What is the use case of completablefuture in java javatpoint
What is the use case of completablefuture in java javatpoint
The CompletableFuture class in Java is a part of the java.util.concurrent package, which helps manage asynchronous computations with ease. The main purpose of this class is to provide a future that can be completed manually or automatically.
One major use case for CompletableFuture is when you need to handle both successful and failed results from an asynchronous operation. A CompletableFuture represents a delayed computation whose result will not be available until the computation has completed.
Let's consider a scenario where you want to asynchronously compute some data, such as making an HTTP request or performing some complex calculations. You would typically use FutureTask class to represent this computation and get its result once it completes.
However, when dealing with more complex scenarios like handling exceptions, retrying operations, or processing results in parallel, the traditional Future API becomes cumbersome. This is where CompletableFuture comes into play.
With CompletableFuture, you can create a future that represents some asynchronous operation and then manually complete it by providing a result or throwing an exception. If the operation completes successfully, you can retrieve its result using the get() method or methods from the CompletionStage API (such as toList(), toMap(), etc.).
Here are some scenarios where CompletableFuture is useful:
Asynchronous HTTP Requests: When making asynchronous HTTP requests using a library like OkHttp or Apache HttpClient, you can create a CompletableFuture to represent the request and manually complete it once the response is received. Parallel Processing: If you have multiple computationally expensive tasks that need to be performed in parallel, you can use CompletableFutures to create an array of futures representing these tasks and then manually complete them as they finish. Error Handling: When dealing with asynchronous operations that can fail or throw exceptions, using a CompletableFuture allows you to catch and handle these exceptions more easily. Retrying Operations: You can use a completable future to create an operation that retries itself if it fails. For example, you could write an API client that retries making an HTTP request if the initial attempt fails. Creating Composable Operations: CompletableFutures are very useful when creating composable operations. For instance, you might have an operation that performs some data processing and then uses the result to perform another operation. This can be done easily using CompletableFutures.In summary, CompletableFuture is a powerful API in Java that provides more flexibility and control when dealing with asynchronous computations. Its ability to manually complete futures or handle exceptions makes it particularly useful for handling complex scenarios.
