How can arrays be used in Java?
How can arrays be used in Java?
I'm happy to respond in English!
Arrays are a fundamental data structure in Java, and they play a crucial role in storing and manipulating collections of values. In this response, I'll cover the basics of arrays in Java, their usage, and some best practices.
What is an Array?
In Java, an array is a fixed-size, homogeneous collection of elements of the same data type. It's essentially a container that stores values of the same type, such as integers, strings, or objects. You can think of an array like a box where you can store multiple values of the same type.
Declaring and Initializing Arrays
To declare an array in Java, you need to specify its data type and size. For example:
int[] scores = new int[5];
This declares an integer array called scores
with a size of 5 elements. The new
keyword is used to create the array.
You can also initialize arrays during declaration using the following syntax:
String[] colors = {"Red", "Green", "Blue"};
This declares a string array called colors
and initializes it with three values: "Red", "Green", and "Blue".
Accessing Array Elements
Arrays use zero-based indexing, which means that the first element is at index 0. You can access an array element using its index:
int score = scores[0]; // accesses the first element (index 0)
You can also iterate through an array using a for
loop:
for (int i = 0; i < scores.length; i++) {
System.out.println("Score: " + scores[i]);
}
Multidimensional Arrays
Java supports multidimensional arrays, which are arrays of arrays. You can declare a multidimensional array as follows:
int[][] matrix = new int[2][3];
This declares a 2x3 integer array called matrix
. Each row is an array of integers.
Array Methods
Arrays in Java provide several useful methods, including:
length
: Returns the number of elements in the array. get()
: Retrieves an element at a specified index. set()
: Sets an element at a specified index. clone()
: Creates a copy of the entire array.
Best Practices
Here are some best practices to keep in mind when working with arrays in Java:
Use meaningful variable names: Choose descriptive names for your arrays and variables to make your code easy to read. Initialize arrays correctly: Make sure you initialize arrays properly, either during declaration or using thenew
keyword. Use indexing correctly: Remember that arrays use zero-based indexing, so be careful when accessing array elements. Avoid using magic numbers: Instead of hardcoding indices or sizes, define constants to make your code more readable and maintainable.
In conclusion, arrays are a fundamental data structure in Java, providing a flexible way to store and manipulate collections of values. By understanding how to declare, initialize, access, and use array methods effectively, you can write efficient and readable code that takes advantage of arrays' features.
