How to create get URL in Java?
How to create get URL in Java?
I'm happy to help! However, please note that I'll respond in English as per your request.
To create a GET URL in Java, you can use the java.net.URL
class or the org.apache.http.client.utils.URIBuilder
class from Apache HttpComponents.
Here's an example using the URL
class:
import java.net.*;
public class Main {
public static void main(String[] args) throws MalformedURLException {
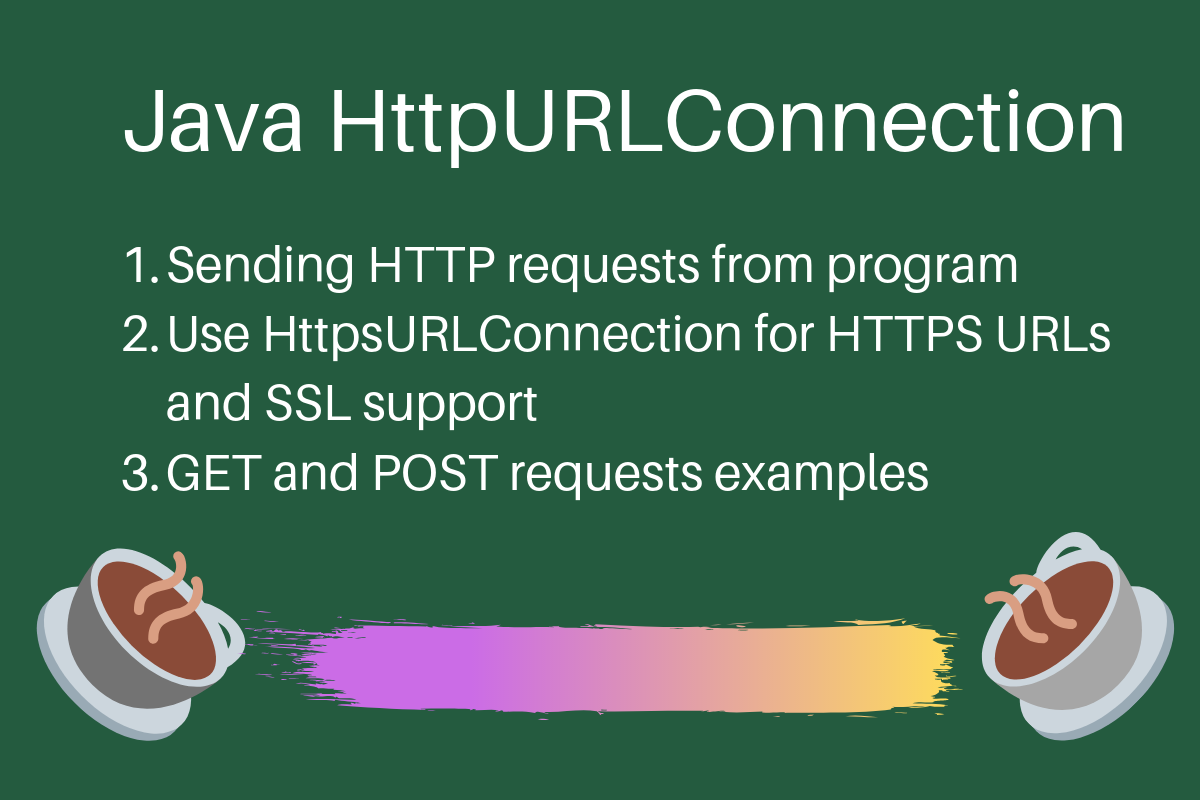
String urlStr = "http://example.com/path?param1=value1¶m2=value2";
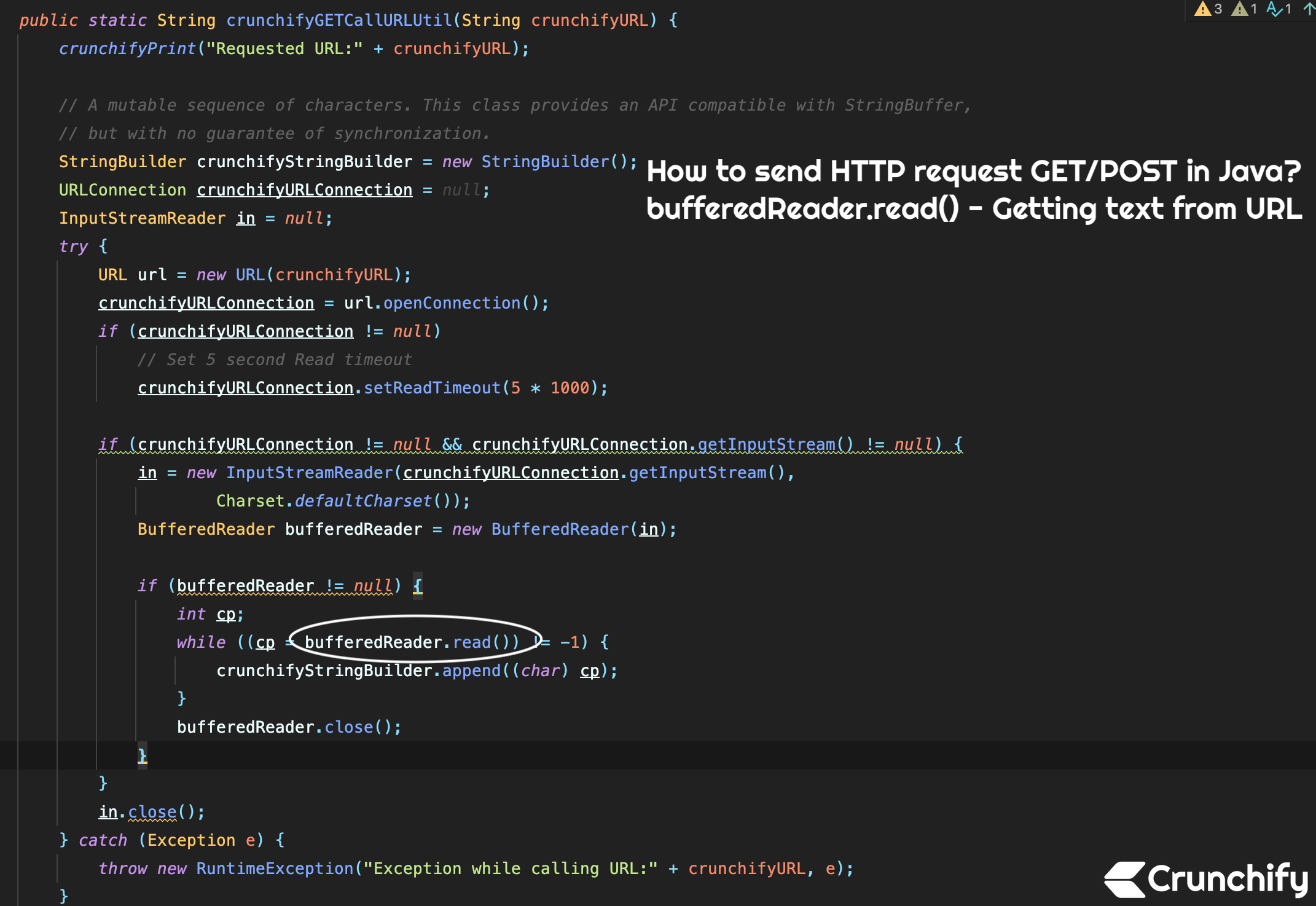
URL url = new URL(urlStr);
System.out.println("GET URL: " + url.toString());
}
}
In this example, we create a URL
object from the string "http://example.com/path?param1=value1¶m2=value2"
. The resulting URL
object represents a GET request to the specified URL.
Now, let's explore the Apache HttpComponents way of creating a GET URL:
import org.apache.http.client.utils.URIBuilder;
public class Main {
public static void main(String[] args) throws Exception {
String urlStr = "http://example.com/path";
URIBuilder builder = new URIBuilder(urlStr);
builder.setParameter("param1", "value1");
builder.setParameter("param2", "value2");
URL getURL = builder.build();
System.out.println("GET URL: " + getURL.toString());
}
}
In this example, we create a URIBuilder
object from the string "http://example.com/path"
. Then, we add parameters to the URIBuilder
using the setParameter
method. Finally, we call the build
method to build the GET URL.
Both examples demonstrate how to create a GET URL in Java. The main difference is that the Apache HttpComponents approach provides more flexibility and control over the creation of the URL.
I hope this helps!
Java build URL with query parameters
I'm happy to respond in English!
Java provides a built-in API for constructing URLs that can include query parameters. The URI
