How to send data from server to client in socket programming in Java?
How to send data from server to client in socket programming in Java?
I'm happy to help!
In Java socket programming, sending data from the server to the client involves several steps:
Creating a Socket: First, create a socket on both the server and the client. The server creates a server socket (ServerSocket), while the client creates a client socket (Socket). The server socket listens for incoming connections, whereas the client socket connects to the server.// Server-side:
ServerSocket serverSocket = new ServerSocket(8000);
Socket clientSocket = serverSocket.accept();
// Client-side:
Socket clientSocket = new Socket("localhost", 8000);
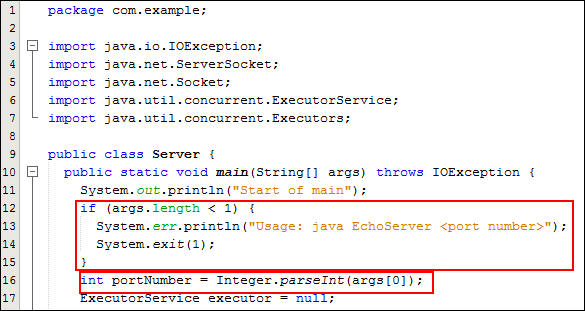
// Server-side:
InputStream in = clientSocket.getInputStream();
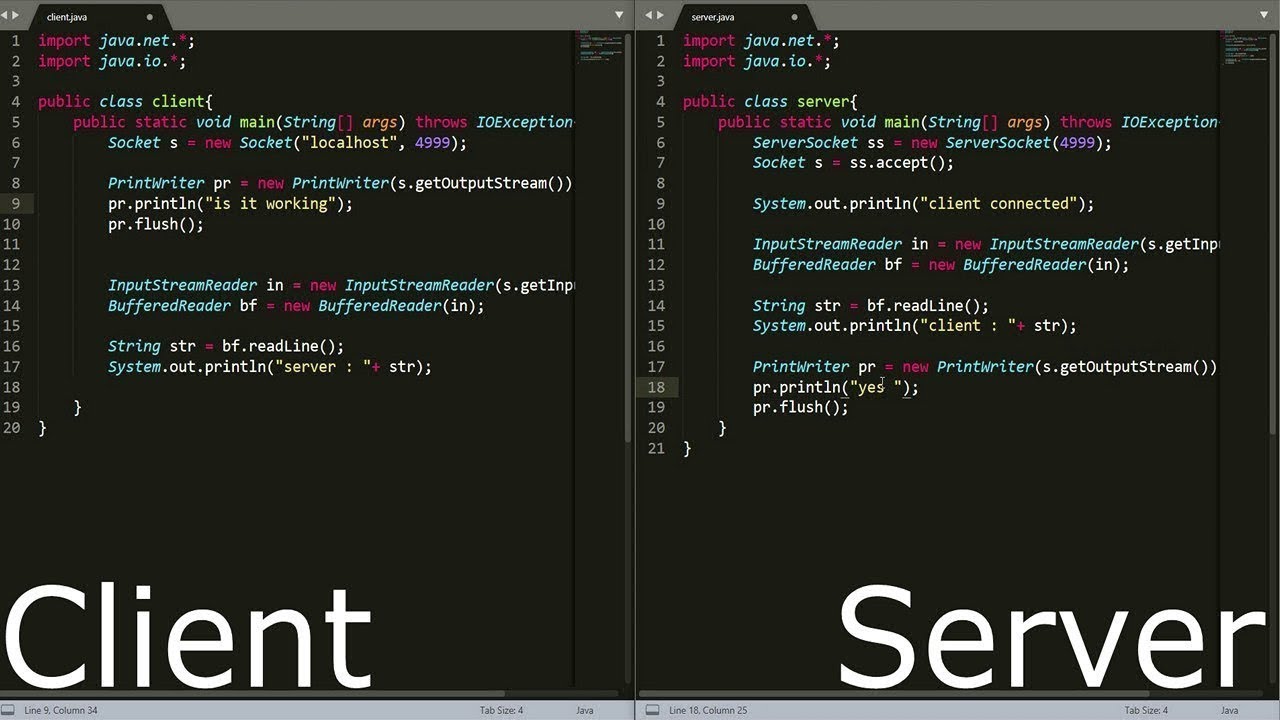
OutputStream out = clientSocket.getOutputStream();
// Client-side:
InputStream in = clientSocket.getInputStream();
OutputStream out = clientSocket.getOutputStream();
Sending Data: On the server side, write the data to be sent into the output stream using a DataOutputStream
. On the client side, read the data from the input stream using a BufferedReader
.
