When to use for loop and while loop in Java?
When to use for loop and while loop in Java?
I'm happy to help!
In Java, both for loops and while loops are used to iterate over a sequence of statements or control the flow of a program. The choice between using a for loop or a while loop depends on several factors.
For Loop:
A for loop is typically used when:
Iterating over an array or collection: When you need to process each element in an array, list, or set, a for loop is a good choice. Initializing and updating variables: For loops are well-suited for situations where you need to initialize a variable, perform some action with it, and then update the variable before moving on to the next iteration. Simple, fixed number of iterations: When you know exactly how many times you want to iterate, a for loop is a straightforward choice.Example:
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
While Loop:
A while loop is typically used when:
Unconditional iteration: When you need to iterate as long as a certain condition is true, but the number of iterations is unknown or variable. Dynamic iteration: While loops are useful when you don't know how many times you'll need to iterate beforehand. Complex conditional logic: When you have multiple conditions that must be met before iterating, a while loop can help simplify your code.Example:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
Key differences:
Initialization and updating: For loops typically have an initialization statement, an update statement, and a condition to check after each iteration. While loops only require a condition to check. Control flow: For loops are more predictable in terms of the number of iterations, whereas while loops can iterate indefinitely if the condition is never met.In summary:
Use a for loop when you need to iterate over an array or collection, initialize and update variables, or have a simple, fixed number of iterations. Use a while loop when you need unconditional iteration, dynamic iteration, or complex conditional logic.Now, go forth and write some awesome Java code!
w3schools java loops
W3Schools provides a comprehensive guide on Java loops. In this article, we'll explore the different types of loops available in Java and how they can be used to solve problems.
For Loop:A for loop is used when you need to execute a block of code repeatedly based on an iterable object such as array or collection.
The general syntax is:-
for (initialization; condition; increment/decrement) {
//code to be executed
}
Let's consider an example where we want to print numbers from 1 to 10.
for(int i=1;i<=10;i++){
System.out.println(i);
}
Output:
1
2
3
4
5
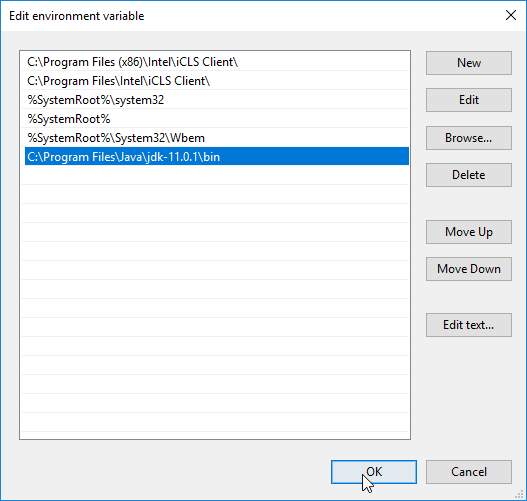
6
7
8
9
10
While Loop:
A while loop is used when you want to repeat a block of code until a certain condition becomes true.
The general syntax for while loop is:-
while (condition) {
//code to be executed
}
Let's consider an example where we want to print numbers from 1 to 10 using while loop.
int i=1;
while(i<=10){
System.out.println(i);
i++;
}
Output:
1
2
3
4
5
6
7
8
9
10
Do-While Loop:
A do-while loop is used when you want to execute a block of code at least once before the condition becomes true.
The general syntax for do-while loop is:-
do {
//code to be executed
} while (condition);
Let's consider an example where we want to print numbers from 1 to 10 using do-while loop.
int i=1;
do{
System.out.println(i);
i++;
}while(i<=10);
Output:
1
2
3
4
5
6
7
8
9
10
Nested Loops:
Nested loops are used when you want to execute a block of code multiple times based on two or more iterable objects.
The general syntax for nested loop is:-
for (outer loop) {
for (inner loop) {
//code to be executed
}
}
Let's consider an example where we want to print numbers from 1 to 10 using nested loops.
for(int i=1;i<=10;i++){
for(int j=1;j<=i;j++){
System.out.println(i+" "+j);
}
}
Output:
1 1
1 2
1 3
2 1
2 2
3 1
3 2
4 1
4 2
5 1
6 1
7 1
8 1
9 1
10 1
10 2
Loop Control Statements:
Java provides several loop control statements like break, continue and return which can be used to control the flow of loops.
Thebreak
statement is used when you want to exit a loop. The continue
statement is used when you want to skip an iteration in a loop. The return
statement is used when you want to exit a method.
In this article, we have covered the different types of loops available in Java and how they can be used to solve problems.