Java static nested class
Java static nested class
I'd be happy to help you with your question about Java static nested classes!
A static nested class is a special type of inner class that can be instantiated without an instance of the outer class. This is achieved by declaring the nested class as static.
Here's an example:
public class Outer {
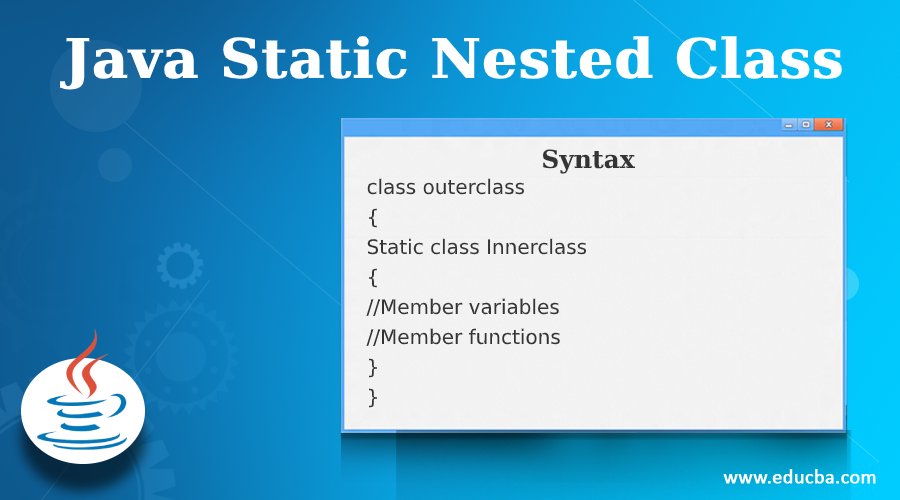
public static class Inner {
public void printHello() {
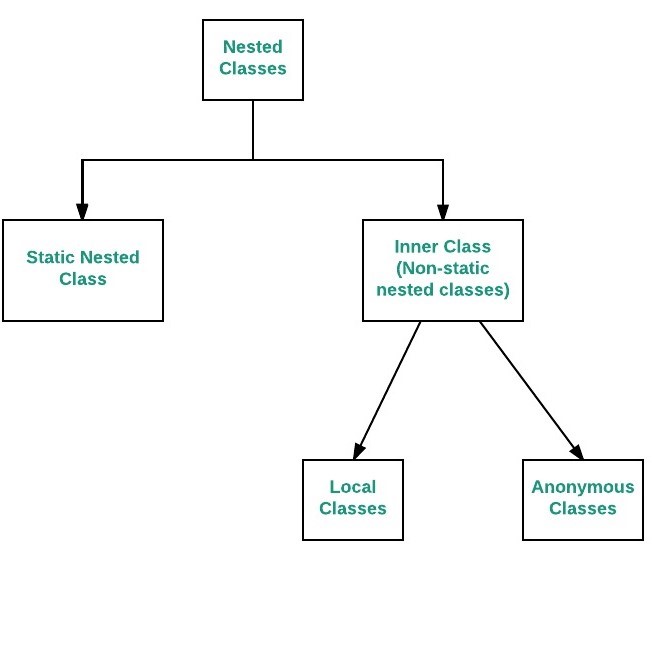
System.out.println("Hello from Inner class!");
}
}
public static void main(String[] args) {
Inner inner = new Inner(); // Can be instantiated without an outer instance
inner.printHello();
}
}
In this example, the Inner
class is a nested class of the Outer
class. The static
keyword on the Inner
class declaration means that it can be instantiated without creating an instance of the Outer
class.
This is in contrast to regular nested classes, which require an instance of the outer class to be created before they can be instantiated:
public class Outer {
public class Inner {
public void printHello() {
System.out.println("Hello from Inner class!");
}
}
public static void main(String[] args) {
// Cannot create an instance of Inner without an Outer instance
// Outer outer = new Outer();
// Outer.Inner inner = outer.new Inner();
// Instead, you would need to create an Outer instance first:
Outer outer = new Outer();
Outer.Inner inner = outer.new Inner();
// Now you can instantiate the Inner class
inner.printHello();
}
}
In this case, we cannot directly create an instance of the Inner
class without first creating an instance of the Outer
class. This is because regular nested classes are not static and require a reference to their containing class.
Static nested classes are useful when you want to encapsulate a utility or helper class that can be used independently of its outer class. They also provide a way to group related functionality together without having to create a separate package or namespace.
One key benefit of static nested classes is that they can access the outer class's members, including variables and methods, using the Outer.this
syntax:
public class Outer {
private int x = 10;
public static class Inner {
public void printX() {
System.out.println("x = " + Outer.this.x);
}
}
public static void main(String[] args) {
Inner inner = new Inner();
inner.printX(); // prints "x = 10"
}
}
In this example, the Inner
class can access the Outer
class's private variable x
using the Outer.this
syntax.
Overall, static nested classes provide a way to encapsulate and organize related functionality in Java, while also providing flexibility and access to members of its outer class.
What is the difference between static and non static in Java?
I'm happy to help!
In Java, the terms "static" and "non-static" (also known as "instance" or "dynamic") refer to the scope of a variable, method, or class.
Static
A static variable or method is bound to the class itself, rather than to an instance of the class. This means that there is only one copy of the variable or method in memory, and it is shared by all instances of the class.
Here are some key characteristics of static members:
Non-static (Instance)
A non-static variable or method is bound to an instance of the class, meaning each object created from the class has its own unique copy of the variable or method.
Here are some key characteristics of non-static members:
Unique per instance: Each instance of the class has its own separate copy of the variable or method. Instance required: You need to create an instance of the class to access a non-static member. Object-level access: Non-static members can be accessed using an instance of the class.To illustrate the difference, let's consider a simple example:
public class Dog {
static int numDogs; // shared among all instances
public Dog(String name) {
this.name = name;
numDogs++; // increments the shared counter
}
public void bark() {
System.out.println("Woof!");
}
}
In this example:
numDogs
is a static variable, so it's shared among all instances of the Dog
class. When you create multiple Dog
objects, they will all share the same value for numDogs
. The bark()
method is an instance method (non-static), so each Dog
object has its own separate copy of the method. When you call bark()
on different instances, it will behave independently.
In summary:
Static members are shared among all instances of a class and can be accessed without creating an instance. Non-static members are bound to individual instances of a class and require an instance to access them.I hope this helps clarify the difference between static and non-static in Java!