// Server-side:
DataOutputStream dos = new DataOutputStream(out);
dos.writeUTF("Hello Client!");
dos.close();
// Client-side:
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String message = reader.readLine();
reader.close();
Closing Sockets: Finally, close the sockets to free up system resources:
clientSocket.close();
serverSocket.close();
Here is a complete example of sending data from server to client in Java socket programming:
import java.io.*;
import java.net.*;
public class SocketServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server started. Listening for incoming connections...");
Socket clientSocket = serverSocket.accept();
System.out.println("Connection established with " + clientSocket.getInetAddress());
BufferedReader reader = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
DataOutputStream dos = new DataOutputStream(clientSocket.getOutputStream());
String message = "Hello Client!";
dos.writeUTF(message);
dos.close();
System.out.println("Sent message: " + message);
reader.close();
clientSocket.close();
}
}
import java.io.*;
import java.net.*;
public class SocketClient {
public static void main(String[] args) throws IOException {
Socket clientSocket = new Socket("localhost", 8000);
System.out.println("Connected to server");
BufferedReader reader = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
String message = reader.readLine();
System.out.println("Received message: " + message);
reader.close();
clientSocket.close();
}
}
This example demonstrates a simple text-based communication between the server and client. The server sends a predefined message to the client, which reads and prints it.
Remember that this is just a basic example of socket programming in Java, and you may need to add error handling, implement more advanced protocols, or use additional libraries depending on your specific requirements.
Client server socket programming in java example
Here is an example of client-server socket programming in Java:
Server Side Code
import java.net.*;
import java.io.*;
public class Server {
public static void main(String[] args) throws IOException {
// Create a server socket
ServerSocket server = new ServerSocket(8000);
System.out.println("Server started. Listening for incoming connections...");
while (true) {
// Wait for a client to connect
Socket socket = server.accept();
System.out.println("Incoming connection from " + socket.getInetAddress());
// Create a separate thread to handle the client request
Thread t = new ServerThread(socket);
t.start();
}
}
}
class ServerThread extends Thread {
Socket socket;
public ServerThread(Socket s) {
this.socket = s;
}
public void run() {
try {
// Read from the client
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received message from client: " + inputLine);
}
// Send a response back to the client
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
out.println("Hello, client!");
} catch (IOException e) {
System.out.println("Error handling client request");
} finally {
try {
socket.close();
} catch (Exception e) {
// Ignore exception on close
}
}
}
}
Client Side Code
import java.net.*;
import java.io.*;
public class Client {
public static void main(String[] args) throws IOException {
// Create a client socket
Socket socket = new Socket("localhost", 8000);
System.out.println("Connected to the server");
// Send a message to the server
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
out.println("Hello, server!");
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received response from server: " + inputLine);
}
// Close the connection
socket.close();
}
}
In this example, the server creates a ServerSocket
and listens for incoming connections. When a client connects, it creates a separate thread to handle the client's request. The thread reads from the client and sends a response back.
On the client side, it creates a Socket
to connect to the server. It then sends a message to the server and receives any responses. Finally, it closes the connection.
This is a basic example of client-server socket programming in Java. In a real-world scenario, you would likely want to add more error handling and possibly use more advanced networking techniques.
Note: Make sure to run the server code first, then the client code. The server will print out messages as clients connect and send requests.