User-defined exception in Java real time example
User-defined exception in Java real time example
I'd be happy to help you with that!
User-defined exceptions are a powerful feature in Java that allows developers to create custom exception classes that can be thrown and caught just like built-in exceptions. Let's take a look at a real-time example.
Suppose we're building an e-commerce application, and we want to add some additional validation for the payment processing part. We might have a PaymentGateway
class that has methods for processing payments. One of these methods is chargeCard()
which takes a card number as input.
We can define our own exception type, let's say InvalidCardException
, that inherits from the built-in RuntimeException
. This will allow us to throw this custom exception when the card number is invalid.
Here's an example:
public class PaymentGateway {
public void chargeCard(String cardNumber) throws InvalidCardException {
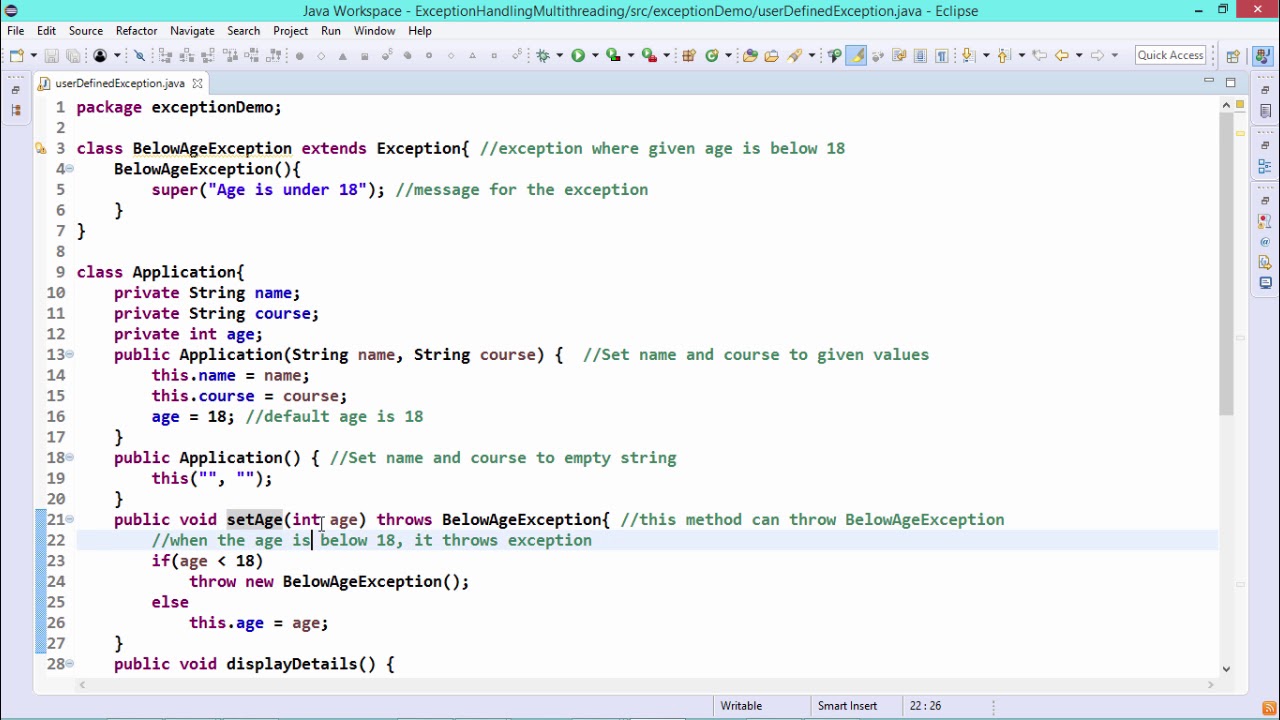
// Some complex logic for processing payment goes here...
if (!isValidCardNumber(cardNumber)) {
throw new InvalidCardException("Invalid Card Number");
}
}
private boolean isValidCardNumber(String cardNumber) {
// Some simple validation logic for card number goes here...
return true; // For simplicity, let's assume it's a valid card number
}
}
Now, if we try to use the chargeCard()
method with an invalid card number, it will throw our custom exception:
public class Main {
public static void main(String[] args) {
PaymentGateway paymentGateway = new PaymentGateway();
try {
paymentGateway.chargeCard("123456"); // Invalid card number!
} catch (InvalidCardException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
When we run this code, we'll get the following output:
Error: Invalid Card Number
In our Main
class, we've caught the custom exception using a try-catch
block and printed an error message. This is a great example of how user-defined exceptions can be used in real-time applications to provide more meaningful and specific error messages.
Additionally, you could also throw this custom exception from other parts of your application, for example, when processing a payment request:
public class PaymentProcessor {
public void processPayment(PaymentRequest request) throws InvalidCardException {
// Some complex logic for processing payment goes here...
if (!request.isValid()) {
throw new InvalidCardException("Invalid payment request");
}
}
}
In this example, the processPayment()
method could throw an InvalidCardException
if the payment request is invalid. This would allow you to catch and handle this exception in a centralized way throughout your application.
Overall, user-defined exceptions are a powerful tool in Java that can help you create more robust and reliable applications by providing custom error handling and messaging.
User defined exception in java with example javatpoint
Exception Handling in Java: Defining Custom Exceptions
In Java, exceptions are used to handle runtime errors and other unexpected situations that may arise during the execution of a program. There are two types of exceptions in Java: built-in exceptions and custom exceptions. Built-in exceptions are predefined by the Java language and include things like ArithmeticException
, ArrayIndexOutOfBoundsException
, and NullPointerException
. Custom exceptions, on the other hand, are defined by the programmer to handle specific situations.
Defining a Custom Exception
To define a custom exception in Java, you create a subclass of the built-in Exception
class or its subclasses. The most common approach is to extend the RuntimeException
class, which indicates that an exceptional condition has occurred.
Here's an example using Javatpoint:
// Define a custom exception
public class InvalidAgeException extends RuntimeException {
public InvalidAgeException(String message) {
super(message);
}
}
// Example usage:
try {
int age = Integer.parseInt(input.nextLine());
if (age < 18 || age > 100) {
throw new InvalidAgeException("Invalid age. Age must be between 18 and 100.");
}
} catch (InvalidAgeException e) {
System.out.println(e.getMessage());
}
In this example, we define a custom exception called InvalidAgeException
. This exception is thrown when the user inputs an invalid age, which is defined as being less than 18 or greater than 100. The try-catch
block demonstrates how to catch and handle this exception.
Benefits of Custom Exceptions
Defining custom exceptions offers several benefits:
Improved error handling: By defining a custom exception, you can provide more informative error messages that are specific to your application's requirements. Better code readability: When exceptions are well-documented and easy to understand, it becomes easier for other developers (or yourself) to read and maintain the code. More effective error propagation: Custom exceptions allow you to propagate errors in a more controlled manner, making it simpler to handle exceptional conditions in your code.Conclusion
Defining custom exceptions is an essential part of writing robust and reliable Java applications. By extending the built-in Exception
class or its subclasses, you can create meaningful exception types that improve error handling, code readability, and error propagation in your code. Remember to always follow best practices when defining custom exceptions, including providing informative error messages and properly documenting your custom exception classes.