How to pass proxy in Java?
How to pass proxy in Java?
I'd be happy to help you with that!
In Java, passing a proxy is achieved through the use of interfaces and classes. A proxy is essentially an intermediary object that handles requests on behalf of another object, known as the target object.
Here's a step-by-step guide on how to pass a proxy in Java:
Step 1: Create an interface or abstract class
Define an interface or abstract class that represents the methods you want your target object to expose. For example:
public interface MyInterface {
void doSomething();
}
Step 2: Implement the interface or create a concrete subclass
Create a concrete class that implements the interface or extends the abstract class. This will be your target object.
For example:
public class MyClass implements MyInterface {
public void doSomething() {
System.out.println("Doing something...");
}
}
Step 3: Create a proxy class
Create another class that implements the same interface as the target object (MyInterface in this case). This will be your proxy object.
For example:
public class MyProxy implements MyInterface {
private MyClass target; // reference to the target object
public MyProxy(MyClass target) {
this.target = target;
}
@Override
public void doSomething() {
System.out.println("Proxy: Handling request...");
target.doSomething(); // delegate the call to the target object
}
}
Step 4: Use the proxy
Now, you can use your proxy instead of the target object. For example:
public class Main {
public static void main(String[] args) {
MyClass target = new MyClass();
MyProxy proxy = new MyProxy(target);
// Use the proxy
proxy.doSomething(); // Output: Proxy: Handling request... Doing something...
}
}
In this example, when you call doSomething()
on the proxy object (proxy
), it will handle the request by printing a message and then delegate the actual implementation to the target object (target
). This is the essence of proxying in Java.
By using proxies, you can decouple clients from the details of how they interact with targets. You can also use proxies for caching, logging, exception handling, or other purposes.
How to pass JVM arguments in Java code?
Passing JVM arguments in Java code is a straightforward process. There are several ways to achieve this:
1. Using the main
method
In your main
method, you can access the command-line arguments using the String[] args
parameter. This method works well for small-scale applications or when you want to pass specific parameters.
Here's an example:
public class Main {
public static void main(String[] args) {
// Accessing the command-line arguments
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
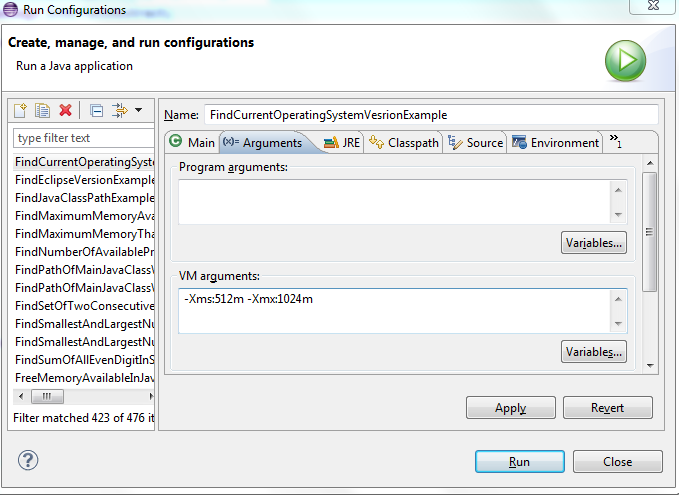
}
}
}
2. Using system properties
Java provides a way to access system properties using the System.getProperty()
method. This approach is useful when you want to pass configuration settings or environment variables.
Here's an example:
public class Main {
public static void main(String[] args) {
String propertyValue = System.getProperty("my.property");
// Use the property value as needed
}
}
3. Using a properties file
You can store JVM arguments in a properties file (e.g., config.properties
) and load them using the Properties
class.
Here's an example:
public class Main {
public static void main(String[] args) {
Properties props = new Properties();
try {
props.load(new FileInputStream("config.properties"));
String propertyValue = props.getProperty("my.property");
// Use the property value as needed
} catch (Exception e) {
e.printStackTrace();
}
}
}
4. Using an external configuration file
You can store JVM arguments in an external configuration file (e.g., a JSON or XML file) and load them using a library like Jackson or XStream.
Here's an example with Jackson:
public class Main {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
try {
JsonNode config = mapper.readTree(new File("config.json"));
String propertyValue = config.get("my.property").asText();
// Use the property value as needed
} catch (Exception e) {
e.printStackTrace();
}
}
}
When running your Java program, you can pass JVM arguments using the command line. For example:
java -Dmy.property=value Main
In this example, my.property
is a system property set to the value "value"
. You can access this property in your code using System.getProperty("my.property")
.
Remember to always follow best practices for storing sensitive information and configuration settings.