Java CompletableFuture allOf
Java CompletableFuture allOf
I'm happy to respond in English!
allOf()
is a method provided by the CompletableFuture
class in Java, which allows you to combine multiple completable futures into one that completes when all of them have completed.
Here's an example:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
public class CompletableFutureAllOfExample {
public static void main(String[] args) throws InterruptedException {
// Create three completable futures
CompletableFuture future1 = CompletableFuture.completedFuture("Future 1").thenApply(str -> str.toUpperCase());
CompletableFuture future2 = CompletableFuture.completedFuture("Future 2").thenApply(str -> str.toLowerCase());
CompletableFuture future3 = CompletableFuture.runAsync(() -> {
try {
TimeUnit.SECONDS.sleep(2);
return 42;
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
});
// Use allOf() to combine the futures
CompletableFuture combinedFuture = CompletableFuture.allOf(future1, future2, future3);
// Wait for the combined future to complete
combinedFuture.get();
}
}
In this example, we create three completable futures: future1
, future2
, and future3
. The first two are simple futures that return strings in uppercase and lowercase respectively. The third one simulates some long-running operation by sleeping for 2 seconds.
We then use the allOf()
method to combine these futures into a single future, combinedFuture
. This future completes when all three of the original futures have completed.
Finally, we wait for the combined future to complete using the get()
method. When this happens, we know that all three of our original futures have completed.
The benefits of allOf()
are:
allOf()
, a single exception is propagated if any of the futures fail, making it easier to handle errors. Improved code readability: By combining multiple futures into one, you can write more concise and readable code that's easier to maintain. Faster code execution: When multiple futures are combined with allOf()
, they can be executed concurrently, which can improve the overall performance of your code.
In summary, allOf()
is a powerful method in Java that allows you to combine multiple completable futures into one, making it easier to write concurrent and asynchronous code.
CompletableFuture Java 21
CompletableFuture in Java 21
In Java 8 and later versions, the CompletableFuture
class was introduced as part of the Java API to support asynchronous programming using lambda expressions and method references. It provides a powerful way to handle asynchronous computations by allowing you to compose together multiple tasks that can run concurrently or sequentially.
Key Features
Asynchronous Execution:CompletableFuture
allows you to execute tasks asynchronously, which means that your code can continue running without blocking until the task is completed. Handling Completion: You can handle the completion of a task by providing an onComplete()
method that gets called when the task is finished.
CompletableFuture
instances can be composed together to create complex workflows involving multiple tasks, which can run concurrently or sequentially. Error Handling: You can handle errors that occur during task execution using the whenComplete()
and exceptionally()
methods.
Example Usage
Here's a simple example of how you might use CompletableFuture
in a Java application:
import java.util.concurrent.*;
public class CompletableFutureExample {
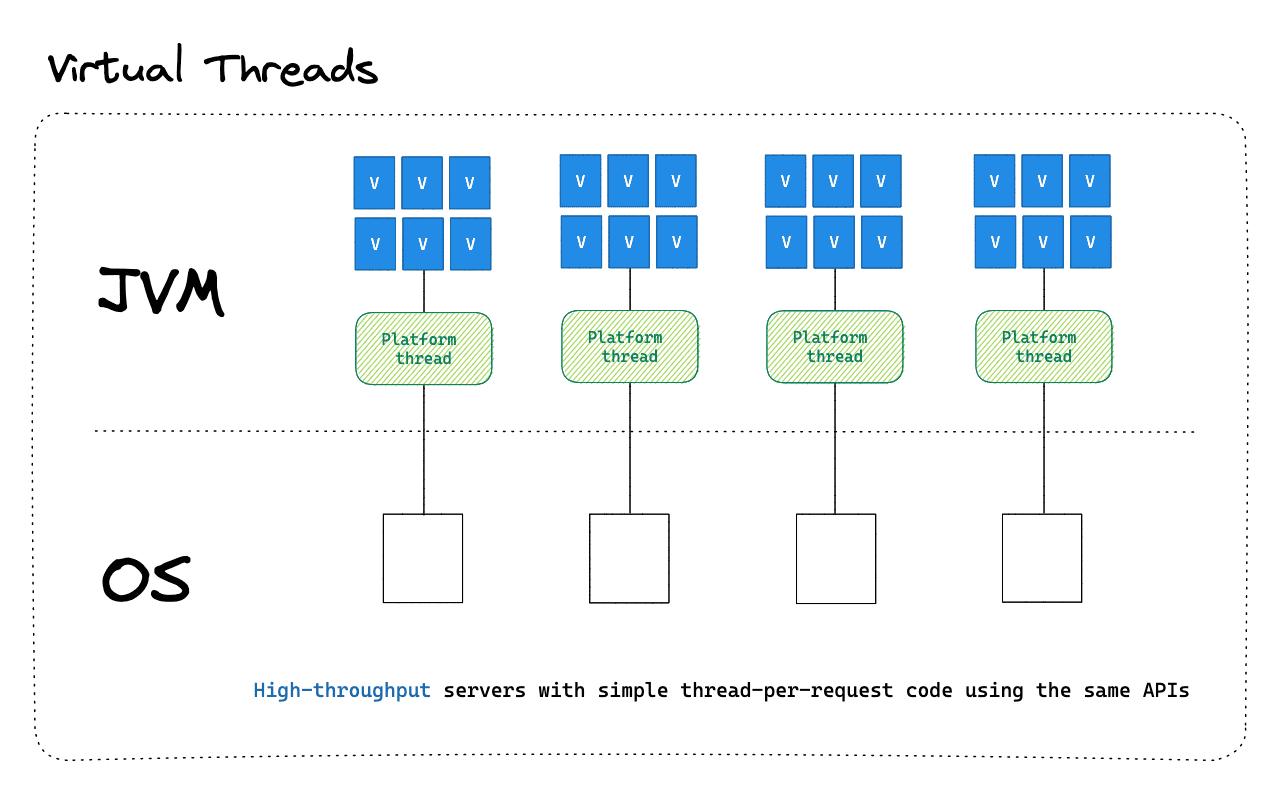
public static void main(String[] args) throws InterruptedException, ExecutionException {
// Create a CompletableFuture that runs after 2 seconds
CompletableFuture future = CompletableFuture.runAfterDelay(() -> {
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
return 42;
});
// Get the result of the future when it's complete
FutureResult result = future.whenComplete((r, ex) -> {
if (ex == null) {
System.out.println("The answer is: " + r.get());
} else {
System.out.println("An error occurred: " + ex.getMessage());
}
});
// Run the main thread for a few seconds to simulate some other work
Thread.sleep(3000);
}
}
Best Practices
When working with CompletableFuture
, it's essential to follow best practices to avoid common pitfalls:
.get()
: The get()
method can block your program if the task has not yet completed, leading to performance issues. Use whenComplete()
instead of .thenApply()
: The whenComplete()
method allows you to handle the completion of a task without blocking, whereas thenApply()
will block until the task is finished. Test for Errors: Always test for errors and handle them appropriately using the exceptionally()
or whenComplete()
methods.
Conclusion
CompletableFuture
provides a powerful way to handle asynchronous computations in Java, allowing you to create complex workflows that can run concurrently or sequentially. By following best practices and understanding its key features and usage, you can effectively use CompletableFuture
to improve the performance of your applications.