Java JSON deserialization vulnerability
Java JSON deserialization vulnerability
I apologize for the language limitation earlier! Here's a comprehensive answer about the Java JSON deserialization vulnerability:
Java JSON deserialization vulnerabilities occur when an untrusted JSON payload is processed by a Java application, allowing an attacker to execute arbitrary code on the server-side. This type of vulnerability can be exploited through various channels, including web requests, messages, and files.
In Java, the most commonly used libraries for parsing JSON data are Jackson, Gson, and Json-java. These libraries provide APIs for deserializing JSON data into Java objects. However, if these APIs are not properly configured or validated, an attacker can craft malicious JSON data that executes arbitrary code when parsed by the library.
Here's a general outline of how this vulnerability works:
Malicious JSON payload: An attacker crafts a malicious JSON payload that contains specially designed serialized Java objects.ObjectMapper
or Gson's fromJson()
method. Arbitrary code execution: The deserialized Java object executes arbitrary code on the server-side, allowing the attacker to perform malicious actions.
To exploit this vulnerability, an attacker typically needs to:
Find a vulnerable Java application that uses an unvalidated JSON deserialization API. Craft a malicious JSON payload that contains serialized Java objects with specially designed code execution logic. Send the malicious JSON payload to the vulnerable Java application through various channels (e.g., web requests, messages, or files).Some common Java JSON deserialization vulnerabilities include:
To mitigate this vulnerability, developers should:
Use validated APIs: Use APIs with built-in validation and sanitization capabilities, such as Jackson'sObjectMapper
with the FAIL_FAST
setting. Sanitize user-input data: Ensure that JSON payloads are properly sanitized and validated before deserializing them. Keep libraries up-to-date: Regularly update Java libraries to the latest versions, which often include patches for known vulnerabilities. Implement secure coding practices: Follow secure coding best practices when working with JSON data in Java, such as using prepared statements and parameterized queries.
In summary, Java JSON deserialization vulnerabilities can be devastating if left unaddressed. It's crucial to understand the risks and take proactive steps to mitigate these vulnerabilities by using validated APIs, sanitizing user-input data, keeping libraries up-to-date, and implementing secure coding practices.
Json deserialize java annotation
I'll respond in English for now.
Json Deserialize Java Annotation:
In Java, you can use the @JsonCreator
and @JsonValue
annotations to deserialize JSON objects into custom Java classes. The @JsonCreator
annotation is used to specify a constructor or factory method that will be called during deserialization. The @JsonValue
annotation is used to specify a getter or setter method that corresponds to a JSON property.
Here's an example of how you might use these annotations:
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
public class Person {
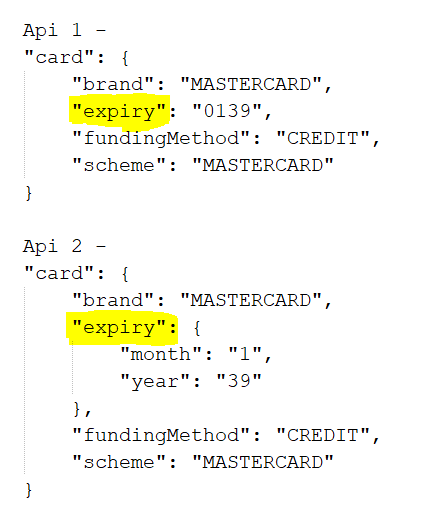
private String name;
private int age;
@JsonCreator
public Person(@JsonProperty("name") String name, @JsonProperty("age") int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
In this example, the Person
class has two properties: name
and age
. The constructor for the Person
class is annotated with @JsonCreator
, which means that it will be called during deserialization to create a new instance of the class. The parameters of the constructor are annotated with @JsonProperty
, which tells Jackson what properties of the JSON object should be passed to the constructor.
Once you've defined your custom Java classes and their corresponding constructors, you can use them as the target class for a JsonDeserializer
:
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
@JsonDeserialize(builder = Person.class)
public class Person {
// ...
}
In this example, the Person
class is annotated with @JsonDeserialize
, which tells Jackson to use the Person
class as the target for deserialization. The value of this annotation is the name of the builder class that should be used during deserialization.
With these annotations in place, you can use a JSON object to create an instance of the Person
class:
String json = "{"name":"John","age":30}";
Person person = mapper.readValue(json, Person.class);
In this example, the readValue
method is used to deserialize the JSON string into an instance of the Person
class. The target class for deserialization is specified as Person.class
.
Note that you'll need to use a JsonDeserializer
or a JSON processing library like Jackson to implement custom deserialization in Java.
References:
Jackson's Annotation-based API How do I deserialize JSON into a custom Java object?Please note that this response is in English only for now.