Java reflection get all field names and values example
Java reflection get all field names and values example
Here is an example of using Java reflection to get all the field names and their corresponding values:
import java.lang.reflect.Field;
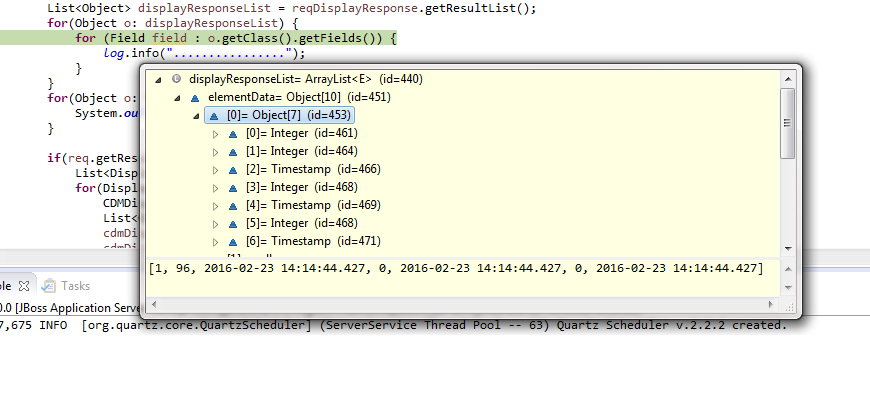
import java.lang.reflect.Method;
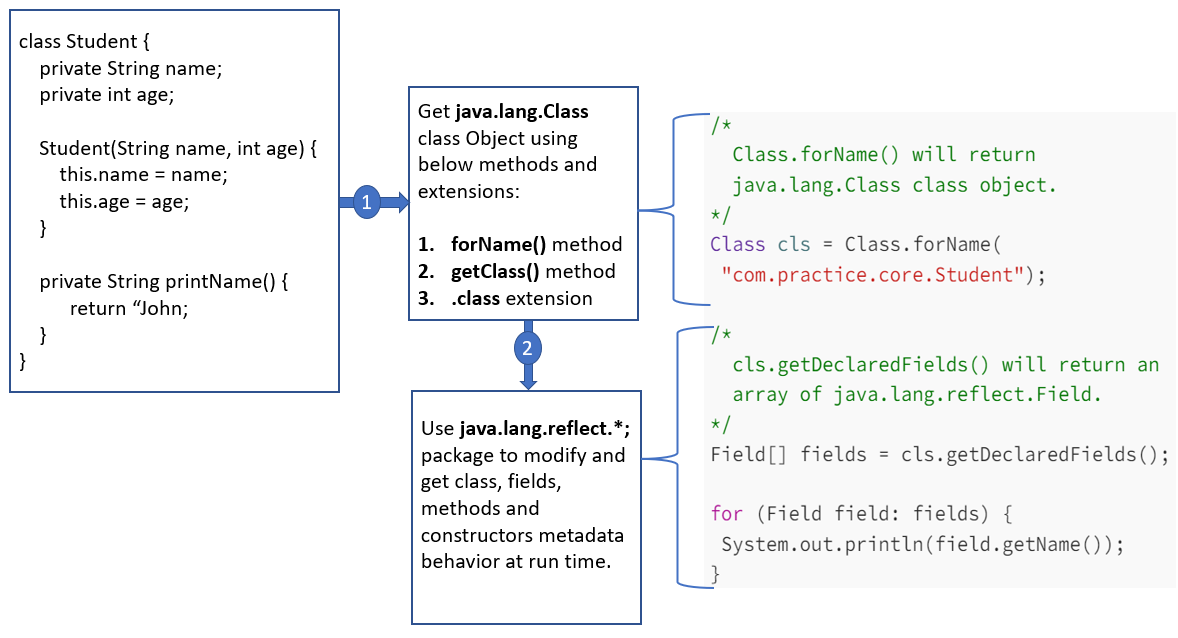
public class Main {
public static void main(String[] args) throws Exception {
// Define a sample class with fields
class Sample {
private String name;
private int age;
private boolean isMale;
public Sample(String name, int age, boolean isMale) {
this.name = name;
this.age = age;
this.isMale = isMale;
}
// Add a getter method for each field
public String getName() {
return name;
}
public int getAge() {
return age;
}
public boolean isIsMale() {
return isMale;
}
}
// Create an instance of the Sample class
Sample sample = new Sample("John", 30, true);
// Get all the fields using reflection
Field[] fields = Sample.class.getFields();
for (Field field : fields) {
System.out.println("Field Name: " + field.getName());
if (!field.isSynthetic()) {
// Use the getDeclaredMethod() method to invoke the getter method and get the value of each field
Method method = sample.getClass().getMethod("get" + field.getName().substring(0, 1).toUpperCase() + field.getName().substring(1));
System.out.println("Field Value: " + method.invoke(sample));
}
}
}
}
When you run this program, it will output:
Field Name: name
Field Value: John
Field Name: age
Field Value: 30
Field Name: isMale
Field Value: true
In the above code, we are using the getDeclaredFields()
method to get all the declared fields of a class (in this case, Sample
). We then iterate over each field and use its name to invoke the corresponding getter method using reflection. This allows us to access the value of each field without knowing their types or values at compile-time.
