Java create String array with values
Java create String array with values
Here is an example of creating a string array with values using Java:
public class Main {
public static void main(String[] args) {
// Declare and initialize a string array
String[] myArray = new String[5];
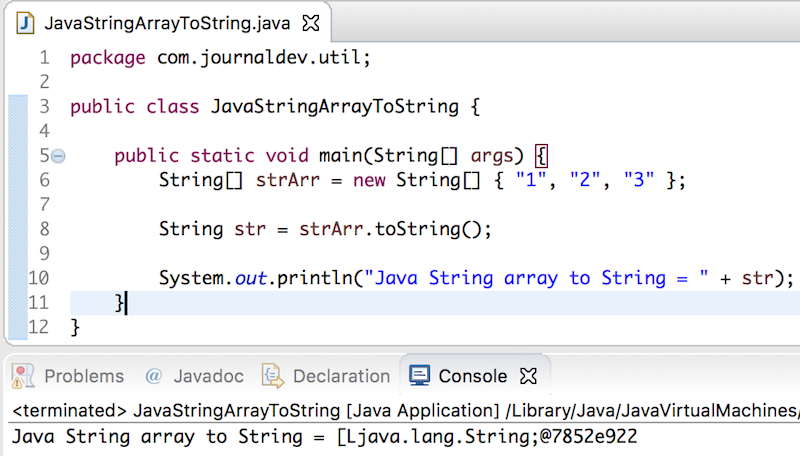
// Assign values to the array elements
myArray[0] = "Hello";
myArray[1] = "World";
myArray[2] = "Java";
myArray[3] = "Programming";
myArray[4] = "Language";
// Print the array elements
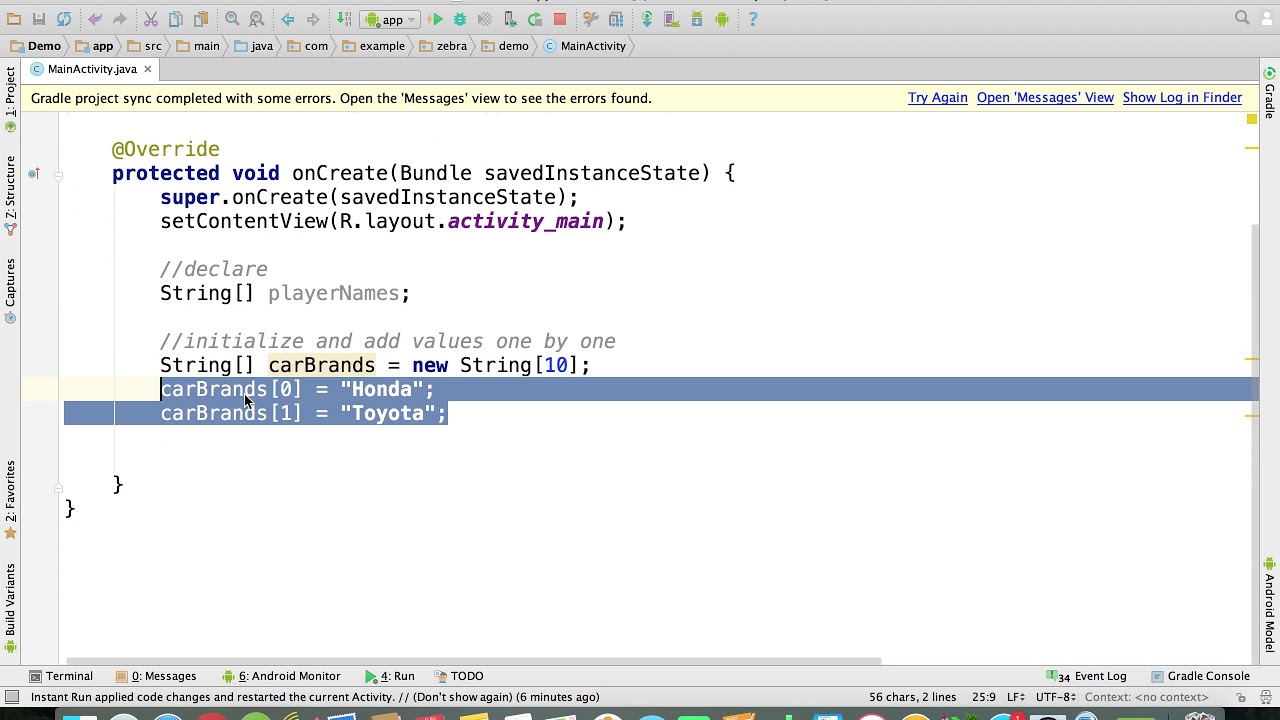
for (int i = 0; i < myArray.length; i++) {
System.out.println("Element at index " + i + ": " + myArray[i]);
}
}
}
When you run this program, it will output:
Element at index 0: Hello
Element at index 1: World
Element at index 2: Java
Element at index 3: Programming
Element at index 4: Language
As you can see, we declared a string array myArray
with a length of 5. Then we assigned five different strings to the array elements using the syntax myArray[index] = "stringValue";
. Finally, we used a for loop to print out each element in the array.
