Java socket client example
Java socket client example
Here is a simple example of a Java Socket Client:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.Socket;
public class SocketClient {
public static void main(String[] args) throws IOException {
// Define the server's IP address and port
String serverIP = "localhost";
int serverPort = 1234;
// Create a socket object
Socket socket = new Socket(serverIP, serverPort);
// Get the input and output streams
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
// Send data to the server
String message = "Hello from client!";
out.println(message);
System.out.println("Client sent: " + message);
// Receive data from the server
String response;
while ((response = in.readLine()) != null) {
System.out.println("Server response: " + response);
}
// Close the socket
socket.close();
}
}
This Java program demonstrates a basic client-server communication using Socket programming. Here's what it does:
Connect to Server: Themain
method creates a Socket
object and connects to a server at IP address "localhost"
and port number 1234
. Get Input/Output Streams: The program gets the input stream (in
) from the socket, which allows it to receive data from the server, and the output stream (out
), which enables sending data to the server. Send Data to Server: The client sends a message "Hello from client!"
to the server using the PrintWriter
. Receive Data from Server: The program receives lines of text from the server using a loop that reads from the input stream (in
). Each line is printed to the console. Close Socket: Finally, the socket is closed using the close
method.
Note: This code assumes that there is a corresponding server-side Java program running at the specified IP address and port number. For example, you could use the following Java server-side code:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
public class SocketServer {
public static void main(String[] args) throws IOException {
// Define the port for our server
int port = 1234;
// Create a ServerSocket object
ServerSocket server = new ServerSocket(port);
// Wait for client connection
System.out.println("Waiting for client...");
Socket socket = server.accept();
// Get the input and output streams
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
// Receive data from client
String message;
while ((message = in.readLine()) != null) {
System.out.println("Received: " + message);
// Send response back to the client
out.println("Hello, " + message + "!");
}
// Close the socket and server
socket.close();
server.close();
}
}
This code runs a simple echo server that receives messages from clients, prints them to the console, and sends responses back.
Remember: For this example to work, both the client and server programs need to be run simultaneously.
ServerSocket Java example
Here is a simple ServerSocket example written in Java:
In this example, we are creating a basic server that listens for incoming connections on port 8000.
import java.net.*;
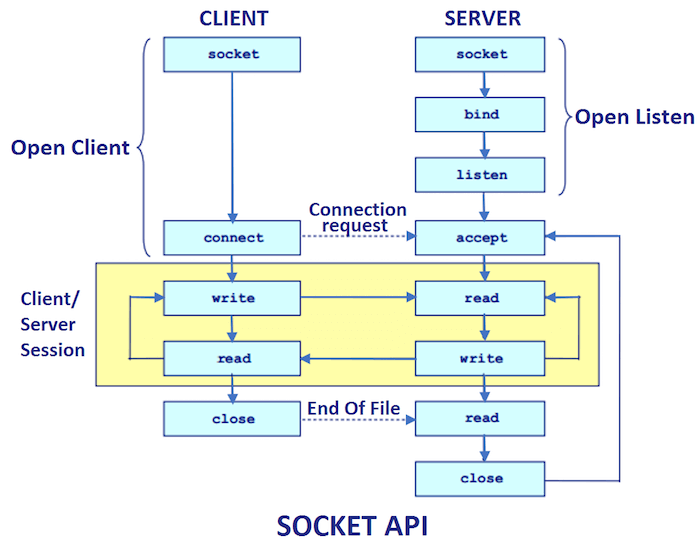
import java.io.*;
public class SocketServer {
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server is listening...");
// Run indefinitely until a termination request.
while (true) {
Socket clientSocket = serverSocket.accept();
System.out.println("Connection accepted");
// Get the client's IP address and port number.
