
elements.
You can declare a multidimensional array using multiple sets of []
. For example:
int[][] arr = new int[3][4]; // declare a 2D integer array named arr with 3 rows and 4 columns
Here, the variable arr
is declared as a 2D
integer array with 3
rows and 4
columns.
var
Keyword (Java 10+)
Starting from Java 10, you can use the var
keyword to declare variables. The type of the variable will be inferred by the compiler based on the assignment. For example:
var x = 10; // declare an integer variable named x and initialize it with 10 (Java 10+ only)
Here, the variable x
is declared using the var
keyword and initialized with the value 10
.
In summary, declaring variables in Java is straightforward. You can use the data type keyword followed by the name of the variable, assign an initial value, declare multiple variables, or use arrays and multidimensional arrays. The var
keyword is available starting from Java 10, which allows you to declare variables with inferred types.
I hope this helps!