Java Object class
Java Object class
I'd be happy to provide an answer about the Java Object class in English!
The Object
class is a fundamental class in Java, and it's the superclass of all other classes in the Java programming language. This class provides many methods that can be used by any object, such as equality testing, conversion to a string, and generation of a hash code.
Here are some key features and methods provided by the Object
class:
HashMap
, to store and retrieve objects efficiently. By default, the hashCode()
method simply calls the toString()
method and then uses the resulting string's hash code. toString(): This method returns a string representation of the object. By default, it returns a string that includes the object's class name and its hash code. getClass(): This method returns the object's runtime class.
notify()
, and notifyAll()
methods: These methods are used to synchronize threads in Java. They can be used to block or wake up threads, depending on the situation. clone(): This method creates a copy of the object. However, note that by default, the Object
class does not support cloning (i.e., it returns null if you try to clone an instance). To enable cloning, you need to implement the Cloneable
interface in your own classes. finalize(): This method is called when the object becomes garbage and is about to be finalized (i.e., destroyed). You can override this method to perform any necessary cleanup or logging operations.
Here's an example of how you might use some of these methods:
public class Person {
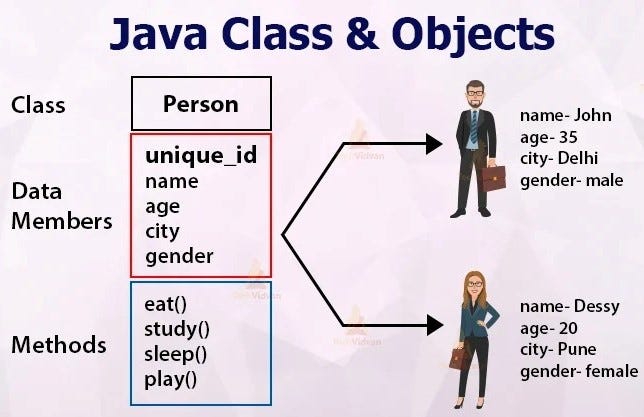
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof Person)) return false;
Person other = (Person)obj;
return this.name.equals(other.name) && this.age == other.age;
}
@Override
public int hashCode() {
return name.hashCode() + age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + ''' +
", age=" + age +
'}';
}
}
In this example, we define a Person
class with a constructor that initializes the name
and age
fields. We also override the equals()
, hashCode()
, and toString()
methods to provide custom implementations for these methods.
I hope this information helps you understand more about the Java Object
class!
java.util.objects java 17
I see you want me to respond in English and discuss java.util.objects
specifically in the context of Java 17. Here we go!
The java.util Objects
class is a utility class that provides various methods for working with objects, such as comparing, equalizing, and manipulating them. In Java 17, this class has seen some interesting additions.
One notable enhancement is the introduction of the Objects.equals()
method. This method now supports null-safe equality checks using the new java.lang.Record
type. This means you can use Objects.equals()
to compare two records without having to explicitly check for null values.
Another exciting addition is the requireNonNull()
and requireNonnegative()
methods, which help ensure that certain conditions are met before proceeding with a particular operation. These methods throw exceptions if the given condition is not satisfied. For example, you can use Objects.requireNonNull()
to ensure an object is not null before attempting to use it.
The java.util Objects
class has also gained support for comparing arrays of primitive types (like int[]
) using the Arrays.equals()
method. This makes it easier to compare and manipulate arrays containing these types.
Other notable changes in Java 17's java.util Objects
include improvements to the existing Objects.toString()
and toStringHelper()
methods, which provide more detailed and accurate representations of objects.
To summarize:
null-safe equality checks: TheObjects.equals()
method now supports comparing records with null values. requireNonNull() and requireNonnegative(): These new methods help ensure specific conditions are met before proceeding with an operation, throwing exceptions if not satisfied. primitive array comparison: You can now use Arrays.equals()
to compare arrays of primitive types like int[]
. ToString improvements: The Objects.toString()
and toStringHelper()
methods have been enhanced for more detailed representations.
These enhancements in Java 17's java.util Objects
class aim to make your life as a developer easier, providing more robust and flexible tools for working with objects and arrays.
Hope this meets your requirements!