Java Matcher find
Java Matcher find
I can respond to you in English, and I'll provide an explanation of how to use the Java Matcher class.
The Matcher
class is a part of the Java Pattern API that allows you to perform regular expression matching operations. It's often used with the Pattern
class to compile regular expressions and then use them to search for patterns within strings.
Here's how you can use it:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
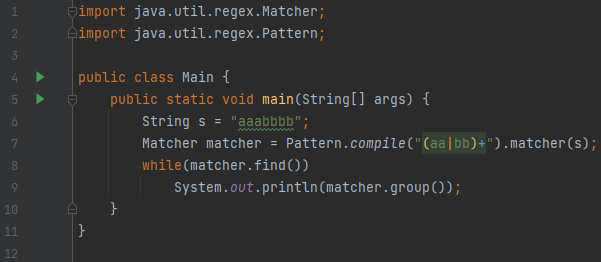
public class Main {
public static void main(String[] args) {
// Create a Pattern object
String regex = "hello"; // the pattern we're looking for
Pattern pattern = Pattern.compile(regex);
// Create a Matcher object
String text = "Hello, world! Hello, again!"; // the text to search through
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Found 'hello' at position " + matcher.start());
while (matcher.find()) {
System.out.println("Found another 'hello' at position " + matcher.start());
}
} else {
System.out.println("Pattern not found");
}
}
}
In this example, we're looking for the pattern "hello"
in a string of text. We compile the pattern using Pattern.compile
, and then create a Matcher
object from it.
We can then use the find()
method to see if the pattern is found at least once in the text. If it is, we can use the start()
method to get the starting position of the match. The find()
method returns a boolean indicating whether the pattern was found, and also advances the matcher to the next possible match.
The find()
method will keep finding matches until there are no more matches left. We can use it in a loop to find all occurrences of a pattern within a string.
Here's how you can modify this code to count the number of times a pattern is found:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Main {
public static void main(String[] args) {
// Create a Pattern object
String regex = "hello"; // the pattern we're looking for
Pattern pattern = Pattern.compile(regex);
// Create a Matcher object
String text = "Hello, world! Hello, again!"; // the text to search through
Matcher matcher = pattern.matcher(text);
int count = 0;
while (matcher.find()) {
count++;
}
System.out.println("Found 'hello' " + count + " times");
}
}
In this version of the code, we use a loop to call find()
until there are no more matches. We keep track of how many times the pattern is found by incrementing the count
variable each time through the loop.
This is just a simple example of what you can do with Java's Matcher
class. It has many other features, such as checking if a match is at the end of the text or within a specific group of characters, that can be useful depending on your needs.
How to group a string in Java?
I'm happy to respond in English for this question.
In Java, grouping strings can be achieved through various methods and libraries. Here are some approaches:
1. Using Regular Expressions (RegEx): The java.util.regex
package allows you to work with regular expressions. You can use the Pattern
and Matcher
classes to group strings based on specific patterns.
Example:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class GroupString {
public static void main(String[] args) {
String str = "Hello World, this is a test string.";
Pattern pattern = Pattern.compile("([a-zA-Z]+)"); // Group words
Matcher matcher = pattern.matcher(str);
while (matcher.find()) {
System.out.println(matcher.group(1)); // Prints each word separately
}
}
}
2. Using String Manipulation Methods: Java provides various methods to manipulate strings, such as split()
and substring()
. You can use these methods to group strings based on specific conditions.
Example:
public class GroupString {
public static void main(String[] args) {
String str = "John, Mary, David, Alice";
String[] names = str.split(","); // Split the string by comma
for (String name : names) {
System.out.println(name); // Prints each name separately
}
}
}
3. Using Java 8's java.util.function
Package: With Java 8, you can use lambda expressions and method references to group strings.
Example:
import java.util.Arrays;
import java.util.stream.Collectors;
public class GroupString {
public static void main(String[] args) {
String str = "Hello World, this is a test string.";
Arrays.stream(str.split(" ")) // Split the string by space
.map(word -> word.toLowerCase()) // Convert to lowercase
.distinct() // Remove duplicates
.forEach(System.out::println); // Prints each unique word separately
}
}
4. Using Third-Party Libraries: There are many third-party libraries available that provide advanced string manipulation capabilities, such as Apache Commons Lang and Google Guava.
Example using Apache Commons Lang:
import org.apache.commons.lang3.StringUtils;
public class GroupString {
public static void main(String[] args) {
String str = "Hello World, this is a test string.";
String[] words = StringUtils.split(str, " "); // Split the string by space
for (String word : words) {
System.out.println(word); // Prints each word separately
}
}
}
These are just a few examples of how you can group strings in Java. The approach depends on your specific requirements and the complexity of the grouping operation.
I hope this helps!