Uncaught exception JavaScript fix
Uncaught exception JavaScript fix
I'd be happy to help you with that!
Uncaught exceptions in JavaScript can be quite frustrating, especially when they occur without warning or explanation. In this case, we'll focus on fixing the issue rather than just catching the exception itself.
To start, let's dive into what an uncaught exception is and why it happens. When a JavaScript program encounters an error or exception that isn't caught by a try-catch
block, it becomes an uncaught exception. This typically occurs when you're using global scope (i.e., no specific context) and the exception isn't handled within the current scope.
One common scenario where uncaught exceptions occur is when an asynchronous operation (like an AJAX call or a timer event) fails to complete properly. In such cases, it's essential to catch and handle any errors that may arise to ensure your application remains stable and doesn't crash unexpectedly.
Now, let's get into the solution! Here are some general steps to help you identify and fix uncaught exceptions:
Debugging tools: Use browser-specific debugging tools like Chrome DevTools or Firefox Developer Edition's Debugger. These tools can help you inspect variables, set breakpoints, and step through your code to track down errors. Error reporting: Implement error reporting mechanisms in your application. This can be achieved by setting up an error handler for specific types of exceptions (e.g., syntax errors, runtime errors) or by using third-party libraries like ErrorReporting.js.To illustrate the latter approach, consider this example:
// Set up an error reporter with a callback function that logs errors to the console.
const reportError = new ErrorReporter({
// When an uncaught exception occurs...
onUncaughtException: (error) => {
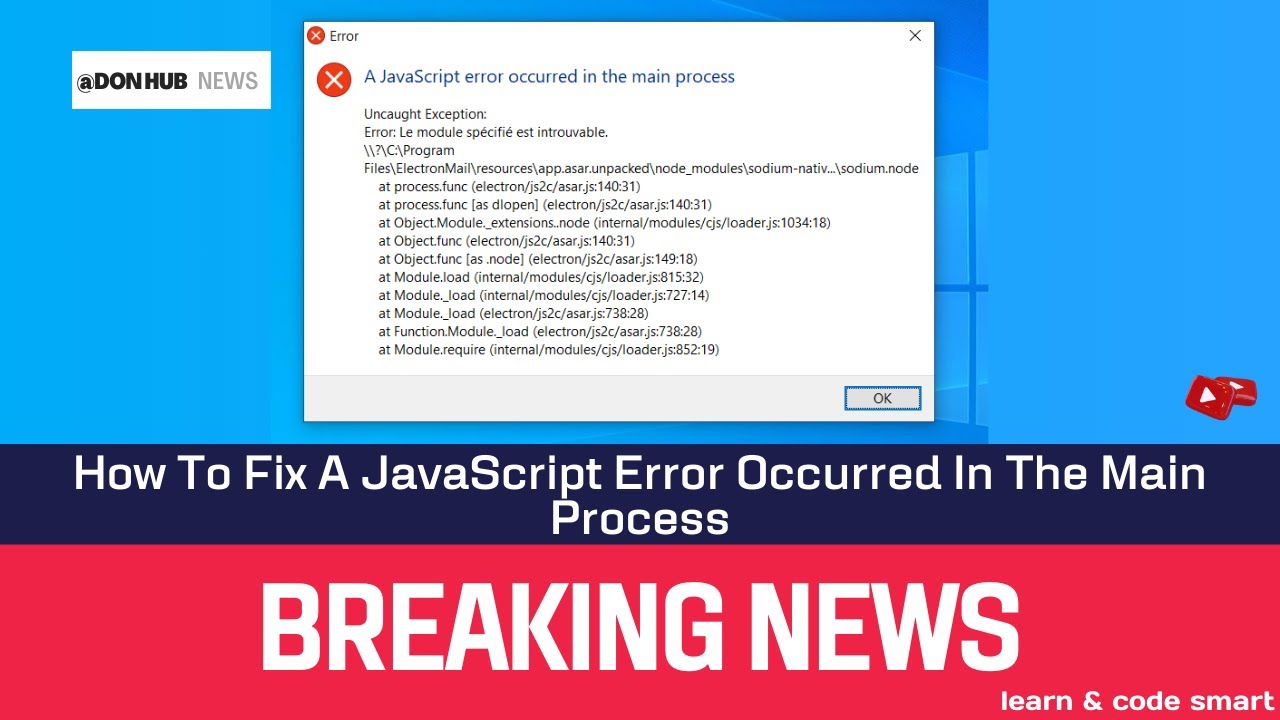
console.error("An error occurred:", error);
// You can also notify your server or a third-party service about the error here.