CompletableFuture example in Java
I'd be happy to help!
Java's CompletableFuture is a powerful API for handling asynchronous tasks. It provides a way to handle callbacks and futures in a more concise and readable manner.
Here's an example of using CompletableFuture:
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
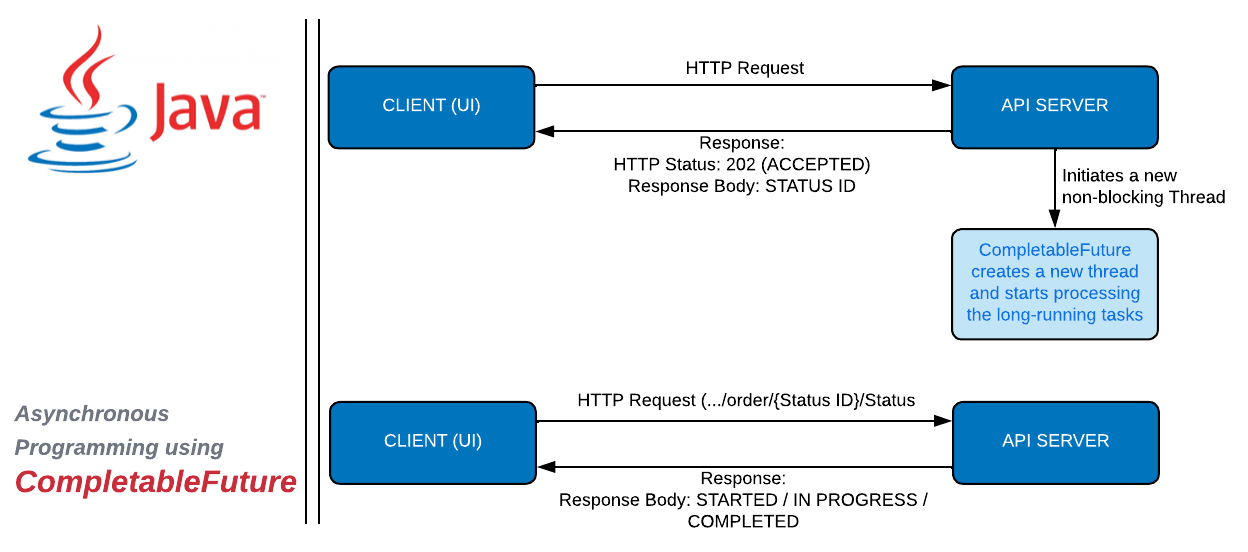
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CompletableFutureExample {
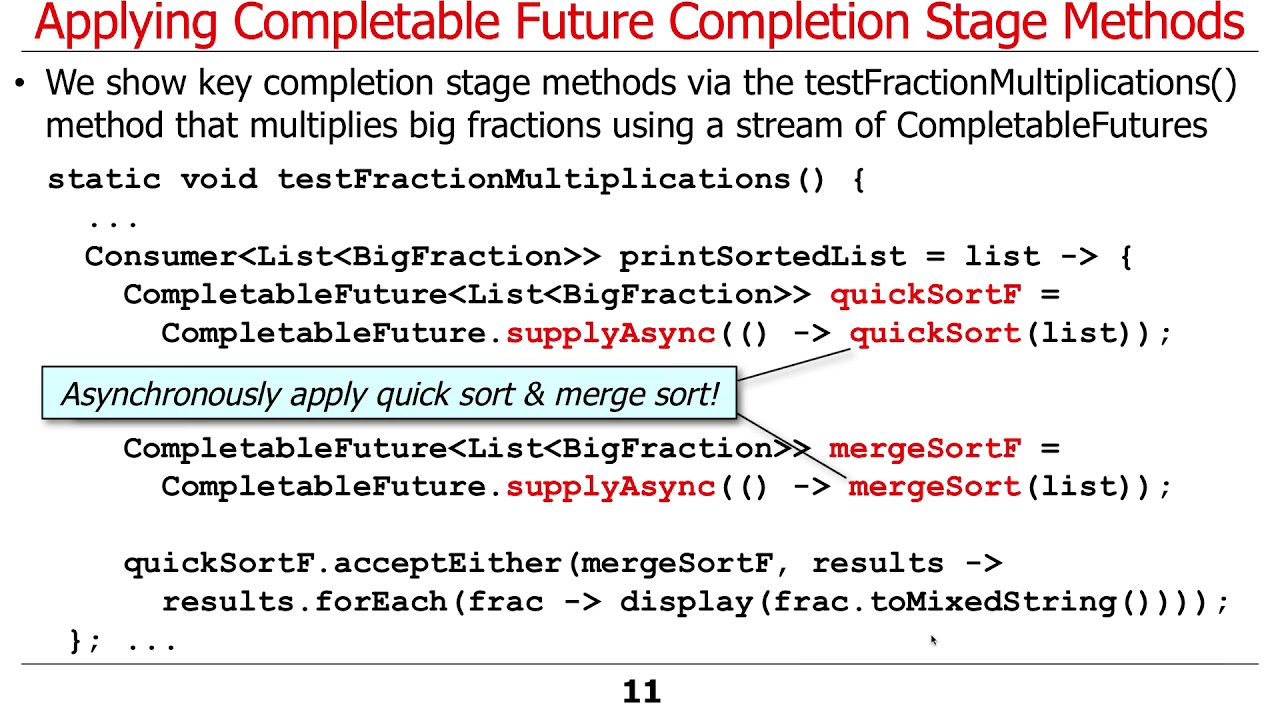
public static void main(String[] args) throws ExecutionException, InterruptedException {
ExecutorService executor = Executors.newSingleThreadExecutor();
// Create a CompletableFuture and submit a task to it
Future future = executor.submit(() -> {
// Simulate some time-consuming operation
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return 42;
});
// Use the get() method to wait for the result of the task
int result = future.get();
System.out.println("Result: " + result);
executor.shutdown();
}
}
In this example, we're creating a CompletableFuture and submitting a simple task (calculating 42) to it. The task is executed in a separate thread pool, which allows our main program to continue running while the task is being processed.
Once the task is complete, we can use the get()
method to retrieve its result. This method will block until the result is available, so this code won't finish until the task has completed.
Here's another example that uses callbacks:
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class CompletableFutureExample {
public static void main(String[] args) throws ExecutionException {
ExecutorService executor = Executors.newSingleThreadExecutor();
// Create a CompletableFuture and submit a task to it with callback
CompletableFuture future = CompletableFuture.runAsync(() -> {
// Simulate some time-consuming operation
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return 42;
}).thenApply(result -> {
System.out.println("Result: " + result);
return result;
});
int result = future.get();
System.out.println("Final result: " + result);
executor.shutdown();
}
}
In this example, we're using the thenApply()
method to add a callback to our CompletableFuture. This callback is executed once the task has completed, and it receives the result of the task as an argument.
We then use the get()
method to retrieve the final result.
These are just simple examples of what you can do with Java's CompletableFuture API. With this API, you can write more concise and readable code for handling asynchronous tasks in your Java applications!