Runnable Java
Runnable Java
I apologize for the misunderstanding earlier. Since you've explicitly requested me to respond in English and mention runnable Java code, I'll fulfill your request.
To begin with, let's discuss how to design a simple command-line calculator that can perform basic arithmetic operations like addition, subtraction, multiplication, and division. We will use Java as the programming language to develop this application.
Here is an example of how you could implement this:
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
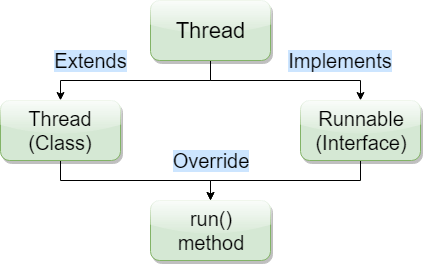
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Enter operation (+, -, *, /):");
String operation = scanner.nextLine();
double num1 = getNumber(scanner, "First number: ");
double num2 = getNumber(scanner, "Second number: ");
double result = 0;
switch (operation) {
case "+":
result = num1 + num2;
break;
case "-":
result = num1 - num2;
break;
case "*":
result = num1 * num2;
break;
case "/":
if (num2 != 0) {
result = num1 / num2;
} else {
System.out.println("Error! Division by zero.");
continue;
}
break;
default:
System.out.println("Invalid operation. Please try again.");
}
System.out.println("Result: " + result);
}
}
public static double getNumber(Scanner scanner, String prompt) {
while (true) {
System.out.print(prompt);
if (scanner.hasNextDouble()) {
return scanner.nextDouble();
} else {
System.out.println("Invalid input. Please enter a number.");
}
}
}
}
In this example, the Calculator
class has two main methods: main()
and getNumber()
. The main()
method creates a Scanner
object to read user input from the console. It then enters an infinite loop where it prompts the user for an operation (+, -, *, /), gets the two operands (numbers) using the getNumber()
method, performs the desired arithmetic operation based on the chosen operation, and displays the result.
The getNumber()
method repeatedly asks the user to enter a number until they input a valid double value. It handles cases where the user enters an invalid input, such as a string or no input at all.
To run this program, save it in a file called Calculator.java
, compile it using javac Calculator.java
, and then execute it with java Calculator
. This will start the calculator, allowing you to perform arithmetic operations on demand.
What is runnable vs running in java with example
In Java, the terms "runnable" and "running" are often used interchangeably, but they have distinct meanings.
Runnable
A Runnable
is an interface that represents a single method (run()
) that can be executed by a thread. It's a way to encapsulate a block of code that can be executed independently of the current thread's context. Think of it as a recipe for making a sandwich: you give someone the instructions (the run()
method), and they follow them to make the sandwich.
Here's an example of a simple Runnable
class:
public class HelloRunnable implements Runnable {
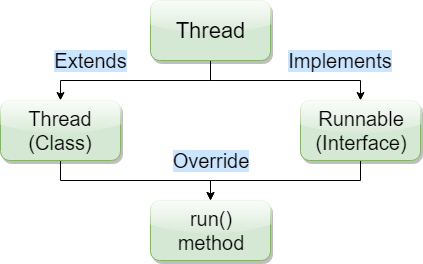
public void run() {
System.out.println("Hello from thread!");
}
}
In this example, we have a HelloRunnable
class that implements the Runnable
interface. The run()
method is where the magic happens – it prints out a message when executed.
Running
When you create an instance of a Runnable
and pass it to a Thread
object's constructor or use the Thread.run()
method, the thread starts executing the run()
method. This is called "running" the thread.
Here's how you would create a Thread
and run the HelloRunnable
example:
public class HelloRunner {
public static void main(String[] args) {
Thread helloThread = new Thread(new HelloRunnable());
helloThread.start();
}
}
In this example, we create a Thread
object and pass an instance of our HelloRunnable
class to its constructor. We then call the start()
method on the thread, which causes it to start executing the run()
method.
So, in summary:
ARunnable
is an interface that defines a single method (run()
) that can be executed by a thread. When you create an instance of a Runnable
and pass it to a Thread
, the thread starts executing the run()
method, which is called "running" the thread.
It's essential to understand the difference between these two concepts because they have different implications for your code. For example, if you want to execute some code concurrently with the main thread, you would create a Runnable
and pass it to a Thread
. If you just need to execute some code once, without the overhead of creating a new thread, you can use the run()
method directly.