How do you call the main class in Java?
How do you call the main class in Java?
The main class in Java! That's a crucial concept to grasp when learning the world of programming with Java.
In Java, the main class is the starting point for any program that uses the Java Virtual Machine (JVM) to run. It's the entry point where your program begins execution. This special class contains the main
method, which is the first method called by the JVM when it starts running your program.
The main
method is a static method, meaning it can be called without creating an instance of the class. Its signature looks like this:
public static void main(String[] args) {
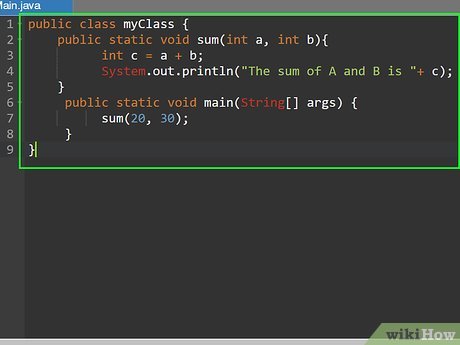
// Your program's entry point!
}
Now, let's talk about what makes a Java class "the main class." To be considered the main class, your Java file (usually with a .java
extension) must meet these conditions:
.java
extension). For example, if your file is named HelloWorld.java
, then your class must be declared as public class HelloWorld
. Public access modifier: Your main class must have a public access modifier to allow the JVM to find it. Main method: Your class must contain a main
method with the exact signature shown above (static, void returning, taking an array of Strings).
Here's an example of a simple Java program that demonstrates these conditions:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
When you run this program, the JVM will execute the main
method in the HelloWorld
class, and print "Hello, World!" to the console.
In summary, the main class in Java is a special class that serves as the starting point for your program. It must have a public access modifier, be named exactly like the filename (excluding the .java
extension), and contain a main
method with a specific signature.
Now, go forth and create some amazing Java programs!
How to call local class in Java?
To call a local class in Java, you can create an instance of the local class and then invoke its methods or access its fields. Here's a step-by-step guide:
What is a Local Class?
In Java, a local class is a non-public inner class that is defined inside another class, but not nested within any other class. It has access to all members (methods and variables) of the enclosing class.
Creating an Instance of a Local Class
To use a local class, you first need to create an instance of it. You can do this by using the new
keyword followed by the name of the local class:
MyEnclosingClass myObject = new MyEnclosingClass();
LocalClass myLocalInstance = myObject.new LocalClass();
In the above code, MyEnclosingClass
is the enclosing class and LocalClass
is the local class.
Accessing Methods and Fields of a Local Class
Once you have created an instance of the local class, you can access its methods and fields just like any other class. Here's how:
myLocalInstance.myMethod(); // calls the myMethod() method of the local class
String myField = myLocalInstance.myField; // accesses the value of the myField field
Advantages of Local Classes
There are several advantages to using local classes in Java. Here are a few:
Encapsulation: Local classes can encapsulate complex logic or data that is specific to the enclosing class, without exposing it to the outside world. Namespace: Local classes can use the same namespace as the enclosing class, making it easier to access members of the enclosing class. Scope: Local classes are scoped to the enclosing class, which means they are not visible outside that class.Example Code
Here's an example of using a local class in Java:
public class MyEnclosingClass {
private int myField;
public void myMethod() {
// ...
}
public class LocalClass {
public void doSomething() {
System.out.println("I'm doing something!");
}
}
}
public class Main {
public static void main(String[] args) {
MyEnclosingClass myObject = new MyEnclosingClass();
MyEnclosingClass.LocalClass myLocalInstance = myObject.new LocalClass();
myLocalInstance.doSomething(); // prints "I'm doing something!"
}
}
In this example, the MyEnclosingClass
has a local class called LocalClass
. The main()
method in the Main
class creates an instance of MyEnclosingClass
and uses it to create an instance of the LocalClass
. It then calls the doSomething()
method on that instance.