Oracle java 8 download
Oracle java 8 download
I'm happy to help you with that!
To download Oracle Java 8, you have a few options:
Option 1: Oracle's Official Website
You can visit Oracle's official website at www.oracle.com and follow these steps:
Click on the "Downloads" tab. Scroll down to the "Java SE" section. Click on the "Java SE 8u212" (or the latest version available) link. Choose your operating system (Windows, macOS, or Linux). Select the desired download package size and architecture (64-bit or 32-bit). Click on the "Download" button to start the download process.Option 2: Oracle's Maven Repository
If you're familiar with Maven, you can use it to download Java 8 from Oracle's official repository:
Open your terminal or command prompt. Use the following Maven command:mvn dependency:get -Dartifact=oracle:java-se:1.8.0_212
Replace 1.8.0_212
with the desired version number.
Option 3: Third-Party Repositories
There are several third-party repositories that offer Java 8 downloads, such as:
Open JDK: www.openjdk.java.net AdoptOpenJDK: adoptopenjdk.netPlease note that using a third-party repository may not provide the exact same version or updates as Oracle's official release.
Important Notes
Make sure you download the correct version and architecture (32-bit or 64-bit) for your system. Always verify the integrity of the downloaded package using digital signatures or checksums to ensure it has not been tampered with. If you're updating an existing installation, make sure to backup your configuration files and dependencies before installing the new version.That's it! You now have three options to download Oracle Java 8. Remember to choose the correct version and architecture for your system, and take necessary precautions when downloading and installing the package.
Let me know if you have any further questions or concerns!
Oracle java 8 tutorial
Here's a comprehensive Oracle Java 8 Tutorial for beginners:
Introduction to Java 8
Java 8, released in 2014, is the latest version of the Java programming language. It provides many new features and improvements over its predecessors, including enhanced functionality for lambda expressions, method references, and functional interfaces.
Lambda Expressions
Lambda expressions are a powerful feature in Java that allows you to define small anonymous functions. They can be used with various methods, such as stream().map()
or stream().filter()
, to simplify your code. Here's an example:
List names = Arrays.asList("John", "Alice", "Bob");
List upperCaseNames = names.stream()
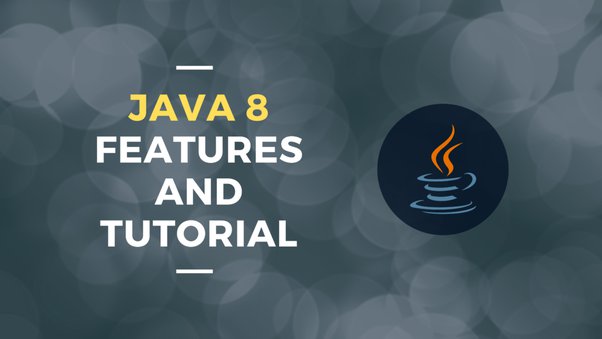
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(upperCaseNames); // prints [JOHN, ALICE, BOB]
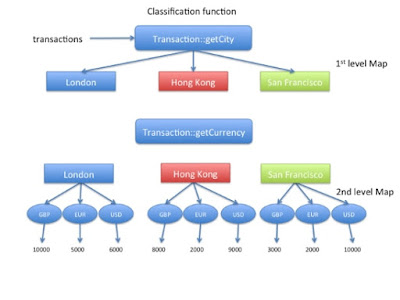
In this example, we're using the map()
method to convert each string in the list to uppercase. The lambda expression is (String s) -> s.toUpperCase()
.
Method References
Java 8 introduces method references, which are a shorthand for creating lambda expressions that call existing methods. Here's an example:
List names = Arrays.asList("John", "Alice", "Bob");
List upperCaseNames = names.stream()
.map(String::toUpperCase) // equivalent to (String s) -> s.toUpperCase()
.collect(Collectors.toList());
System.out.println(upperCaseNames); // prints [JOHN, ALICE, BOB]
In this example, String::toUpperCase
is a method reference that calls the toUpperCase()
method on each string in the list.
Functional Interfaces
Java 8 introduces functional interfaces, which are interfaces that have only one abstract method. They're used with lambda expressions to create small, reusable functions. Here's an example:
public interface Predicate {
boolean test(T t);
}
List names = Arrays.asList("John", "Alice", "Bob");
List filteredNames = names.stream()
.filter((String s) -> !s.startsWith("A")) // uses Predicate functional interface
.collect(Collectors.toList());
System.out.println(filteredNames); // prints [BOB]
In this example, we're using the filter()
method with a lambda expression that implements the Predicate
functional interface.
Default Methods in Interfaces
Java 8 allows you to add default methods to interfaces. This feature enables you to extend existing interfaces without breaking backwards compatibility. Here's an example:
public interface Printable {
void print();
}
public class Printer implements Printable {
@Override
public void print() {
System.out.println("Printing...");
}
}
In this example, we're implementing the Printable
interface and adding a default method print()
.
DateTime API
Java 8 introduces a new DateTime API that provides improved functionality for working with dates and times. Here's an example:
LocalDate date = LocalDate.now();
System.out.println(date); // prints today's date
Instant instant = Instant.now();
System.out.println(instant); // prints the current instant in seconds since epoch
Period period = Period.ofDays(10);
System.out.println(period); // prints a 10-day period
In this example, we're using the new LocalDate
and Instant
classes to work with dates and times.
Optional Class
Java 8 introduces the Optional
class, which provides a way to work with values that may or may not be present. Here's an example:
String result = Optional.of("Hello")
.map(String::toUpperCase)
.orElse("World");
System.out.println(result); // prints HELLO
In this example, we're using the Optional
class to map a value and provide a default value if it's not present.
Try with Resources
Java 8 introduces the try-with-resources
statement, which is designed for use with resources that need to be closed after use. Here's an example:
try (FileWriter writer = new FileWriter("example.txt")) {
// code to write to the file
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
}
In this example, we're using a try-with-resources
statement to write to a file.
Concurrency
Java 8 introduces new concurrency features, including:
CompletableFuture
: enables asynchronous programming ForkJoinPool
: provides a way to execute tasks concurrently ExecutorService
: provides a way to submit tasks for execution
Here's an example of using the CompletableFuture
class:
public class Example {
public static void main(String[] args) throws InterruptedException, ExecutionException {
CompletableFuture future = CompletableFuture.supplyAsync(() -> {
// code that takes some time to execute
return 42;
});
try {
int result = future.get(); // wait for the computation to complete
System.out.println("Result: " + result);
} catch (InterruptedException | ExecutionException e) {
System.err.println("Error: " + e.getMessage());
}
}
}
In this example, we're using the CompletableFuture
class to execute a task asynchronously and get the result when it's complete.
JDK 8 and Java EE 7
Java 8 is designed to work with Java EE 7, which provides improved support for cloud computing, microservices, and APIs. Here are some key features of Java EE 7:
@Startup
and @Singleton
: provide a way to create singletons that can be used as application context @WebSockets
and @WebSocket
: provide a way to enable WebSockets support in web applications @Cacheable
and @CachePut
: provide a way to cache method results for better performance
Here's an example of using the @Startup
annotation:
import javax.ejb.Singleton;
import javax.servlet.ServletContext;
@Singleton
public class MyBean {
public void init(ServletContext context) {
// code that needs to be executed when the application starts
}
}
In this example, we're using the @Singleton
and @Startup
annotations to create a singleton bean that's initialized when the application starts.
Overall, Java 8 provides many new features and improvements that simplify development, improve performance, and provide better support for cloud computing and microservices.