class is part of the Java Standard Edition (SE) and is used to create URIs, which are used to identify resources over the Internet.
To build a URL with query parameters using Java, you can use the URIBuilder
or URI
classes. Here's an example:
import java.net.URI;
import java.net.URISyntaxException;
public class Main {
public static void main(String[] args) throws URISyntaxException {
String baseUri = "http://example.com";
String queryParam1 = "param1=value1";
String queryParam2 = "param2=value2";
URI uri = new URI(baseUri + "?" +
queryParam1 + "&" +
queryParam2);
System.out.println(uri.toString());
}
}
This code will output the following URL:
http://example.com?param1=value1¶m2=value2
In this example, we're using the URI
class to create a new URI. The constructor takes three parameters: the scheme (in this case, "http"), the authority (which is optional), and the path (also optional). We're also appending query parameters to the end of the URL.
The URIBuilder
class provides an easier way to build URIs by providing setter methods for various components. Here's an example:
import org.apache.commons.uri.builder.UriBuilder;
public class Main {
public static void main(String[] args) {
UriBuilder uriBuilder = new UriBuilder("http://example.com");
uriBuilder.addParameter("param1", "value1");
uriBuilder.addParameter("param2", "value2");
URI uri = uriBuilder.build();
System.out.println(uri.toString());
}
}
This code will also output the following URL:
http://example.com?param1=value1¶m2=value2
The addParameter
method is used to add query parameters to the URI. You can chain multiple calls to this method together to build a complex query string.
Both of these examples demonstrate how you can use Java to construct URLs that include query parameters. The specific approach you choose will depend on your needs and preferences.