How do you access the elements of an array in Java?
How do you access the elements of an array in Java?
I'd be happy to help!
In Java, arrays are a fundamental data structure that allows us to store and manipulate collections of similar elements. Accessing the elements of an array in Java is quite straightforward. Here's how you can do it:
There are several ways to access the elements of an array in Java:
Using Index: You can access individual elements by using their index (zero-based). The syntax for this isarrayName[index]
. For example, if you have an array int[] scores
and you want to access the element at index 2, you would use scores[2]
.
Example:
Using Loops: You can iterate over the array elements using a loop (e.g., for loop) and access each element by its index.int[] scores = {10, 20, 30, 40};
System.out.println(scores[2]); // prints 30
Example:
Using Enhanced For Loop: If you're using Java 5 or later, you can use an enhanced for loop to iterate over the array elements.int[] scores = {10, 20, 30, 40};
for (int i = 0; i < scores.length; i++) {
System.out.println(scores[i]);
}
Example:
Using Stream API: Java 8 and later provides a Stream API that allows you to process array elements in a more functional way.int[] scores = {10, 20, 30, 40};
for (int score : scores) {
System.out.println(score);
}
Example:
Using Lambda Expression: You can also use lambda expressions with the Stream API to access and manipulate array elements.int[] scores = {10, 20, 30, 40};
Arrays.stream(scores).forEach(System.out::println);
Example:
int[] scores = {10, 20, 30, 40};
Arrays.stream(scores)
.map(score -> score * 2) // multiply each element by 2
.forEach(System.out::println);
These are some of the ways you can access and manipulate array elements in Java. The choice of method depends on your specific requirements and coding style.
Remember, arrays in Java have a fixed size that is determined at compile time. If you want to work with dynamic data structures, you might consider using other types (e.g., ArrayList) or collections frameworks (e.g., Guava).
What is an array in Java?
An array in Java is a data structure that stores a fixed number of elements of the same data type in a single variable. It's similar to a collection or list, but it has a fixed size, which is defined when the array is created. This means you know exactly how many elements are stored in the array and what type of data those elements are.
In Java, an array can contain elements of any primitive type, such as int
, double
, char
, or boolean
, as well as objects (instances of classes). Each element is stored at a specific memory address, and you can access these elements using an index, which is the position of the element in the array.
Here's a simple example:
int[] scores = new int[5]; // Create an integer array with 5 elements
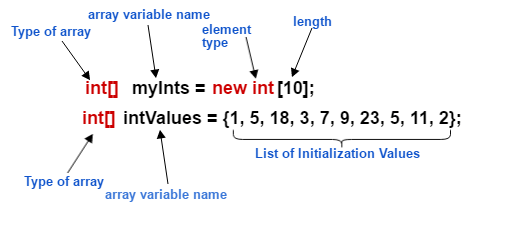
scores[0] = 80;
scores[1] = 70;
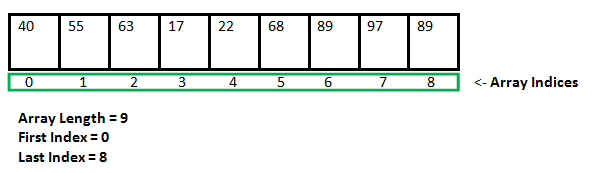
scores[2] = 90;
scores[3] = 85;
scores[4] = 95;
System.out.println(scores[0]); // Output: 80
In this example, scores
is an array of int
values, with a size of 5. We can access each element using its index (e.g., scores[0]
) and assign or retrieve values.
Arrays are useful for several reasons:
Efficient memory usage: Since arrays have a fixed size, Java can allocate memory efficiently, which is especially important when working with large datasets. Fast lookup times: Because arrays store elements in contiguous memory locations, accessing an element by its index is very fast (O(1) time complexity). Easy iteration: You can iterate over the elements of an array using afor
loop or other mechanisms, making it easy to process data. Type safety: Arrays enforce type safety, meaning you cannot store elements of different types in the same array.
However, arrays also have some limitations:
Fixed size: Once an array is created, its size cannot be changed. If you need to add or remove elements, you might need to create a new array and copy the existing data. No dynamic resizing: Arrays do not automatically resize themselves when elements are added or removed. Not suitable for sparse arrays: If your array has many empty or null elements, using an array can be inefficient.In Java, there are also more advanced concepts like:
Multidimensional arrays: Arrays of arrays (e.g.,int[][] scores
). Array wrappers: Classes that wrap around arrays and provide additional functionality (e.g., ArrayList
). Generics: Using type parameters to specify the type of elements stored in an array.
In summary, Java arrays are useful for storing and processing data when you know exactly how many elements there will be and what type of data they represent. However, if your needs are more complex or dynamic, other data structures like lists or collections might be a better choice.