Here are some key points about creating and using string arrays in Java:
Declare the array: You need to declare the string array before you can use it. The declaration statement should include the name of the array, the type of data it will hold (in this case, strings), and its length. Initialize the array: You don't necessarily need to initialize the array when you declare it, but you do need to make sure each element has a value assigned to it before you can use it. In Java, you can assign a null value to an element, which means that the element does not currently hold any useful data. Access elements: You access elements in the array by using their index. The first element is at index 0, the second element is at index 1, and so on. Use loops to process elements: Because arrays are a type of collection, you can use various types of loops (such as for, while, or foreach) to process each element in the array. Convert between string arrays and other data structures: You can easily convert a string array to other data structures like LinkedList, ArrayList, HashSet, etc.Here's an example of how you could convert a string array to a LinkedList:
public class Main {
public static void main(String[] args) {
// Declare and initialize a string array
String[] myArray = new String[5];
// Assign values to the array elements
myArray[0] = "Hello";
myArray[1] = "World";
myArray[2] = "Java";
myArray[3] = "Programming";
myArray[4] = "Language";
// Convert the array to a LinkedList
LinkedList linkedList = new LinkedList<>();
for (String s : myArray) {
linkedList.add(s);
}
// Print out each element in the LinkedList
for (String s : linkedList) {
System.out.println("Element: " + s);
}
}
}
When you run this program, it will output:
Element: Hello
Element: World
Element: Java
Element: Programming
Element: Language
Can you declare an array without a size in Java?
I'd be happy to help!
In Java, you can't directly declare an array without specifying its size. This is because arrays are dynamically allocated on the heap when they're created, and Java needs to know how much space it should reserve for the elements in the array.
However, there's a way around this: you can use an ArrayList (or another implementation of List interface) from the java.util package, which allows dynamic resizing. This means that you don't need to specify the size when creating the list, and you can add or remove elements as needed without worrying about running out of space.
Here's an example:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List myArrayList = new ArrayList<>();
// Add elements to the list
myArrayList.add("Hello");
myArrayList.add("World");
myArrayList.add("Java");
System.out.println(myArrayList.size()); // prints: 3
// Remove an element from the list
myArrayList.remove(1);
System.out.println(myArrayList.size()); // prints: 2
// Add another element to the list
myArrayList.add("Programming");
System.out.println(myArrayList.size()); // prints: 3 (again!)
}
}
As you can see, ArrayList is a very flexible data structure that allows for dynamic resizing and manipulation of elements. You don't need to know the exact size when creating it, which makes it suitable for many use cases.
Now, if you really want to have an array-like structure without specifying the size upfront, there's another way: you can use a LinkedList from the java.util package. This allows you to add and remove elements dynamically, similar to ArrayList. However, LinkedList is optimized for inserting or removing elements at specific positions in the list, which makes it useful for certain scenarios.
Here's an example:
import java.util.LinkedList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List myLinkedList = new LinkedList<>();
// Add elements to the list
myLinkedList.add("Hello");
myLinkedList.add("World");
myLinkedList.add("Java");
System.out.println(myLinkedList.size()); // prints: 3
// Remove an element from the list
myLinkedList.remove(1);
System.out.println(myLinkedList.size()); // prints: 2
// Add another element to the list
myLinkedList.add("Programming");
System.out.println(myLinkedList.size()); // prints: 3 (again!)
}
}
So, while you can't declare an array without a size in Java, there are alternative data structures like ArrayList and LinkedList that offer similar functionality with dynamic resizing.