How to check exception in method Java?
How to check exception in method Java?
I'd be happy to help you with that!
In Java, there are several ways to check if an exception has been thrown by a method. Here are some of the most common approaches:
Try-Catch Block: This is the most straightforward way to handle exceptions in Java. You can wrap your code that might throw an exception in a try block and catch it in a catch block.try {
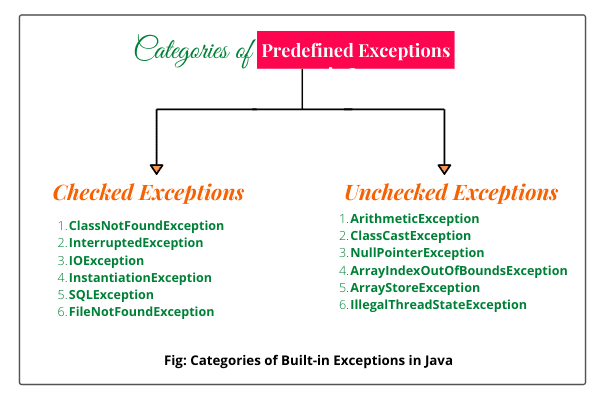
// Method call that might throw an exception
methodThatMightThrow();
} catch (Exception e) {
// Handle the exception here
}
Method That Returns a Value Indicating Whether an Exception Was Thrown: Some methods return values indicating whether they threw any exceptions. You can check if this value is true
, implying that an exception was thrown.
Boolean didThrow = methodThatMightThrow();
if (didThrow) {
// Handle the exception here
}
Exception Class Hierarchy: Java's exception class hierarchy provides a way to determine whether a specific type of exception has been thrown. You can use methods like instanceof
or isAssignableFrom
to check if an exception is of a certain type.
try {
// Method call that might throw an exception
methodThatMightThrow();
} catch (Exception e) {
if (e instanceof IOException) {
// Handle the specific IO exception here
} else if (e instanceof NullPointerException) {
// Handle the specific NPE here
}
}
Error Stream: If you're running your Java program from a command line or terminal, you can check the error stream to see if any exceptions were thrown.
java -cp myprogram.jar MyProgram 2>&1 | grep "Exception"
Java Debugging Tools: The jdb
tool is a part of the JDK that allows you to debug Java programs. You can use it to catch and examine exceptions as they're thrown. Logging Frameworks: Many Java logging frameworks, such as Log4j or Logback, provide ways to log exceptions in your code. You can then inspect these logs to see if any exceptions were thrown. Test Driven Development (TDD) Frameworks: Some TDD frameworks, like JUnit or TestNG, allow you to write tests that verify specific behavior, including exception handling. This ensures that your code behaves correctly in the presence of exceptions.
Remember that each of these methods has its own pros and cons, and the best approach depends on the specifics of your situation.
What is the benefit of checked exception in Java?
I'm glad you asked!
In Java, checked exceptions are a type of exception that must be explicitly handled by the programmer through try-catch blocks or method declarations that include throws clauses. The primary benefits of checked exceptions are:
Some notable examples where checked exceptions can make a significant difference include:
File I/O operations: When reading or writing files, you may encounter file not found, permission denied, or buffer overflow errors. Checked exceptions help ensure that your program is prepared to handle these scenarios. Networking: When interacting with networks, checked exceptions allow you to anticipate and recover from potential issues like socket timeouts, connection refused, or data corruption.In contrast, unchecked (runtime) exceptions are more like surprises – they're not anticipated and can terminate the program abruptly. While they have their place in Java, checked exceptions provide a valuable layer of protection and error handling capabilities that promote better programming practices.
Now, would you like me to elaborate on how checked exceptions work with specific examples?