Java get class of generic type
Java get class of generic type
I'd be happy to help with that!
In Java, generics are a way to achieve polymorphism and improve code reusability by allowing you to define classes and methods that can work with different data types.
When working with generics in Java, it is often necessary to get the actual class of a generic type. This is useful when you want to perform operations on an object that depends on its specific class rather than its interface or superclass.
Here's an example of how you can do this:
class Animal { }
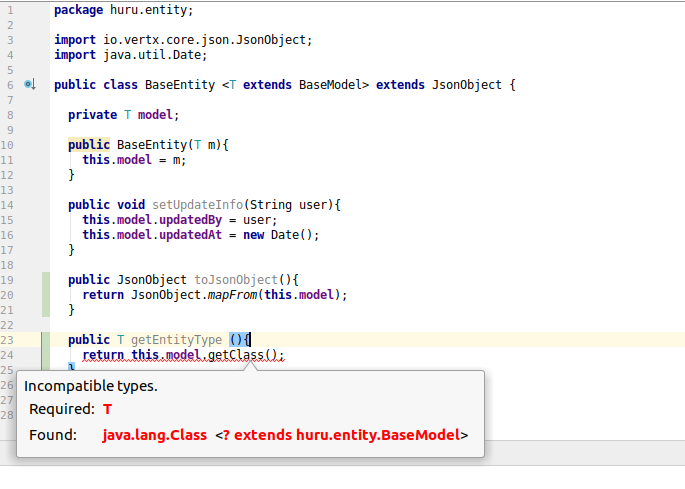
class Dog extends Animal { }
class Cat extends Animal { }
public class GenericClass {
public T getActualType() {
return (T) this.getClass().getGenericSuperclass();
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
Class clazz = ((GenericClass) new GenericClass()).getActualType();
System.out.println(clazz.getSimpleName()); // Output: "Dog"
animal = new Cat();
clazz = ((GenericClass<?>) new GenericClass()).getActualType();
System.out.println(clazz.getSimpleName()); // Output: "Cat"
}
}
In this example, GenericClass
is a generic class that takes a type parameter T
. The getActualType
method returns the actual type of T
.
To get the actual type of T
, we use the getClass()
method to get the current class, and then call getGenericSuperclass()
on it. This gives us the superclass of our generic class, which is the actual type of T
.
In the Main
class, we create instances of Dog
and Cat
, and pass them to GenericClass
. We then use the getActualType
method to get the actual class of each type.
As you can see, this approach allows us to get the actual class of a generic type at runtime. This is useful in situations where you need to perform operations that depend on the specific class of an object rather than its interface or superclass.
I hope this helps! Let me know if you have any questions.
Does Java have templates like C++?
I'd be happy to help!
Java does not have exact templates as C++. However, Java has a feature called Generics (introduced in Java 5) that provides some template-like functionality.
Generics allow you to define a class or method that can work with different data types without explicitly casting objects. This is achieved by using type parameters, which represent the types of the objects that are passed as arguments to methods or used as field types within classes.
Here's an example:
class Box {
private T t;
public void set(T t) {
this.t = t;
}
public T get() {
return t;
}
}
In this example, the Box
class is a generic class that can hold objects of any type T
. The type parameter T
represents the type of the object being stored in the box.
You can create instances of the Box
class and specify the type you want to use:
Box intBox = new Box<>();
intBox.set(42);
Integer value = intBox.get();
Box strBox = new Box<>();
strBox.set("Hello");
String message = strBox.get();
While this is not exactly like C++ templates, Generics in Java do provide a way to write type-safe code that can work with different types without the need for explicit casting.
In addition, Java also has an annotation called @FunctionalInterface
(introduced in Java 8) that allows you to define functional interfaces (like java.lang.Runnable
) and use them as templates. For example:
@FunctionalInterface
interface MyFunction {
void apply(T t);
}
public class Main {
public static void main(String[] args) {
MyFunction intFunction = i -> System.out.println(i * 2);
intFunction.apply(5);
MyFunction strFunction = s -> System.out.println(s.toUpperCase());
strFunction.apply("hello");
}
}
This example shows how you can define a functional interface MyFunction
that takes an argument of type T
, and then use it as a template to create functions that work with different types.
In summary, while Java does not have exact templates like C++, Generics and annotations provide ways to write type-safe code and use templates-like functionality in Java.