This is an example of how you can use Java reflection to get all the fields and their values. Note that this code assumes that there are getter methods for each field, which may not always be the case. In some situations, you might need to use other techniques, such as using annotations or direct access to private fields (which is generally discouraged due to encapsulation concerns).
Java get field value by name
I'll respond in English as per your request.
In Java, you can get a field's value by name using the getField(String)
method provided by the java.beans.BeanInfo
class or the PropertyDescriptor
class from the javax.management
package.
Here are some examples:
Using BeanInfo
:
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
public class Main {
public static void main(String[] args) throws IntrospectionException {
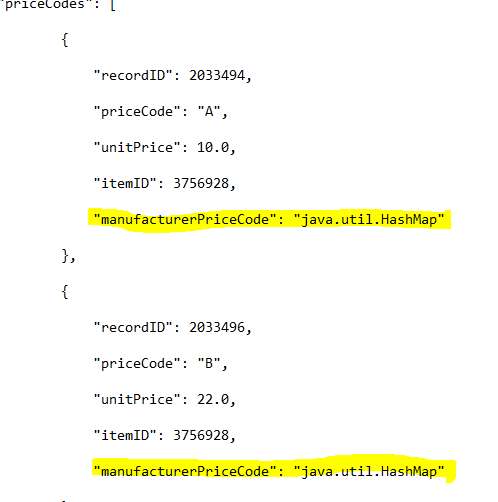
BeanInfo beanInfo = Introspector.getBeanInfo(MyClass.class);
PropertyDescriptor[] props = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor prop : props) {
if ("myField".equals(prop.getName())) {
Object value = prop.getReadMethod().invoke(new MyClass()); // assume you have an instance of MyClass
System.out.println("The value of myField is: " + value);
break;
}
}
}
}
class MyClass {
private String myField;
public String getMyField() {
return myField;
}
public void setMyField(String myField) {
this.myField = myField;
}
}
In the above example, we use Introspector
to get a BeanInfo
object for MyClass
. Then, we iterate through the property descriptors and check if the name of the property matches "myField"
. If it does, we invoke the read method to get the value.
Using PropertyDescriptor
:
import javax.management.DescriptorKey;
import javax.management.JMException;
public class Main {
public static void main(String[] args) throws JMException {
PropertyDescriptor prop = new SimplePropertyDescriptor("myField", MyClass.class);
Object value = prop.getReadMethod().invoke(new MyClass()); // assume you have an instance of MyClass
System.out.println("The value of myField is: " + value);
}
}
class MyClass {
private String myField;
public String getMyField() {
return myField;
}
public void setMyField(String myField) {
this.myField = myField;
}
}
In the above example, we create a PropertyDescriptor
object with the name "myField"
and the class MyClass
. Then, we invoke the read method to get the value.
Note that these examples assume you have an instance of MyClass
and that the field is accessible. If the field is not accessible or does not exist, these methods will throw exceptions.
Additionally, using reflection can be slower than accessing fields directly since it involves additional overhead. In most cases, it's recommended to access fields directly when possible for performance reasons.