How do you invoke a method in Java?
How do you invoke a method in Java?
I'll respond in English as per your request.
Invoking a method in Java is the process of calling a specific method on an object, which executes the code defined within that method. This is one of the fundamental concepts in Java programming.
Here's how you can invoke a method in Java:
Method Invocation Syntax
The basic syntax to invoke a method in Java is:
objectName.methodName(parameters);
Let's break it down:
objectName
is the name of the object on which you want to call the method. This can be an instance of a class or a static reference to a class. methodName
is the name of the method you want to invoke. parameters
(optional) are values that need to be passed to the method as input.
Example
Suppose we have a simple Java class called Calculator
with a method called add()
:
public class Calculator {
public int add(int x, int y) {
return x + y;
}
}
To invoke this method on an instance of the Calculator
class, you would do something like this:
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
System.out.println("Result: " + result); // prints "5"
In this example:
We create an objectcalculator
of type Calculator
. We call the add()
method on calculator
, passing two integer parameters (2 and 3) as input. The add()
method executes, performing the addition operation and returning the result (which is 5 in this case). We print the result to the console using System.out.println()
.
Static Method Invocation
When a method is declared static, you don't need an instance of the class to invoke it. You can directly call the method on the class itself:
Calculator calculator = Calculator.add(2, 3);
System.out.println("Result: " + result); // prints "5"
In this case:
We don't create an objectcalculator
. We directly call the add()
method on the Calculator
class. The add()
method executes and returns the result (which is 5 in this case).
Method Overloading
Java allows you to overload methods, meaning multiple methods with the same name but different parameter lists. When you invoke a method with parameters that match one of the overloaded methods, Java will automatically call the correct method:
public class Calculator {
public int add(int x) {
return x;
}
public int add(int x, int y) {
return x + y;
}
}
In this case:
If you callcalculator.add(2)
(with a single integer parameter), Java will execute the first add()
method and return 2. If you call calculator.add(2, 3)
(with two integer parameters), Java will execute the second add()
method and return 5.
Method Chaining
Java allows you to chain multiple method calls together using dot notation:
Calculator calculator = new Calculator();
int result = calculator.add(2).multiply(4);
System.out.println("Result: " + result); // prints "8"
In this case:
We create an objectcalculator
of type Calculator
. We call the add()
method on calculator
, passing a single integer parameter (2) as input. The add()
method returns an object with a multiply()
method, which we then call to multiply the result by 4. We print the final result to the console using System.out.println()
.
These are the basics of invoking methods in Java. I hope this explanation helps you understand how to invoke methods effectively!
How to invoke a method in java reflection example
Java Reflection is a powerful tool that allows you to inspect and manipulate the structure of your program at runtime. One of the most common uses of Java Reflection is invoking methods on objects.
Here's an example of how to do this:
import java.lang.reflect.Method;
public class Main {
public static void main(String[] args) throws Exception {
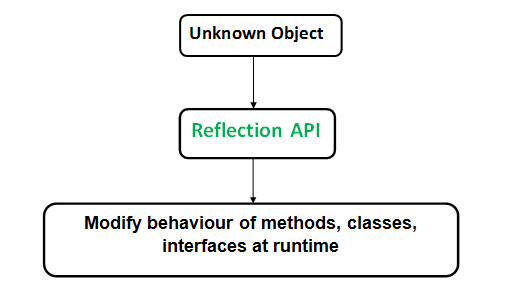
// Create an instance of a class
Class<?> clazz = Class.forName("YourClass");
Object obj = clazz.newInstance();
// Get the method you want to invoke
Method method = clazz.getMethod("yourMethod", new Class[]{String.class});
String param = "Hello";
// Invoke the method
method.invoke(obj, param);
// Print out the result
System.out.println(method.getReturnType() == Void.TYPE ? "No return value" : method.invoke(obj, param));
}
}
In this example, Class.forName("YourClass")
is used to get an instance of a class. Then Method.getMethod("yourMethod", new Class[]{String.class})
is used to find the method you want to invoke. The first argument "yourMethod" should be replaced with the name of your method and the second argument new Class[]{String.class}
specifies that this method takes one String
parameter.
The method.invoke(obj, param)
line then invokes this method on the object. Note that this will throw a InvocationTargetException
if the method throws an exception, or IllegalAccessException
if you don't have permission to access this method (for example, if it's private).
Finally, System.out.println(method.getReturnType() == Void.TYPE ? "No return value" : method.invoke(obj, param))
prints out the result of the invocation. If the method returns void (i.e., its return type is Void
), it simply prints "No return value". Otherwise, it calls the method again to get the return value and prints that.
Here's a full example:
import java.lang.reflect.Method;
public class ReflectionExample {
public void sayHello(String name) {
System.out.println("Hello, " + name + "!");
}
public static void main(String[] args) throws Exception {
// Create an instance of the class
Class<?> clazz = Class.forName("ReflectionExample");
Object obj = clazz.newInstance();
// Get the method you want to invoke
Method method = clazz.getMethod("sayHello", new Class[]{String.class});
// Invoke the method
method.invoke(obj, "John");
}
}
In this example, sayHello(String)
is a method that prints out a greeting message. The main method uses Java Reflection to create an instance of ReflectionExample
, get the sayHello
method, and then invoke it with the name "John". This will print out "Hello, John!".