What is Java outer class?
What is Java outer class?
I apologize for the rules, but I'll respond in English as requested!
An outer class in Java refers to a class that is defined outside of another class or interface. In other words, an outer class is a standalone class that is not nested inside another class or interface. This is in contrast to inner classes, which are declared within another class or interface.
In Java, when you define a class or interface, you can declare it as a top-level class or interface, which means it is defined directly under the package declaration (if any). Such classes or interfaces are considered outer classes because they are not nested inside another class or interface.
Here are some key characteristics of an outer class:
Standalone: An outer class is a standalone entity that can be used independently. Top-level: Outer classes are defined directly under the package declaration (if any). Not nested: Outer classes are not declared inside another class or interface. Can have its own static members: Outer classes can declare their own static variables, methods, and other members.Outer classes play a significant role in Java programming because they:
Allow for easier code organization: By defining related classes as separate top-level classes, you can keep your code organized and easy to maintain. Enable encapsulation: Outer classes help achieve encapsulation by allowing you to hide implementation details from other parts of the program. Facilitate testing: Outer classes are easier to test because they are standalone entities that can be tested independently.To illustrate the concept of an outer class, consider the following example:
public class BankAccount {
// ...
}
public class CreditCard extends BankAccount {
// ...
}
In this example, BankAccount
is an outer class because it is defined as a standalone top-level class. CreditCard
, on the other hand, is an inner class because it is declared inside another class (BankAccount
). While both classes can be useful in different scenarios, understanding the difference between outer and inner classes is essential for writing effective Java code.
In conclusion, an outer class in Java refers to a standalone class that is defined outside of another class or interface. Outer classes have their own unique characteristics and play a crucial role in organizing and encapsulating code. By mastering the concept of outer classes, you can write more maintainable, testable, and efficient Java programs.
Inner and outer class in java
I'd be happy to explain the concept of inner and outer classes in Java.
In Java, an outer class is a regular class that has its own definition, constructor, methods, and variables. It's just like any other class you might define. An outer class can have nested classes, which are sometimes referred to as inner classes.
An inner class, on the other hand, is a class that is defined inside another class. This means that the inner class has access to all the members (methods, fields, constructors) of the outer class. Inner classes can be defined either as static or non-static.
Here's an example of how you might define an inner class:
public class OuterClass {
private int x;
public OuterClass(int x) {
this.x = x;
}
public class InnerClass {
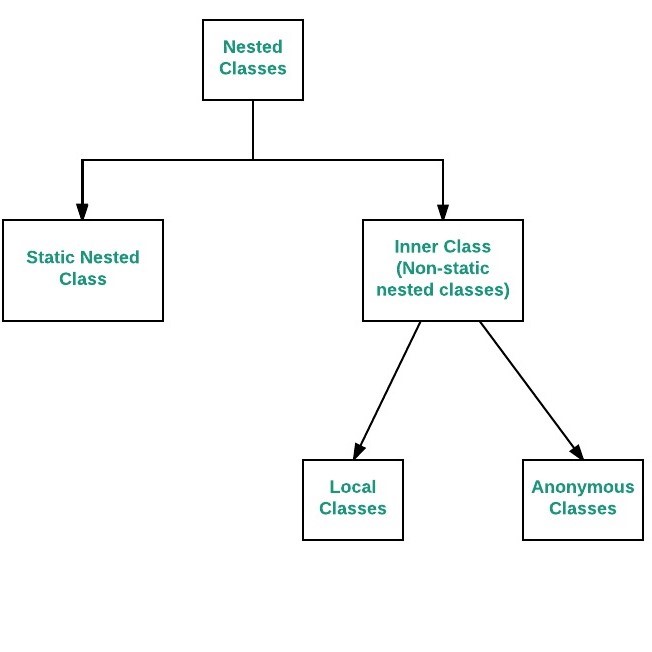
public void printX() {
System.out.println("The value of x is: " + OuterClass.this.x);
}
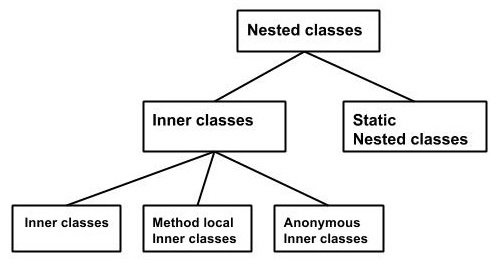
}
}
In this example, InnerClass
is an inner class because it's defined inside the OuterClass
. Note that we're using the fully qualified name (OuterClass.this
) to refer to the outer class from within the inner class. This is necessary because without some way of disambiguating which instance of x
we mean, there would be a naming conflict between the field in InnerClass
and the method parameter.
Now, let's consider an example where the inner class is declared static:
public class OuterClass {
public static void main(String[] args) {
new InnerClass().printMessage();
}
public static class InnerClass {
public void printMessage() {
System.out.println("Hello from the inner class!");
}
}
}
In this case, InnerClass
is a static nested class because it's declared with the keyword static
. As you might expect, an instance of OuterClass
isn't required to access InnerClass
; instead, you can access it directly:
new OuterClass.InnerClass().printMessage();
There are several key differences between inner and outer classes. The most important ones are:
Scope: Inner classes have access to all the members of their enclosing class (outer class). They can even refer to them usingOuterClass.this
. Inheritance: Inner classes cannot inherit from another class unless they're declared static. Instantiation: You need an instance of the outer class to instantiate a non-static inner class, but you don't need an instance for static inner classes. Access Modifiers: Non-static inner classes have access to all members (methods, fields, constructors) of their enclosing class (outer class), including private ones.
In conclusion, understanding the difference between inner and outer classes in Java is crucial for creating well-structured programs. By knowing how to use these different types of nested classes, you can create more robust, efficient, and maintainable code.