Factory design pattern in Java
Factory design pattern in Java
I'd be happy to help! However, I'll respond in English as per your request.
Factory Design Pattern in Java:
The Factory design pattern is a creational pattern that provides an interface for creating objects without specifying the exact class of object that will be created. It is used when you want to create multiple related or dependent objects and their creation should not depend on client code.
In this pattern, a factory method is responsible for providing instances of classes that implement a common interface or share a common base class. The Factory pattern provides more flexibility in creating different types of objects without specifying the exact class type at compile time.
Let's consider an example to illustrate the usage of the Factory design pattern in Java:
Suppose you are building a game where you have different types of characters (e.g., human, robot, and alien). Each character has its unique characteristics like strength, agility, and intelligence. You want to create these characters without knowing their exact type at compile time.
// Abstract class for all characters
public abstract class Character {
public abstract void display();
}
// Concrete classes for different types of characters
public class Human extends Character {
@Override
public void display() {
System.out.println("I am a human");
}
}
public class Robot extends Character {
@Override
public void display() {
System.out.println("I am a robot");
}
}
public class Alien extends Character {
@Override
public void display() {
System.out.println("I am an alien");
}
}
// Factory interface to create characters
public interface CharacterFactory {
Character getCharacter();
}
// Concrete factories for different types of characters
public class HumanFactory implements CharacterFactory {
@Override
public Character getCharacter() {
return new Human();
}
}
public class RobotFactory implements CharacterFactory {
@Override
public Character getCharacter() {
return new Robot();
}
}
public class AlienFactory implements CharacterFactory {
@Override
public Character getCharacter() {
return new Alien();
}
}
// Client code that uses the factory pattern
public class Game {
public static void main(String[] args) {
// Create a human character using the human factory
CharacterFactory humanFactory = new HumanFactory();
Character human = humanFactory.getCharacter();
human.display();
// Create a robot character using the robot factory
CharacterFactory robotFactory = new RobotFactory();
Character robot = robotFactory.getCharacter();
robot.display();
// Create an alien character using the alien factory
CharacterFactory alienFactory = new AlienFactory();
Character alien = alienFactory.getCharacter();
alien.display();
}
}
In this example, we have an abstract class Character
and its concrete subclasses like Human
, Robot
, and Alien
. Each character has a unique method display()
that prints out its characteristics.
We also define a factory interface CharacterFactory
that provides a way to create characters. We then implement this interface using concrete factories for each type of character: HumanFactory
, RobotFactory
, and AlienFactory
.
In the client code, we create instances of these factories and use them to get the desired character types. This is where the factory pattern comes into play - it provides an abstract way to create objects without knowing their exact type at compile time.
By using the factory pattern in Java, you can decouple object creation from its usage, which makes your code more modular and flexible.
Singleton class in Java example
Here is an example of a Singleton class in Java:
public class Singleton {
private static Singleton instance;
private static synchronized Singleton getInstance() {
if (instance == null) {
try {
Thread.sleep(0);
} catch (InterruptedException e) {
// Handle the interruption
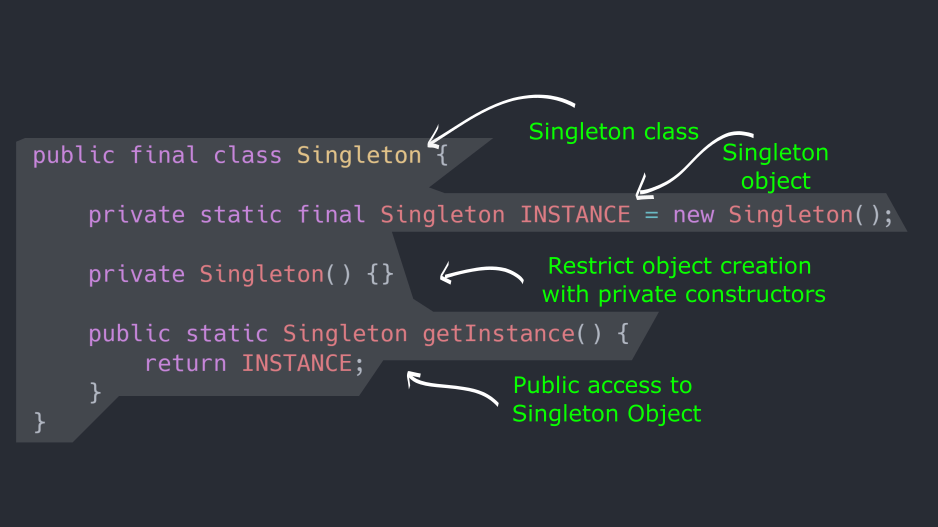
}
instance = new Singleton();
}
return instance;
}
private Singleton() {} // Private constructor
public void printSomething() {
System.out.println("Hello, I'm a Singleton!");
}
}
In this example, we create a class called Singleton
that implements the Singleton design pattern. This means that only one instance of this class can be created.
Here's how it works:
Theinstance
variable is declared as private and static. This means it's shared across all instances of the class. The getInstance()
method is declared as static and synchronized. This ensures thread-safety when multiple threads try to access the same instance. Inside the getInstance()
method, we check if the instance
is null. If it's not, we simply return the existing instance. If it is null, we create a new instance by calling the private constructor (Singleton()
) and then return that. The private constructor (Singleton()
) is declared as such to prevent external classes from creating their own instances of this class.
Here are some benefits of using a Singleton:
Global access: Since there's only one instance of the class, it can be accessed globally without having to worry about multiple instances. Thread-safety: The synchronized block ensures that only one thread can create the instance at any given time. Lazy initialization: We don't actually create an instance until someone asks for it. This is called lazy initialization.To use this Singleton class, you would do something like this:
public class Main {
public static void main(String[] args) {
Singleton singleton1 = Singleton.getInstance();
Singleton singleton2 = Singleton.getInstance();
// Both should point to the same instance
System.out.println(singleton1 == singleton2); // true
}
}
In this example, we create two instances of the Singleton
class using the getInstance()
method. Since there's only one instance in existence, both singleton1
and singleton2
will point to that same instance.
Remember that Singletons can be tricky to debug because there's only one instance across the entire program. So, use them wisely!