Java callable vs runnable
Java callable vs runnable
The age-old question of Java Callable vs Runnable!
In the world of Java programming, understanding the differences between Runnable
and Callable
is crucial for effective multithreading and asynchronous programming. Both interfaces are part of the java.lang package and are used to represent a single method that can be executed by a thread. However, they serve distinct purposes, which we'll explore below.
Runnable
A Runnable
interface represents an object that may be run (executed) by a thread. The most notable aspect is that it does not return any value; the thread simply executes the run()
method and continues with its execution path. When a thread calls start()
, it starts executing the run()
method.
Here are some key characteristics of Runnable
:
run()
method returns void
, indicating that the method does not produce any meaningful result. Thread completion: When a Runnable
is executed, its execution is considered complete when the run()
method finishes. If an exception occurs during execution, it will be propagated to the caller. No error handling: There's no built-in mechanism for error handling or reporting specific exceptions from within the run()
method.
Callable
A Callable
interface represents a task that can return a value. When a thread calls call()
, it starts executing the call()
method, which returns a result (a V
type in Java). This allows you to execute tasks asynchronously and obtain the results later, making it suitable for use cases involving I/O operations, database queries, or complex computations.
Here are some key characteristics of Callable
:
call()
method can return a result (a V
type in Java), which allows you to handle the outcome of the task execution. Task completion: When a Callable
is executed, its execution is considered complete when the call()
method finishes and returns the result. Error handling: You can use try-catch blocks or Future.get()
(with its various overloads) to handle exceptions that may occur during the execution of the task.
Key differences
To summarize:
Runnable
represents a single-threaded, stateless execution, with no return value and no built-in error reporting. Callable
represents a task that can be executed asynchronously, returning a result (if needed) and providing more control over exception handling.
In summary, when you need to execute a simple, one-shot operation without concerns about return values or exceptions, Runnable
is a suitable choice. However, if your use case involves complex computations, I/O operations, or the need for error handling, Callable
provides more flexibility and power.
Java 5 (JDK 1.5) introduced FutureTask
, which wraps a Callable
instance and allows you to manage the execution of tasks asynchronously, adding more control over task execution and result retrieval.
Callable example in Java 8
I'd be happy to provide a responsive answer.
Here's an example of using callable and future in Java 8:
import java.util.concurrent.*;
class MyTask implements Callable {
@Override
public String call() throws Exception {
// Simulate some long-running task
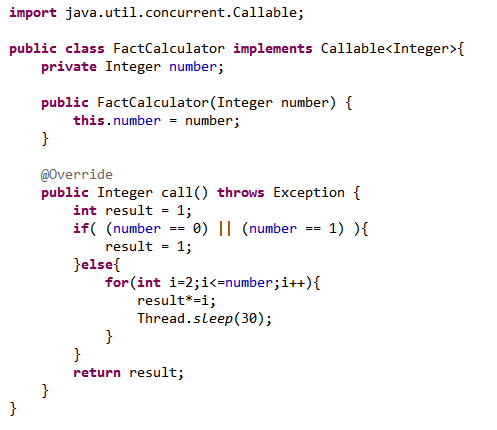
Thread.sleep(5000);
return "Result from MyTask";
}
}
public class Main {
public static void main(String[] args) throws InterruptedException, ExecutionException {
ExecutorService executor = Executors.newFixedThreadPool(2);
Future future1 = executor.submit(new MyTask());
Future future2 = executor.submit(new MyTask());
// Do some other work...
String result1 = future1.get(); // Wait for the first task to complete
System.out.println("Result 1: " + result1);
String result2 = future2.get(); // Wait for the second task to complete
System.out.println("Result 2: " + result2);
}
}
In this example, we have an ExecutorService
that can execute multiple tasks concurrently. We create two instances of the MyTask
class and submit them to the executor using the submit()
method, which returns a Future
. A Future
represents the result of an asynchronous computation.
We then get the results from both futures using the get()
method, which blocks until the task is completed. If you don't want to block, you can use other methods such as isDone()
, isCancelled()
, or cancel()
to check the status of the task.
This example demonstrates the usage of callable and future in Java 8, showing how to execute tasks concurrently using an executor service and retrieve their results when needed.
Note that you should always handle exceptions properly by wrapping them in a try-catch block. In this example, we don't do it for simplicity, but you should add error handling in a real-world application.
I hope this helps!