Java inner class vs nested class vs static
Java inner class vs nested class vs static
I'll respond in English this time!
In Java, there are three types of classes: inner classes, nested classes, and static classes. While they share some similarities, each has its own unique characteristics.
Inner Classes
An inner class is a non-static nested class that is defined inside another class. It is essentially a member of the enclosing class, which means it has access to all the members (methods, fields, etc.) of the outer class. Inner classes are used to create objects that are closely related to the outer class.
Here's an example:
public class Outer {
private int x;
public Outer(int x) {
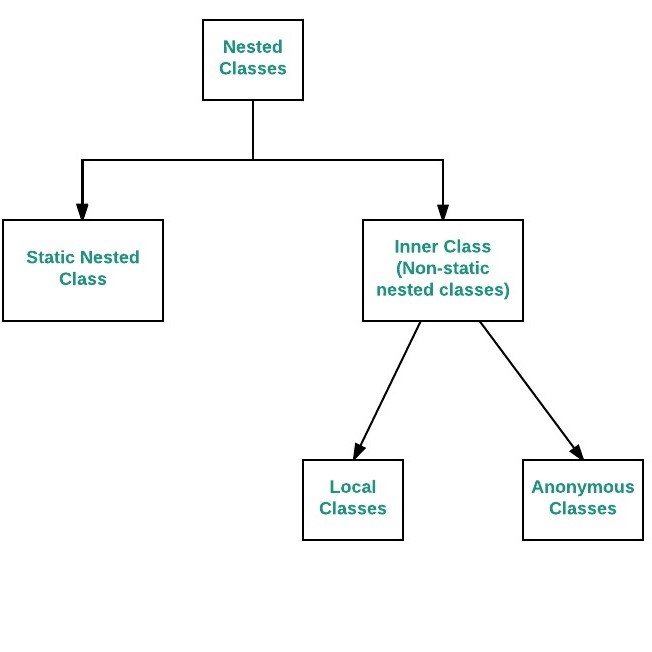
this.x = x;
}
public class Inner {
public void printX() {
System.out.println(Outer.this.x);
}
}
}
In this example, the Inner
class is an inner class of the Outer
class. The Inner
class has access to the x
field and can print its value using Outer.this.x
.
Nested Classes
A nested class is a static or non-static class that is defined inside another class. Unlike inner classes, nested classes do not have direct access to the members of the enclosing class.
Here's an example:
public class Outer {
public static class Nested {
public void printMessage() {
System.out.println("Hello from Nested!");
}
}
}
In this example, the Nested
class is a nested class of the Outer
class. It can be accessed using the fully qualified name Outer.Nested
.
Static Classes
A static class is a top-level class that is defined inside another class. Static classes are essentially outer classes with no direct access to the members of the enclosing class.
Here's an example:
public class Outer {
public static class StaticNested {
public void printMessage() {
System.out.println("Hello from Static Nested!");
}
}
}
In this example, the StaticNested
class is a static class that can be accessed using the fully qualified name Outer.StaticNested
.
Key differences
To summarize:
Inner classes are non-static and have direct access to the members of the enclosing class. Nested classes (static or non-static) do not have direct access to the members of the enclosing class, but can still be used as utility classes. Static classes are top-level classes with no direct access to the members of the enclosing class.In conclusion, Java's inner, nested, and static classes provide a range of options for creating reusable code. Each type has its own unique characteristics, and choosing the right one depends on the specific requirements of your project.
Java inner class vs nested class javatpoint
Here's a detailed explanation of the difference between a Java inner class and a nested class:
What is an Inner Class?
In Java, an inner class is a non-static nested class that has access to its enclosing class's members (methods, variables, etc.) without being qualified by an instance of the outer class. This means that an inner class can use the outer class's variables and methods directly.
Inner classes are defined inside the definition of another class, method, or constructor. They have access to the outer class's private members as well, which is not possible with a nested class. Inner classes are also known as "local classes" because they are defined locally within the scope of an outer class or block.