How to initialize ArrayList in Java with default values
Initializing an ArrayList
in Java can be done in various ways, depending on the requirements and needs of your program. In this response, we'll explore different methods for initializing an ArrayList
with default values.
Method 1: Using a Constructor
You can create an ArrayList
using its constructor, specifying the initial capacity and the type of elements it will hold. Here's how to do it:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an ArrayList with default values (null in this case)
List arrayList = new ArrayList<>(10);
// Now, you can add elements to the list as needed
arrayList.add("Hello");
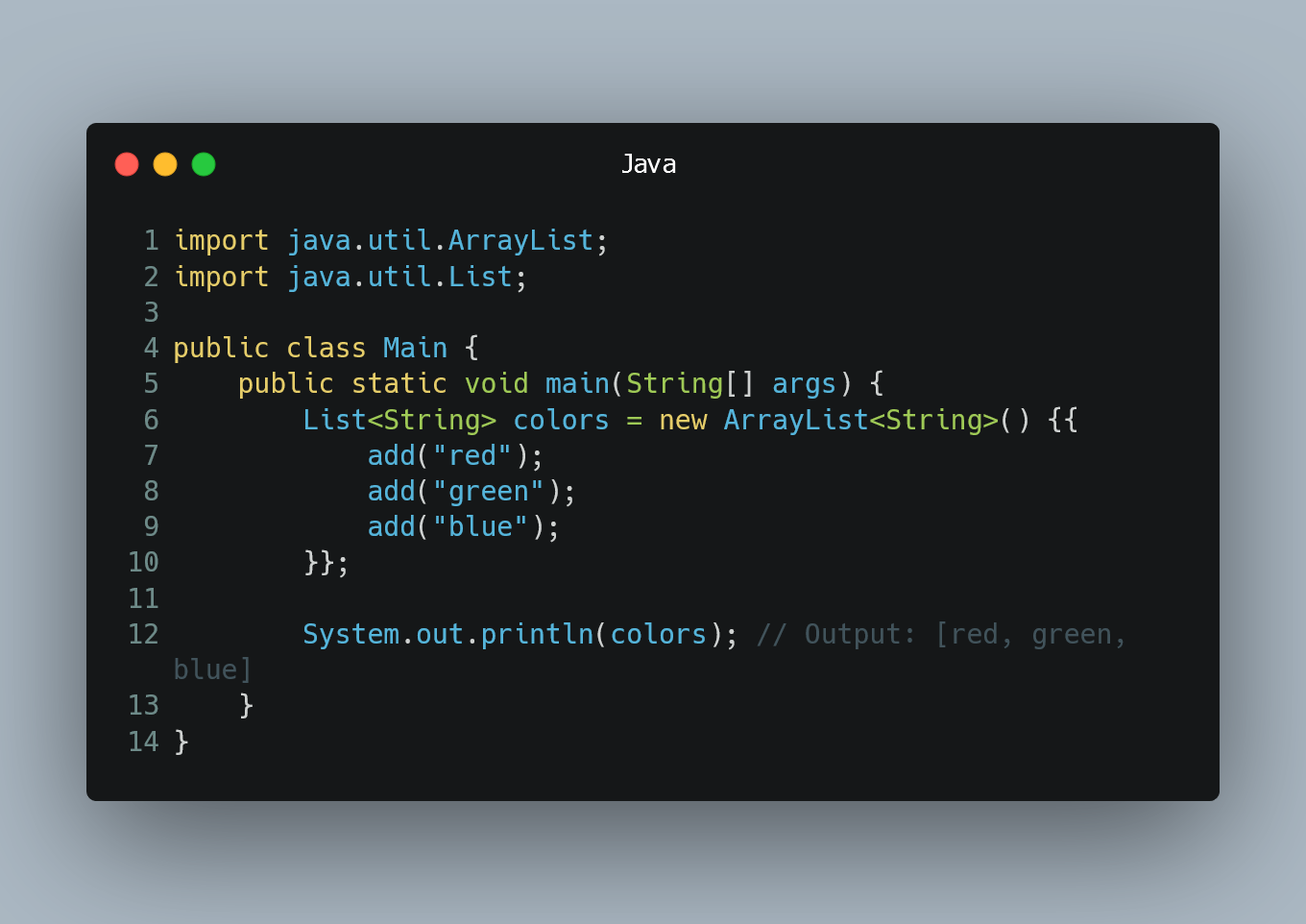
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
In this example, we're creating an ArrayList
that will hold String
objects. We specify an initial capacity of 10, which means the list can initially store up to 10 elements without needing to be resized. The default values for the list are null, meaning all elements will start out as null references.
Method 2: Using a Generic Constructor
If you know the type of elements your ArrayList
will hold ahead of time, you can specify it using a generic constructor:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an ArrayList with default values (null in this case)
List arrayList = new ArrayList<>();
// Now, you can add elements to the list as needed
arrayList.add("Hello");
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
This constructor is equivalent to calling new ArrayList<>();
or new ArrayList<String>();
, which initializes an empty list with no initial values.
Method 3: Using a Static Method
The Arrays.asList()
method can be used to initialize an ArrayList
from a collection:
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// Create an ArrayList with default values (null in this case)
List arrayList = new ArrayList<>(Arrays.asList());
// Now, you can add elements to the list as needed
arrayList.add("Hello");
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
This method creates an empty list and then initializes it with default values.
Method 4: Using a Loop
You can also initialize an ArrayList
by using a loop to add elements:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an ArrayList and add default values (null in this case)
List arrayList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
arrayList.add(null);
}
// Now, you can add elements to the list as needed
arrayList.add("Hello");
arrayList.add(null);
arrayList.add("World");
System.out.println(arrayList);
}
}
This method creates an empty list and then adds null values to it using a loop.
In conclusion, initializing an ArrayList
in Java can be done in various ways, depending on your specific needs. The methods outlined above provide different approaches for initializing an ArrayList
with default values, allowing you to create lists that are tailored to your programming requirements.