Java inheritance example w3schools
Java inheritance example w3schools
I'm happy to help you with the Java inheritance example from W3Schools!
Here's an example of how you can use Java inheritance:
// Parent class (Vehicle)
class Vehicle {
private String color;
public Vehicle(String color) {
this.color = color;
}
public void display() {
System.out.println("I am a " + color + " vehicle.");
}
}
// Child class (Car) inherits from the Vehicle class
class Car extends Vehicle {
private int speed;
public Car(String color, int speed) {
super(color); // Calls the parent constructor
this.speed = speed;
}
@Override
public void display() {
System.out.println("I am a " + getColor() + " car that goes at a speed of " + speed + ".");
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car("red", 120);
myCar.display(); // Output: I am a red car that goes at a speed of 120.
Vehicle v = myCar;
v.display(); // Output: I am a red vehicle.
}
}
In this example, Vehicle
is the parent class and Car
is the child class. The Car
class inherits properties from the Vehicle
class. You can see that the Car
constructor calls the Vehicle
constructor using super(color)
.
The display()
method in the Car
class overrides the one in the Vehicle
class, providing a specific implementation for cars. In this example, when you create an instance of Car
and call its display()
method, it prints out a message with the car's color and speed.
In the Main
class, we demonstrate inheritance by creating an instance of Car
, calling its display()
method, and then casting that instance to Vehicle
. When we call the display()
method on the Vehicle
reference, we see that it inherits the behavior from the parent class.
I hope this helps!
What is inheritance in Java example?
I'd be happy to explain inheritance in Java with an example!
Inheritance in Java is a mechanism that allows one class (the subclass or derived class) to inherit the properties and behavior of another class (the superclass or parent class). This concept is based on the "is-a" relationship, where the subclass is considered a special type of the superclass.
Here's a simple example:
Let's say we have an animal called "Mammal" with characteristics like the ability to give birth to live young and produce milk for their offspring. Now, let's create a new animal called "Dog", which inherits the characteristics of Mammal but has some additional features unique to dogs.
public class Animal {
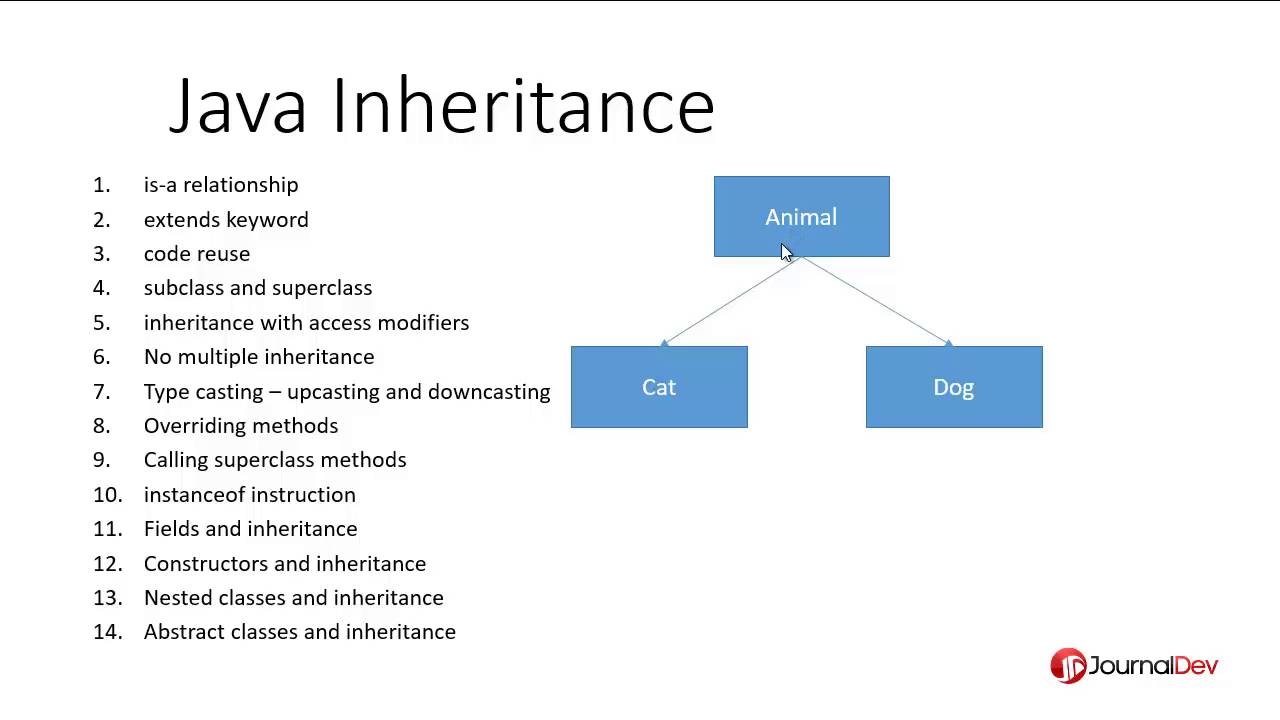
public void eat() {
System.out.println("The animal is eating.");
}
}
public class Mammal extends Animal {
public void giveBirth() {
System.out.println("The mammal gives birth.");
}
public void produceMilk() {
System.out.println("The mammal produces milk for its offspring.");
}
}
public class Dog extends Mammal {
public void bark() {
System.out.println("The dog barks.");
}
}
In this example:
Animal
is the superclass (or parent class). Mammal
inherits from Animal
, which means it has access to all the methods and fields of Animal
. In addition, Mammal
adds its own specific characteristics (giveBirth()
and produceMilk()
) that are unique to mammals. Dog
inherits from Mammal
, which means it also has access to all the methods and fields of both Animal
and Mammal
. In addition, Dog
adds its own specific characteristic (bark()
), which is unique to dogs.
Now let's create an instance of Dog
and call some of these methods:
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat(); // calls the eat() method from Animal
myDog.giveBirth(); // calls the giveBirth() method from Mammal
myDog.produceMilk(); // calls the produceMilk() method from Mammal
myDog.bark(); // calls the bark() method from Dog
}
}
When we run this program, it will output:
The animal is eating.
The mammal gives birth.
The mammal produces milk for its offspring.
The dog barks.
As you can see, inheritance allows us to create a hierarchy of classes with shared characteristics and behavior. This helps simplify code by allowing us to reuse methods and fields from superclasses, while also enabling us to add unique features specific to subclasses.
Does this example help illustrate the concept of inheritance in Java?