Example of an Inner Class:
public class OuterClass {
int x = 10;
public void display() {
System.out.println("Value of x: " + x);
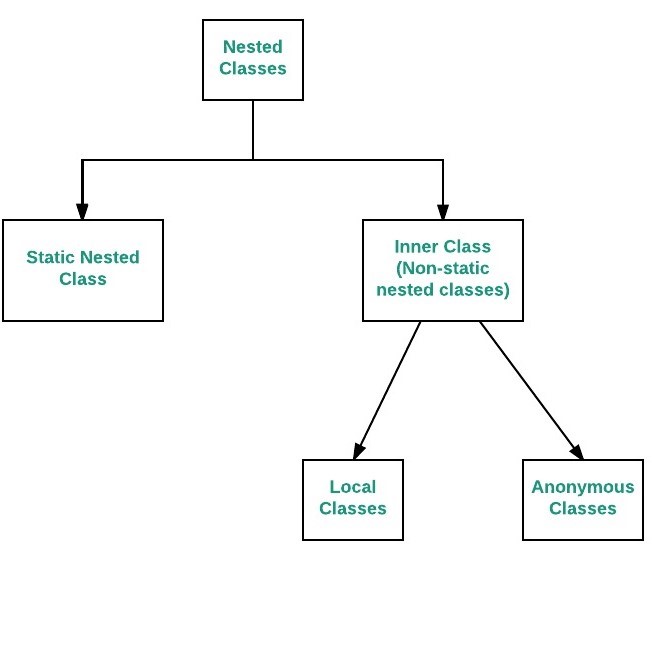
class InnerClass {
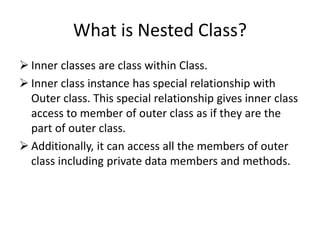
int y = 20;
public void print() {
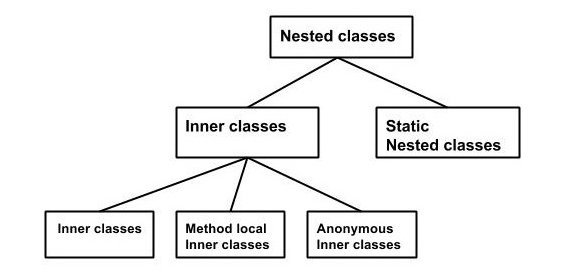
System.out.println("Value of x and y: " + x + ", " + y);
}
}
InnerClass inner = new InnerClass();
inner.print(); // Prints: Value of x: 10, 20
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
outer.display();
}
}
What is a Nested Class?
A nested class is a class that is defined inside another class or interface. Unlike an inner class, a nested class does not have direct access to its enclosing class's members without being qualified by an instance of the outer class.
Nested classes are also defined inside the definition of another class, method, or constructor. However, unlike inner classes, nested classes do not have automatic access to the outer class's private members.
Example of a Nested Class:
public class OuterClass {
int x = 10;
public void display() {
System.out.println("Value of x: " + x);
public static class NestedClass {
int y = 20;
public void print() {
System.out.println("Value of x and y: " + OuterClass.this.x + ", " + y);
}
}
NestedClass nested = new NestedClass();
nested.print(); // Prints: Value of x: 10, 20
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
outer.display();
}
}
In the above examples, the inner class has direct access to x
without qualifying it with an instance of OuterClass
, whereas the nested class does not have direct access and needs to qualify it with OuterClass.this
.
Key Differences:
Inner classes can access their enclosing class's private members directly, while nested classes cannot. Inner classes do not require qualification withthis
or the outer class name, whereas nested classes do.
In summary, inner classes are non-static nested classes that have direct access to their enclosing class's members without being qualified by an instance of the outer class. Nested classes are static classes defined inside another class or interface and cannot access the private members of the outer class directly.