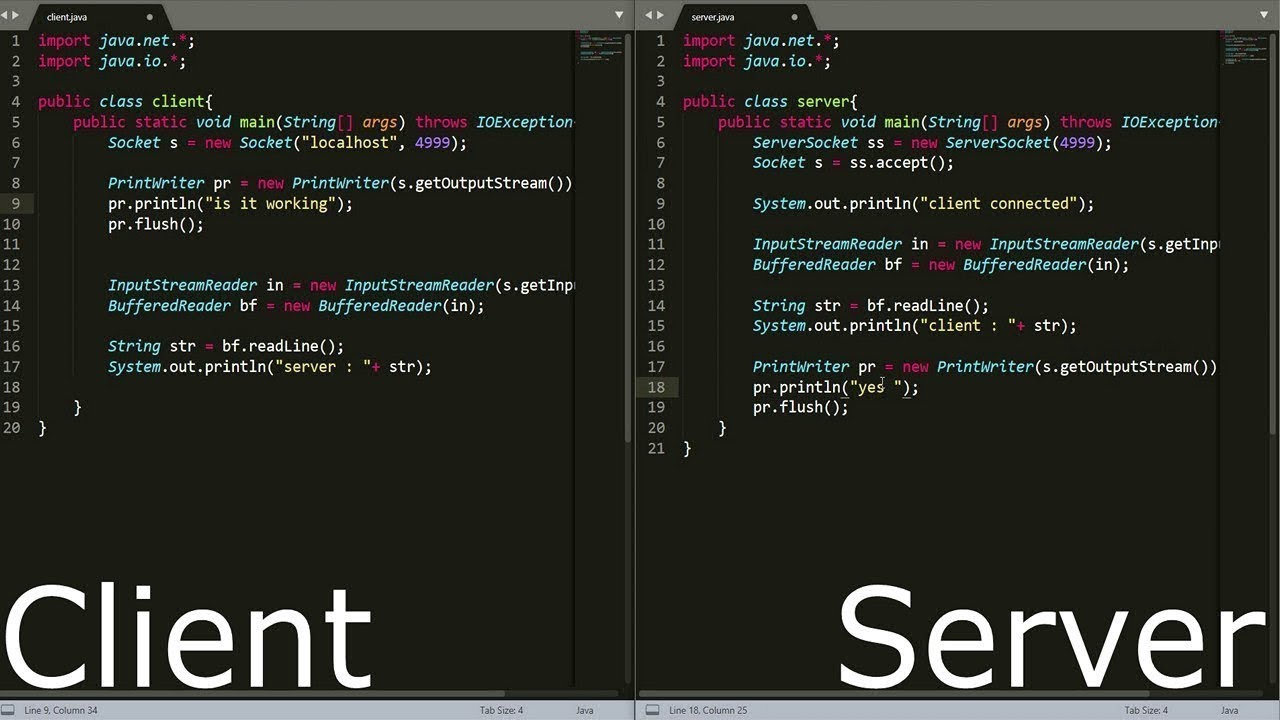
InetAddress clientAddress = clientSocket.getInetAddress();
int clientPort = clientSocket.getPort();
// Create a new thread to handle the client request.
Thread t = new ClientThread(clientSocket);
t.start();
}
}
static class ClientThread extends Thread {
Socket clientSocket;
public ClientThread(Socket s) {
this.clientSocket = s;
}
public void run() {
try {
// Read from the client's socket.
BufferedReader reader = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println("Received message from client: " + line);
// Do something with the received data.
// Here, we are just sending it back to the client.
PrintWriter writer = new PrintWriter(clientSocket.getOutputStream(), true);
writer.println(line);
}
} catch (IOException e) {
System.out.println("Exception caught while reading from socket");
e.printStackTrace();
} finally {
try {
this.clientSocket.close();
} catch (IOException e) {
System.out.println("Error closing the socket");
e.printStackTrace();
}
}
}
}
}
Here's a breakdown of what each part does:
The first step is to create a ServerSocket object, specifying which port to listen on. Then we run an infinite loop that waits for incoming connections using theaccept()
method. Once a connection is established, we get the client's IP address and port number. We then start a new thread (ClientThread
) that handles communication with this client. The ClientThread reads from the client's socket in an infinite loop, until the client closes their end of the connection. For each line received, it sends back any message you want.
ServerSocket example is quite basic and may need additional error handling or additional logic based on your specific requirements.
I hope this helps!