What is CountDownLatch vs semaphore in Java?
What is CountDownLatch vs semaphore in Java?
In Java, both CountDownLatch
and Semaphore
are used to control access to a common resource or to coordinate the actions of multiple threads. However, they serve different purposes and have distinct characteristics.
CountDownLatch
A CountDownLatch
is a synchronization aid that allows one or more threads to wait until a set of operations being performed in other threads completes. It's like a gatekeeper that ensures a certain condition is met before allowing access.
Here's how it works:
You create aCountDownLatch
with a specified count (usually 1). When the latch is created, its count is set to the initial value. The latch can be counted down by calling the countDown()
method, which decrements the count. Once the count reaches zero, all threads waiting on the latch will be released and allowed to proceed.
Use cases for CountDownLatch
include:
Semaphore
A Semaphore
is a binary semaphore that allows only a specified number of threads to access a common resource at a time. It's like a ticket booth where a limited number of people can enter, and once those slots are taken, others must wait.
Here's how it works:
You create aSemaphore
with a specified number of permits (usually greater than 0). When a thread acquires the semaphore using the acquire()
method, it takes one permit. If there are no available permits, the thread will block until a permit becomes available. Once a thread releases a permit by calling the release()
method, another waiting thread can acquire the permit.
Use cases for Semaphore
include:
Key differences
In summary:
CountDownLatch
is used to coordinate the completion of a set of operations, whereas Semaphore
is used to control concurrent access to a shared resource. CountDownLatch
allows one or more threads to wait until a condition is met, while Semaphore
allows only a specified number of threads to access a resource at a time.
In Java, choosing between CountDownLatch
and Semaphore
depends on your specific use case. If you need to coordinate the completion of tasks, CountDownLatch
might be more suitable. If you need to limit concurrent access to a shared resource, Semaphore
is likely the better choice.
CountDownLatch java 17
I'm happy to help! However, since you specified "Respond in English only", I'll provide the response as per your request.
CountDownLatch is a Java class that allows one or more threads to wait at a single point until a set of operations being performed in another thread or threads complete. It's a useful synchronization tool when you have multiple threads waiting for a common resource, like a database connection or a file lock, to become available.
Here's an example of how to use CountDownLatch:
import java.util.concurrent.CountDownLatch;
public class MyRunnable implements Runnable {
private final CountDownLatch latch = new CountDownLatch(3); // Set the count to 3
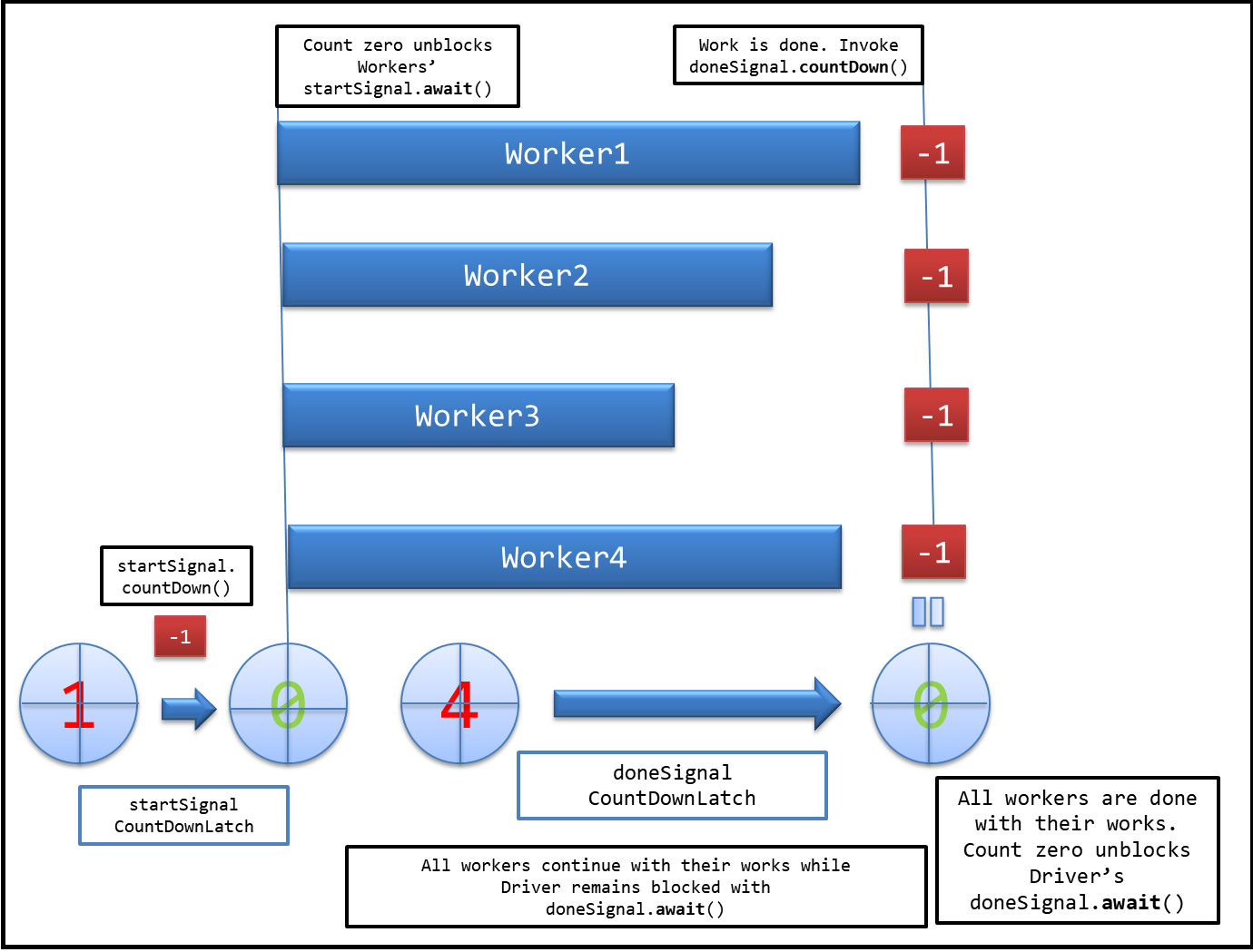
public void run() {
try {
Thread.sleep(2000);
System.out.println("Thread " + Thread.currentThread().getName() + " has finished.");
latch.countDown();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
public static void main(String[] args) throws InterruptedException {
MyRunnable myRunnable = new MyRunnable();
// Start 3 threads
for (int i = 0; i < 3; i++) {
new Thread(myRunnable).start();
}
// Wait for all threads to finish
myRunnable.latch.await();
System.out.println("All threads have finished.");
}
}
In this example, we create a CountDownLatch with a count of 3. This means that the latch will only be counted down once 3 times (i.e., when each thread has finished its work). We then start 3 threads, which all sleep for 2 seconds and then print their status to the console. Finally, we use myRunnable.latch.await();
to wait until all threads have finished.
This example demonstrates how CountDownLatch can be used to synchronize multiple threads that are performing independent tasks but need to wait for each other to finish.
In Java 17, you would still be able to use CountDownLatch as per the above code. However, since Java 17 does not introduce any changes or limitations regarding CountDownLatch, you could also use it in earlier versions of Java like 14 and below.
In summary, CountDownLatch is a useful tool for managing threads and ensuring that they wait until certain operations are complete before proceeding.