How to create an abstract class in Java?
How to create an abstract class in Java?
To create an abstract class in Java, you need to declare the class with the abstract
keyword. An abstract class is a class that cannot be instantiated and serves as a base class for other classes.
Here is a basic example of how to create an abstract class in Java:
public abstract class Animal {
// You can define methods and variables just like any other class
public abstract void makeSound(); // Abstract method, which must be implemented by its subclasses
// Example method implementation
public void sleep() {
System.out.println("The animal is sleeping");
}
}
In this example, Animal
is an abstract class. It has one abstract method makeSound()
and a regular method sleep()
.
To create a concrete subclass from the abstract class, you need to provide implementations for all the abstract methods of its superclass:
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
In this example, Dog
is a concrete subclass that implements the makeSound()
method.
You can also create more subclasses from Animal
, for instance:
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
In this example, Cat
is another concrete subclass that implements the makeSound()
method.
Here are some key points about abstract classes in Java:
Abstract Classes Can't Be Instantiated: You can't create an instance of an abstract class because it's declared with theabstract
keyword. Methods Must be Implemented by Subclasses: Any methods declared as abstract
must be implemented by its subclasses. If a subclass doesn't provide an implementation for an abstract method, it will also need to be declared as abstract. Abstract Classes Can Have Both Abstract and Concrete Methods: You can declare both abstract and concrete methods in an abstract class. The difference is that abstract methods don't have a body (i.e., they are not implemented), while concrete methods do. Concrete Subclasses Must Provide Implementations for All Abstract Methods: A concrete subclass must provide implementations for all the abstract methods declared by its superclass.
Here are some scenarios where you would use abstract classes in Java:
To define a blueprint or template for subclasses: You can declare common attributes and methods that will be shared among your subclasses. To provide a foundation for your hierarchy of classes: Abstract classes serve as a base class for other classes, allowing them to inherit behaviors and characteristics from their parent class. To implement the strategy pattern: In this design pattern, you define an abstract class with methods that can be implemented differently by its subclasses, providing a way to encapsulate different strategies or algorithms.Overall, abstract classes provide a powerful mechanism for organizing your code into logical groups, making it easier to maintain and extend your software.
What is abstract vs encapsulation Java?
I'm happy to help! However, please note that I'll respond in English as per your request. Here's a detailed explanation of abstract and encapsulation in the context of Java programming:
Abstract Class:
In object-oriented programming (OOP), an abstract class is a blueprint or template that defines a common base class for a group of related objects. It provides a way to create a class that can't be instantiated on its own, but serves as a superclass for other classes.
A key feature of abstract classes is that they can't be directly instantiated using the new
keyword. Instead, you need to extend or inherit from an abstract class to create a concrete subclass that can be instantiated.
In Java, you declare an abstract class by using the abstract
keyword before the class declaration. For example:
public abstract class Animal {
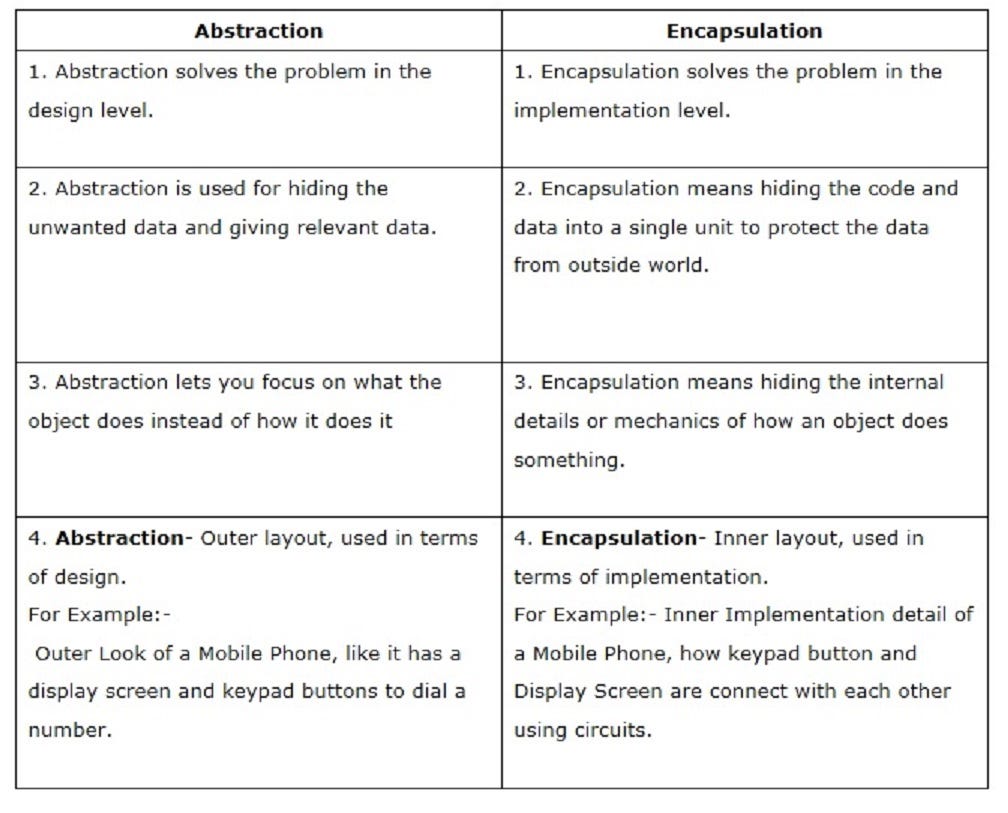
public abstract void makeSound();
}
Abstract classes are useful when you want to define a common base class for a group of related objects that share certain characteristics or behaviors.
Encapsulation:
Encapsulation is a fundamental concept in OOP, which involves hiding the implementation details of an object from the outside world and only exposing the necessary information through public methods or properties. In other words, encapsulation is about bundling data and behavior together into a single unit called an object.
In Java, encapsulation is achieved by making instance variables private (using the private
access modifier) and providing getter and setter methods to access those variables. For example:
public class Employee {
private String name;
public void setName(String name) { this.name = name; }
public String getName() { return this.name; }
}
By encapsulating data and behavior, you can achieve several benefits, such as:
Data hiding: The internal state of an object is hidden from the outside world. Abstraction: An object's implementation details are abstracted away from other objects or parts of your program. Improved security: You can control access to sensitive data or behavior by using private variables and public methods. Easier maintenance: Encapsulating related data and behavior makes it easier to modify or extend an object without affecting other parts of the system.In summary, abstract classes provide a way to define a common base class for a group of related objects, while encapsulation is about bundling data and behavior together into a single unit called an object. By using these concepts in Java programming, you can create robust, maintainable, and scalable software systems.