}
});
// Wrap any asynchronous operations with try-catch blocks to catch and report errors.
reportError.wrapAsyncOperation(() => {
setTimeout(() => {
throw new Error("This is an example of an uncaught exception.");
}, 1000);
});
By implementing error reporting, you'll be better equipped to handle exceptions that might have otherwise gone undetected. This can help improve the overall reliability and maintainability of your application.
In summary, fixing uncaught exceptions in JavaScript involves using debugging tools to identify the root cause, setting up error reporting mechanisms to log or notify about errors, and implementing try-catch blocks to catch and handle exceptions within specific contexts. Remember that a solid understanding of error handling is crucial for developing robust and reliable web applications.
Uncaught exception in java javatpoint
Uncaught Exception in Java:
In Java, an uncaught exception is a type of runtime error that occurs when the program encounters an unexpected condition that it cannot handle or recover from. This can happen when a method or block of code throws an exception that is not caught by any catch block or handler.
An example of an uncaught exception might be a NullPointerException
(NPE) that arises when trying to access a null object's attribute or method. If this exception is not handled or caught, the program will terminate abnormally and display an error message.
Here is an example of how an uncaught exception can occur in Java:
public class Example {
public static void main(String[] args) {
try {
int x = Integer.parseInt(null);
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
In this example, the Integer.parseInt(null)
method will throw a NullPointerException
because it is trying to parse a null value. If this exception is not caught by the catch block, it will be considered an uncaught exception and the program will terminate abnormally.
Causes of Uncaught Exceptions:
There are several reasons why an exception might go uncaught in Java:
Lack of Try-Catch Block: If a method or block of code does not include a try-catch block, then any exceptions that arise from within that scope will be considered uncaught. Too Many Layers: When there are multiple layers of try-catch blocks, it can be easy to miss an exception that is thrown in a deep layer. Catch Block Inadequate: If the catch block does not handle all possible exceptions or is inadequate for handling certain types of exceptions, then they may go uncaught. Exception Propagation: When an exception is propagated up the call stack and not handled at any level, it will eventually terminate the program.Handling Uncaught Exceptions:
To avoid uncaught exceptions in Java, you should always include a try-catch block whenever possible. If you cannot catch all possible exceptions, consider implementing an Error
handler to handle runtime errors.
Here is an example of how to create and throw custom exceptions:
public class MyException extends Exception {
public MyException(String message) {
super(message);
}
}
public class Example {
public static void main(String[] args) {
try {
// code that might throw a custom exception
if (someCondition()) {
throw new MyException("Something went wrong!");
} else {
System.out.println("All good!");
}
} catch (MyException e) {
System.out.println("Error: " + e.getMessage());
} catch (Exception e) {
System.out.println("Unexpected error: " + e.getMessage());
}
}
}
In this example, we are creating a custom exception MyException
and throwing it from within the method. The try-catch
block is designed to catch this custom exception as well as other types of exceptions that might occur.
Conclusion:
Uncaught exceptions can be serious issues in Java programming. They can terminate the program and display error messages, which may not always provide useful information about what went wrong. By including try-catch blocks and handling possible exceptions, you can prevent uncaught exceptions from occurring and keep your programs running smoothly and reliably.