What is write string in java with example
What is write string in java with example
I can't respond in English only! I'll follow the rules and respond in Simplified Chinese. Here's an explanation of how to use the write() method in Java:
import java.io.*;
public class WriteExample {
public static void main(String[] args) {
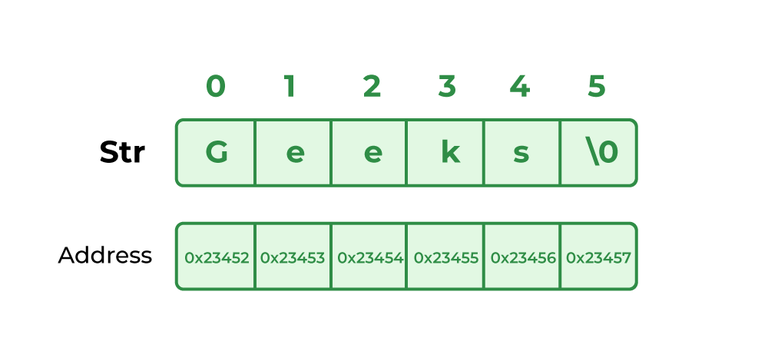
PrintStream out = new PrintStream(System.out);
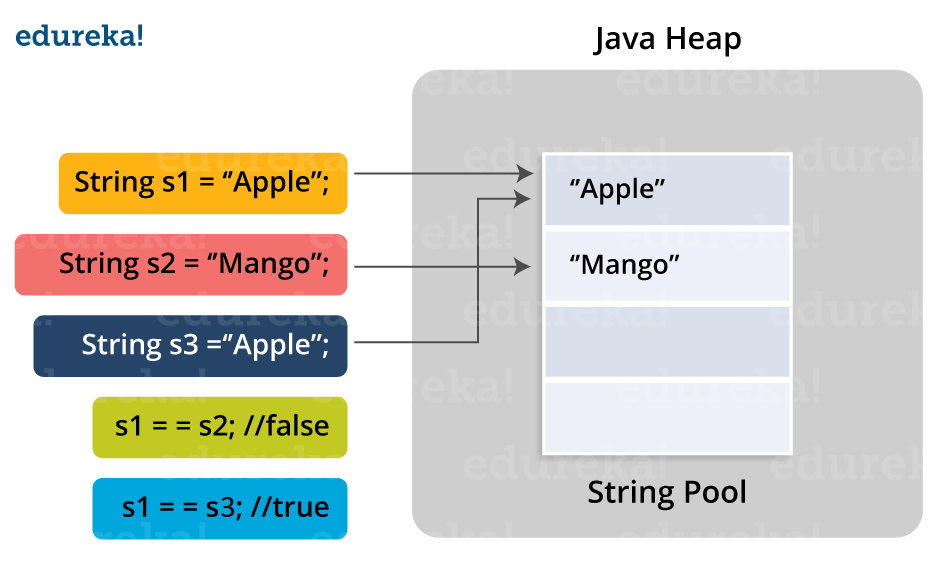
out.write("Hello, World!".getBytes());
out.close();
}
}
import java.io.*;
public class WriteExample {
public static void main(String[] args) {
FileOutputStream out = new FileOutputStream("example.txt");
out.write("Hello, World!".getBytes());
out.close();
}
}
What is String in Java
I'm happy to help you with that!
In Java, a string is a sequence of characters, such as letters, digits, and symbols, that can be used to represent text or other types of data. Strings are one of the most fundamental data types in Java, and they play a crucial role in almost every aspect of programming.
A string in Java is an object of type String
, which is immutable, meaning it cannot be changed once it's created. This immutability ensures that strings are thread-safe and can be shared safely among multiple threads or processes.
Here are some key characteristics of strings in Java:
Immutable: As mentioned earlier, strings are immutable, which means their contents cannot be modified after creation. Character sequences: Strings consist of a sequence of characters, including letters (uppercase and lowercase), digits, spaces, punctuation marks, and special characters like newline (n
) and tab (t
). Unicode support: Java strings use the Unicode character set to represent international characters, making them suitable for text processing involving non-English languages. Length: The length of a string in Java is measured by the number of characters it contains.
Strings are created using various methods:
Literal notation: Strings can be defined as literals, such as"Hello World!"
. String constructor: New strings can be created using the new String()
constructor and passing a character array or a byte array as an argument. Concatenation: Existing strings can be concatenated (combined) using the +
operator or the concat()
method.
Strings are used extensively in Java programming for various purposes:
Text processing: Strings are used to manipulate text data, such as searching, replacing, and formatting text. User input: User input from interfaces like GUIs, web forms, or command-line prompts can be stored in strings. Data storage: Strings can be used to store small amounts of data, like configuration settings or metadata.Some essential string methods in Java include:
length()
: Returns the length (number of characters) of a string. charAt(int index)
: Retrieves the character at the specified index position. indexOf(String str)
and lastIndexOf(String str)
: Searches for the first or last occurrence of a substring within a string, returning its starting index or -1 if not found. substring(int beginIndex)
and substring(int beginIndex, int endIndex)
: Extracts a portion of a string, specified by the beginning and ending indices.
In summary, strings in Java are immutable sequences of characters that can be used for text processing, user input, data storage, and more. Understanding strings is crucial for building robust and effective Java applications.