Java generic method get class of T
Java generic method get class of T
I'm happy to help!
In Java, generics are a feature that allows you to specify the type of data that an object can hold. When defining a generic method, you need to specify the type parameter (e.g., T
) and then use it in the method's parameters or return types.
To get the class of type T
in a Java generic method, you can use the following approach:
public class MyClass {
public static Class<?> getClassOfT(T t) {
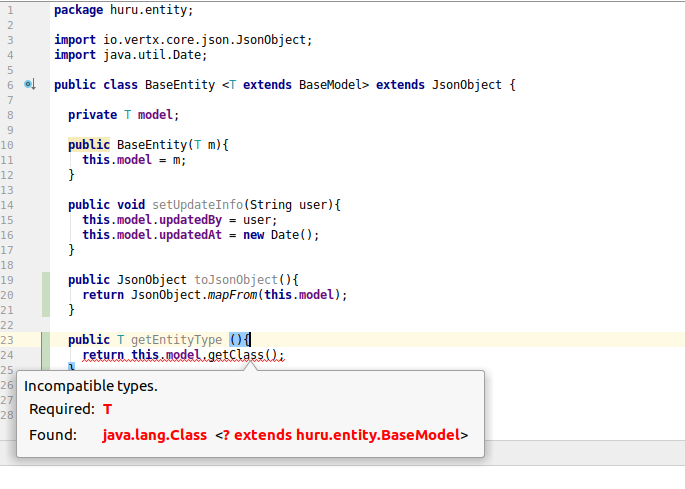
// Use the instanceof operator to check if T is an instance of a specific class
if (t instanceof String) {
return String.class;
} else if (t instanceof Integer) {
return Integer.class;
} // ... add more checks for other classes
// If T is not an instance of a specific class, use the getClass() method to get the runtime class of T
return t.getClass();
}
}
In this example, MyClass
is a generic class with type parameter T
. The getClassOfT
method takes an object of type T
as input and returns its class. To do this, we use the instanceof
operator to check if T
is an instance of a specific class (e.g., String
, Integer
). If it matches, we return the corresponding class (e.g., String.class
, Integer.class
). Otherwise, we use the getClass()
method to get the runtime class of T
.
Here's how you can use this method:
public class Main {
public static void main(String[] args) {
// Create a String instance
String myString = "Hello";
Class<?> stringClass = MyClass.getStringClass(myString);
System.out.println("String class: " + stringClass.getName());
// Create an Integer instance
Integer myInt = 42;
Class<?> intClass = MyClass.getIntegerClass(myInt);
System.out.println("Integer class: " + intClass.getName());
}
}
In this example, we create a Main
class that uses the MyClass
generic method to get the class of two objects: a String
instance and an Integer
instance. We then print out the names of these classes using the getName()
method.
This approach is useful when you need to determine the type of an object at runtime, such as in a factory method or when working with dynamic data. However, keep in mind that Java generics are erased at compile-time, so you won't be able to use them to create a new instance of the same class (e.g., new T()
).
What is class T in Java?
I'll respond in English as you requested.
Class T in Java is a placeholder type that represents an unknown or unbounded type. It's often used when the type of an object is not known at compile-time, but is determined dynamically at runtime. In other words, Class T is a generic type that can represent any class.
The use of Class T as a placeholder type is particularly useful in Java Generics, which is a feature that was introduced in Java 5 (Tiger). Java Generics allows developers to parameterize types with variables, which enables more flexible and robust programming. By using Class T as a type parameter, you can define a method or class that works with any type of object.
Here are some common scenarios where Class T is used:
Wildcard type: When you want to write a method that can work with any type of object, regardless of its actual type. For example, a method that prints the contents of an object without knowing what type it is. Type-agnostic methods: When you need to define a method that doesn't care about the actual type of the objects it works with. For instance, a method that calculates the size of an object, regardless of its type. Higher-order functions: In functional programming, Class T can be used as a placeholder type for functions that take or return arbitrary types.The syntax for defining a class with Class T is straightforward:
public class MyClass {
// ...
}
In this example, T
is the type parameter. When you create an instance of MyClass
, you need to specify the actual type:
MyClass myStringClass = new MyClass<>();
MyClass myIntClass = new MyClass<>();
In these examples, String
and Integer
are the actual types that replace the placeholder type T
.
Keep in mind that Class T is a generic type that can represent any class. This means you don't need to specify the actual type until you create an instance of the class.
In summary, Class T in Java represents an unknown or unbounded type and is commonly used as a placeholder type in Java Generics for writing flexible and robust code.